Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial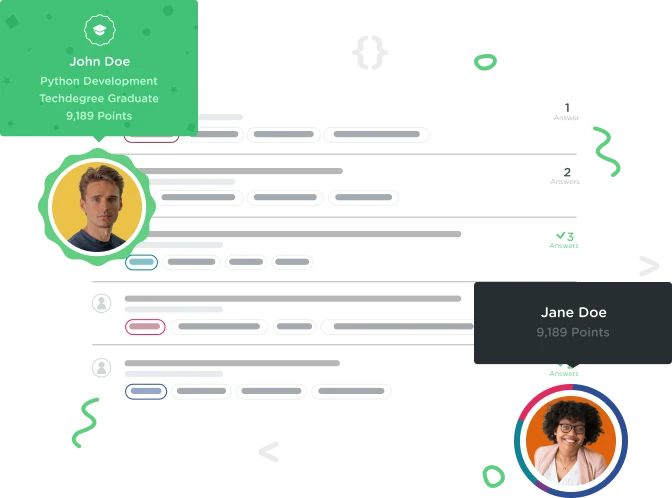
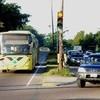
Ryan Croak
4,884 PointsCan you help me I don't understand what to do here I watched the video and it didn't help me
java Objects
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
}
3 Answers
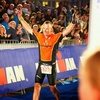
Steve Hunter
57,712 PointsHi Ryan,
The first task requires you to write a public
method that returns a boolean
which is called isBatteryEmpty
. It takes no parameters, so leave the parentheses empty but be sure to include them.
Inside the method, compare barCount
to zero using ==
and return
the result of that comparison.
Let me know how you get on.
Steve.
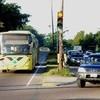
Ryan Croak
4,884 PointsOk, thank you guys for the help i'll see if I can figure it out.
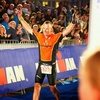
Steve Hunter
57,712 PointsLet me know how you get on.
Steve.

Karel Schwab
12,955 PointsHey Ryan. This is one example of how you can solve it:
Firstly, you would create a public method that returns a boolean and is named isBatteryEmpty: public boolean isBatteryEmpty() {};
Then you would create a boolean variable inside the created method and initialize it to false: boolean isEmpty = false;
Use an if statement to determine if the barCount is equal to 0: if(barCount == 0){isEmpty = true;}
Lastly, return the variable you created in the method: return isEmpty;
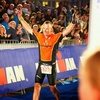
Steve Hunter
57,712 PointsOr you could just return barCount == 0;
- that expression evaluates to a true
or false
outcome, so there's no need to use an if
conditional. Just return the result of the comparison.
If you find yourself writing code such as this (and this is just an example for illustrative purposes):
public boolean methodName(){
if(binaryCondition){
return true;
}else{
return false;
}
}
you can just use:
public boolean methodName(){
return binaryCondition;
}
as long as binaryCondition
can only be true
or false
, such as a comparison to a number. In this example, the variable barCount
is either equal to zero or it isn't; there's no edge case so its equality-comparison to zero can only evaluate to true
or false
, negating the need for an if
conditional.
Steve.