Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial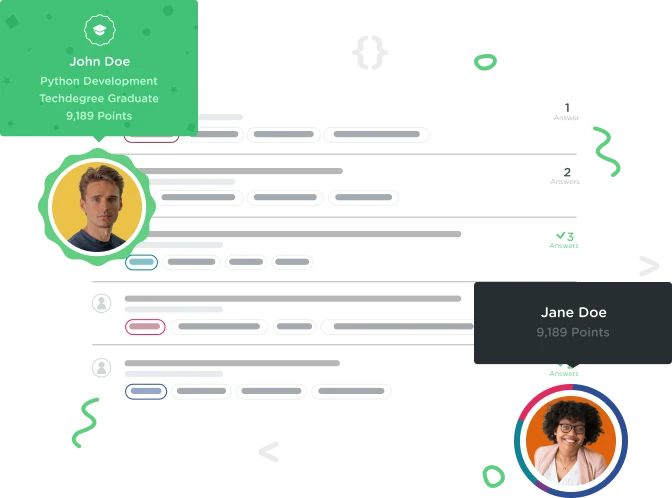
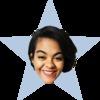
emeliataveras
Courses Plus Student 4,701 PointsCan you help me find out why there is an "undefined" showing up at the end of my program?
let qandA = [
[ `What is my name?`, `EMELY` ],
[ `Where do I live?`, `NJ` ],
[ `How old am I?`, `24` ],
[ `What is my ethnicity?`, `HISPANIC` ]
]
let answerRight = [ ];
let answerWrong = [ ];
function print(message) {
document.write(message);
}
function results() {
let html = `You got ${answerRight.length} question(s) right.<br><br>`;
html += `<strong>You got these questions correct:</strong>`;
html += `<ol><li>${answerRight.join('</li><li>')}</li>`;
html += `</ol>`;
if ( answerWrong.length > 0 ) {
html += `<strong>You got these questions wrong:</strong>`;
html += `<ol><li>${answerWrong.join('</li><li>')}</li>`;
html += `</ol>`;
print(html);
} else {
print(html);
}
}
// -------- Begin program --------------------------->
for ( let i = 0; i < qandA.length; i += 1 ) {
let answer = prompt(qandA[i][0]);
if ( answer.toUpperCase() === qandA[i][1] ) {
answerRight.push(qandA[i][0])
} else {
answerWrong.push(qandA[i][0])
}
}
print(results());
3 Answers
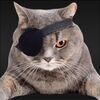
Nicole Antonino
12,834 PointsFunctions always have to return a value. In this case, your results() function isnt returning anything, you're trying to call print() on a value that doesn't exist yet because you haven't defined what the function should spit out. So your print function has nothing to actually print. The answer someone gave above works, but writing it with a return statement is better practice:
function results() {
let html = `You got ${answerRight.length} question(s) right.<br><br>`;
html += `<strong>You got these questions correct:</strong>`;
html += `<ol><li>${answerRight.join("</li><li>")}</li>`;
html += `</ol>`;
if (answerWrong.length > 0) {
html += `<strong>You got these questions wrong:</strong>`;
html += `<ol><li>${answerWrong.join("</li><li>")}</li>`;
html += `</ol>`;
return html;
} else {
return html;
}
}

Blake Larson
13,014 PointsThey explained it better below. Basically the return is used in print and results is calling print. document.write return undefined.
let qandA = [
[`What is my name?`, `EMELY`],
[`Where do I live?`, `NJ`],
[`How old am I?`, `24`],
[`What is my ethnicity?`, `HISPANIC`],
];
let answerRight = [];
let answerWrong = [];
function results() {
let html = `You got ${answerRight.length} question(s) right.<br><br>`;
html += `<strong>You got these questions correct:</strong>`;
html += `<ol><li>${answerRight.join("</li><li>")}</li>`;
html += `</ol>`;
if (answerWrong.length > 0) {
html += `<strong>You got these questions wrong:</strong>`;
html += `<ol><li>${answerWrong.join("</li><li>")}</li>`;
html += `</ol>`;
document.write(html);
} else {
document.write(html);
}
}
// -------- Begin program --------------------------->
for (let i = 0; i < qandA.length; i += 1) {
let answer = prompt(qandA[i][0]);
if (answer.toUpperCase() === qandA[i][1]) {
answerRight.push(qandA[i][0]);
} else {
answerWrong.push(qandA[i][0]);
}
}
results();

Zimri Leijen
11,835 Pointscorrect

Zimri Leijen
11,835 PointsBecause any function that doesn't explicitly return something will return undefined.
You are calling the function print
on results()
, but results doesn't return anything, so you are essentially calling print()
on undefined.
Maybe you intended to simply run results, in which case you can simply call result()
without wrapping it in print()
emeliataveras
Courses Plus Student 4,701 Pointsemeliataveras
Courses Plus Student 4,701 PointsAwesome, thank you so much! JS is so literal compared to CSS I keep forgetting small things like this that matter so much!