Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial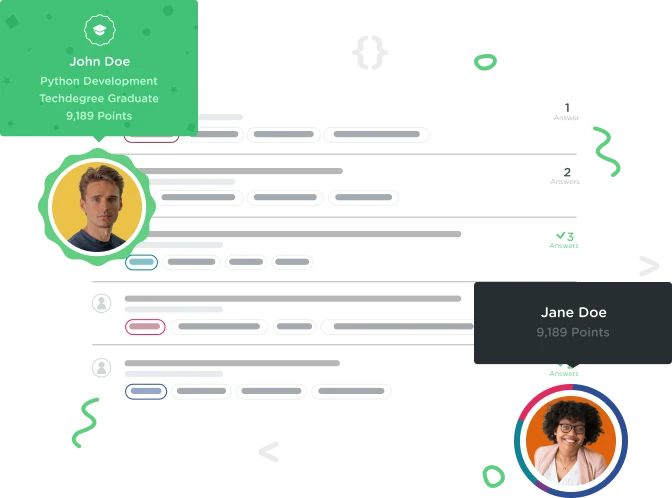
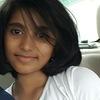
Anusha Singh
Courses Plus Student 22,106 PointsCan you help me out with this?
Add more input validation to your program by printing “You must enter a positive number.” if the user enters a negative number. I can't figure out that how can I add more input validation as I have already written a try and catch statement. PLEASE HELP! Thanks, in advance.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
try {
Console.Write("Enter the number of times to print \"Yay!\": ");
string printYay = Console.ReadLine();
int PrintYay = int.Parse(printYay);
int count = 0;
while (count != PrintYay) {
Console.WriteLine("Yay!");
count ++;
if (count == PrintYay) {
break;
}
}
} catch (FormatException) {
Console.WriteLine("You must enter a whole number.");
}
}
}
}
3 Answers
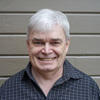
Dale Severude
Full Stack JavaScript Techdegree Graduate 71,350 PointsThis is asking for additional validation within your Try Catch loop. Like Steven said, you will need to add an additional if
statement to test for this. I think it is just a matter of visualizing what the question is asking for.
if (PrintYay <= 0)
{
Console.WriteLine("You must enter a positive number.");
}
else
{
//While loop for printing out Yah!
}
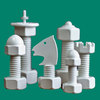
Steven Parker
231,269 PointsThis validation will be a bit easier to add than the previous one. After the input is converted to a number in "PrintYay", just test it (with an "if" statement) to see if the value is less than zero, and issue the error message if so instead of running the loop.
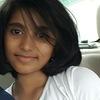
Anusha Singh
Courses Plus Student 22,106 PointsHow do you think I can write that down in code?
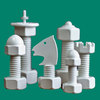
Steven Parker
231,269 PointsDale's example illustrates my suggestion except where I said "to see if the value is less than zero" (the challenge specifically asks you to test for a negative number):
if (PrintYay < 0) // <-- note "<" instead of "<="
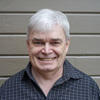
Dale Severude
Full Stack JavaScript Techdegree Graduate 71,350 PointsWith an error message of "You must enter a positive number."
I made the assumption that zero needs to be tested also.
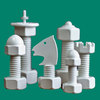
Steven Parker
231,269 PointsEasy mistake. But for best results always follow the instructions.