Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial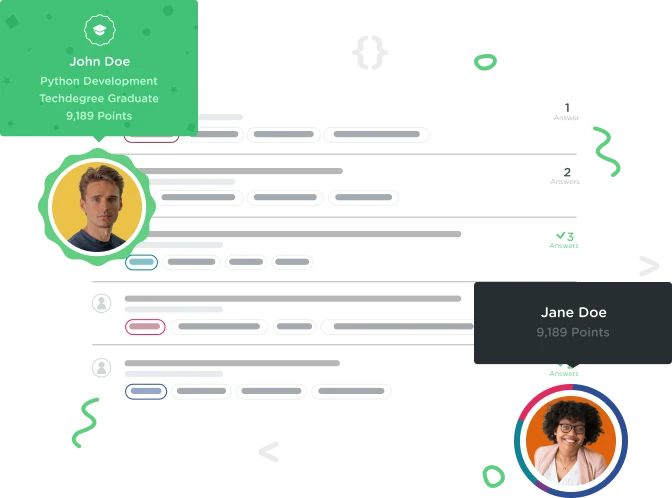

Andrei Oprescu
9,547 PointsCan you help me with this java question? I am struggling.
I am currently doing the 'Java objects' course and I have been given a quiz. The objective is this:
Create a new method named drive that accepts no arguments. It should call the newer drive method passing in a 1 for the default.
Currently my code looks like this:
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive(int laps) {
lapsDriven += laps;
barCount -= laps;
}
}
Thank you!
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive(int laps) {
lapsDriven += laps;
barCount -= laps;
}
}
2 Answers
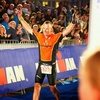
Steve Hunter
57,712 PointsHi again,
Let's take the drive
method:
public void drive(int laps){
// do stuff
}
The laps
integer is the parameter. Many people, including me, will use the two words interchangeably but they aren't quite the same thing. When the method is written, that's a parameter, as above. When you use the method:
drive(32);
then the 32 is the argument. But they relate to the same thing.
So, when I said, call the existing drive method and pass in 1 for its parameter., I should have said argument as this is the terms used when the method is being called.
Either way, they both refer to the things being sent into the method to do something with.
Does that help?
Steve.
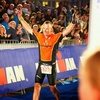
Steve Hunter
57,712 PointsHi there,
You have an existing drive
method that takes a parameter of the number of laps to drive. We want to create a new method, also called drive
that doesn't take any parameters but which uses the existing drive
method to just drive one lap.
So, make a public method called drive
. It returns nothing and takes no parameters. Inside it, call the existing drive
method and pass in 1
for its parameter.
Make sense?
Steve.

Andrei Oprescu
9,547 PointsI understand your response, but I am still a bit uninformed of what parameters and arguments mean. could you explain to me what they mean?
Andrei Oprescu
9,547 PointsAndrei Oprescu
9,547 PointsHi!
Thank you very much for your response. It was very helpful.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem!