Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial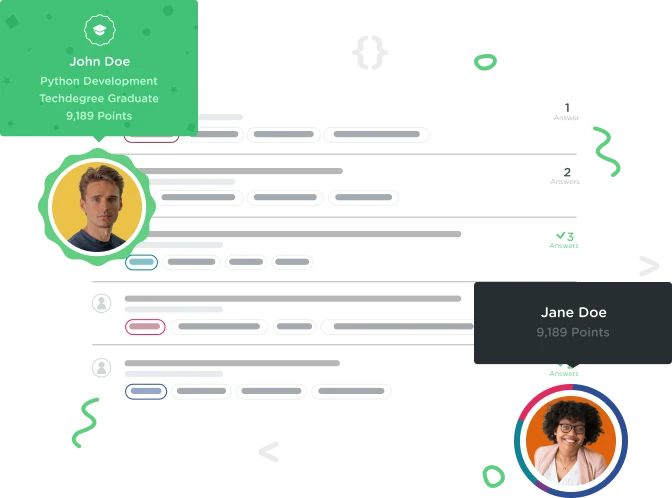
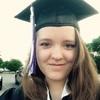
Chandelor Simon
2,242 PointsCan you make this game better?
I've written some code for a number guessing game - everyone is welcome to use it!
For my first solo project I'm feeling pretty proud! Still, I know there's a lot of ways it could be improved (maybe some things could be condensed, some things could be changed to show it's aware the number is > or <, etc.), and I'm not versed enough to know how; so my fellow tree-housers: who wants to take a crack at it?
name = input("Hello - what's your name? ")
initial_response = input("Nice to meet you {}; would you like to play a game? ".format(name))
if initial_response == "yes":
print("Great, let's get started!")
else:
print("C'mon - think about saying, 'yes'! Practice is always good!")
try_again = input("So - {}.... \nWould you like to play a game? ".format(name))
if try_again == "yes":
print("Awesome - thanks for reconsidering, {}; let's get started!!".format(name), "\nSo {}, I'm thinking of a number between 1-100...".format(name))
else:
print("I'll go ahead - see if you can keep up!!! \nI'm thinking of a number between 1-100...")
first_answer = input("Can you guess what number it is? ")
first_answer = int(first_answer)
correct_answer = first_answer == 82
if correct_answer:
print("Way to go! That's the number I was thinking of!!!")
else:
print("Good guess but not quite! The number I'm thinking of is bigger than 50.")
second_answer = input("Guess (another) number bigger than 50: ")
second_answer = int(second_answer)
correct_answer = second_answer == 82
if correct_answer:
print("Way to go! That's exactly right!!!!")
else:
print("Oooh! Another good guess {}, but not quite there!".format(name), "The number I'm thinking consists of 2 digits...\nWhen you add them together, it makes 10...\nIt's bigger than 73...")
third_answer = input("What is the number? ")
third_answer = int(third_answer)
correct_answer = third_answer == 82
if correct_answer:
print("You got it this time! Good game {}!!!".format(name))
else:
print("Almost there! Remember: this number has 2 digits that add to 10...\nIt's bigger than 73... and I'll give you another hint!\nIt's not 91...")
fourth_answer = input("You got it now? What's the number? ")
fourth_answer = int(fourth_answer)
correct_answer = fourth_answer == 82
if correct_answer:
print("YES! You got it! Nice game :)")
else:
print("Nope - it was 82! Better luck next time {}!".format(name))
2 Answers

Samuel Savaria
4,809 PointsWhen are asking them if they want to play a game, you should change the initial_response variable to all lower cases (initial_response.lower()) and check if "yes" is contained inside that string ("yes" in initial_response.lower()). Right now, your code only accepts "yes" as a valid answer. With this change, your code will ignore upper cases and punctuation. That way, the user can type "Yes" or "yes." or "YES!!!" and the code will accept it.
As you said, you could use the >, <, >=, <= operators to tell the user if their guess is too high or too low. But you could also ignore invalid guesses. For example, if the user enters 150, you can tell them their answer is not valid and ask them to try again.
Your correct_answer is useless. If you put boolean operators in the argument, the if statement will use the result of that operation. In other words, you should do your comparison directly into the if statement instead of creating a variable and storing the result.
Instead of repeating your code over and over again, you could use a for loop. You haven't seen those yet, but every time the program reaches the end of the block, a variable is incremented (increased by 1). The loop ends when the variable has reached a pre-determined value. For examples, if you assigned this value to be 4 and put your code for guessing in the loop, after 4 tries the loop would end and the user would lose.
You haven't seen that yet, but you could use a random number generator (RNG) instead of using the same number every time. A simple way of doing that would be referring to a method like time.time() and taking the modulo of that (time.time() % 100). That value would be considered random since it is nearly impossible to predict.
Note: x % 100 will give you a value between 0 and 99. You should use (x % 100) + 1 to adjust the range.
Note2: You also need to include the time module using "import time"
Finally, and most importantly, you should make your code more readable. Separate the different sections of your code with spaces and add comments to tell the reader what your are doing or what your logic is. To insert a comment in Python, you simply need to type "#" at the beginning of a line and the whole line will become a comment. Comments are highlighted in green and are ignored by the program.
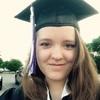
Chandelor Simon
2,242 PointsThanks Samuel - here's what I've done so far (I tried to do snippets of the code for different areas but that didn't work out, so please excuse):
I changed it to recognize any form of 'yes' in the initial response by changing it to: initial_response.lower()
I took out the 'correct_answer' bits and changed it to be reflected in the if statements: if first_answer == 82:
I added in <,>, and accounted for invalid numbers: (if first_answer < 82:) & (else: print("\nThat's not a valid guess; the number I'm thinking of is between 1-100\nTry again!"))
I added in comments and spaces to make it legible: # Prompts user to suggest another number.
I would have added in a loop and used a RNG, but I don't quite know how to do those things yet, so for the sake of making it work in a way I understood I left it out (I'll update it later as I become more aware), however I'm running into a new "problem".
I wanted to be able to end the game early if the user decided they didn't want to play or if they guessed correctly early on without running the rest of the program.
Suppose the user said they didn't want to play, I came up with this: print("\nOkay - maybe next time! You can always type this to play the game:\n") (# this was a new line) exit("python numbers2.py")
Now, technically it worked, but I have a feeling I'm cheating a bit - especially considering the word 'exit' is not the color I thought it would be. I thought I might be able to fix this by writing (after print("\nOkay - maybe next time!"): if "yes" or "maybe" in try_again.lower(): print("\nSo {}, I'm thinking of a number between 1-100...".format(name)
I thought perhaps if I specified, a "yes" or "maybe" response would give reason to print the next bit, but that didn't work; the, "...thinking of a number..." bit came up anyway, so I added in the (exit("python numbers2.py") with a little string before to explain why that was coming up (but passing it off as a way to play the game again)). Is this the correct way to do that? Any thoughts or guidance would be lovely.
I'll attach the whole game so far in another comment so this post isn't a mile long (no loops and all - it's getting long haha) for clarification and so the updates can be seen.
If anyone wants to play around with it or show me some things please do!
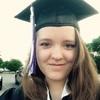
Chandelor Simon
2,242 PointsOkay, here it is so far:
name = input("Hello - what's your name? ")
# Asks if user would like to play and checks to see if 'yes' is in their response.
initial_response = input("\nNice to meet you {}; would you like to play a game? ".format(name))
"yes" in initial_response.lower()
# If 'yes' is in the response: the game starts; if 'yes' is not in response, user is prompted to
#say 'yes'
if "yes" in initial_response.lower():
print("\nGreat, let's get started!\n\nI'm thinking of a number between 1 and 100...")
else:
print("\nC'mon - think about saying 'yes'! Games are fun!")
try_again = input("\nSo - {}.... \nWould you like to play a game? ".format(name))
"yes" in try_again.lower()
# Different responses given depending on user input; if the user says 'yes' or 'maybe' the game #begins; if not: the game is discontinued.
if "yes" in try_again.lower():
print("\nAwesome - thanks for reconsidering, {}; let's get started!!".format(name)),
elif "maybe" in try_again.lower():
print("\nIt's not hard - I promise!")
else:
print("\nOkay - maybe next time! You can always type this to play the game:\n")
exit("python numbers2.py")
# If the user chooses to play, they are asked to guess a number between 1-100
if "yes" or "maybe" in try_again.lower():
print("\nSo {}, I'm thinking of a number between 1-100...".format(name))
first_answer = input("Can you guess what number it is? ")
# Number changed from string to integer.
first_answer = int(first_answer)
# Determines if the number picked by user is 82, higher, or lower; if incorrect - gives hint.
if first_answer == 82:
print("\nWay to go! That's the number I was thinking of!!!\n\nIf you'd like to play again you can type in:")
exit("python numbers2.py")
elif first_answer > 82:
print("\nGood guess - but not quite! The number I'm thinking of is less than that.")
elif first_answer < 82:
print("\nGood guess - but not quite! The number I'm thinking of is higher than that.")
else:
print("\nThat's not a valid guess; the number I'm thinking of is between 1-100\nTry again!")
# Prompts user to suggest another number
second_answer = input("Guess another number: ")
# Number changed from string to integer.
second_answer = int(second_answer)
# Determines if the number picked by user is 82; if incorrect - gives hint.
if second_answer == 82:
print("Way to go! That's exactly right!!!!\n\nIf you'd like to play again you can type in:")
exit("python numbers2.py")
else:
print("\nOooh! Another good guess {}, but not the number I'm thinking of!".format(name), "\n\nThe number I'm thinking of is a 2 digit number...\nWhen you add the digits together, it makes 10...\nIt's bigger than 73...")
# Prompts user to suggest another number.
third_answer = input("\nWhat is the number? ")
# Number changed from string to integer.
third_answer = int(third_answer)
# Determines if the number picked by user is 82; if incorrect - gives final hint.
if third_answer == 82:
print("\nYou got it this time! Good game {}!!!\n\nIf you'd like to play again you can type in:".format(name))
exit("python numbers2.py")
else:
print("\nAlmost there! Remember: this number has 2 digits that add to 10...\n\nIt's bigger than 73... and I'll give you another hint!\n\nIt's not 91...\n")
#Prompts user to suggest another number.
fourth_answer = input("\nYou got it now? What's the number? ")
# Number changed from string to integer.
fourth_answer = int(fourth_answer)
#Determines if the number picked by the user is 82; if incorrect - the user loses.
if fourth_answer == 82:
print("\nYES! You got it! Nice game, {} :)".format(name))
else:
print("\nNope - it was 82! Better luck next time {}!".format(name))````

Samuel Savaria
4,809 PointsAt this point I would recommend finishing this course. Loops and functions are gonna make your code so much cleaner and efficient, and I think he gives you advice on how to write your own code in the last section.
Once you have a more efficient program you can re-post it in here or you can send me a private message. A number guesser is one of the first 3 programs I write when learning a language so I can also send you my version if you want to see another example.
RNG aren't covered in this track but they can be pretty simple so I can show you how they work real quick if you want.
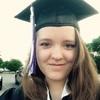
Chandelor Simon
2,242 PointsI would love to see your number program and any clarification you have on RNG.
As I learn more, I'll keep cleaning this program up and add it in case someone can use it. It'll probably be helpful for someone to see how I got from A to B.
Thanks for the help - happy coding!

Samuel Savaria
4,809 PointsI just noticed that you can't message someone on Treehouse, so do you have a Skype or a Discord or something to communicate?
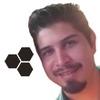
Fernando Balandran
492 PointsNice game Chandelor Simon. Note that I am not a beginner, so don't get discourage if you think my program is advanced.
As Samuel Savaria said this game needs to be done with a loop. I did like the way you said the two numbers added to 10, kinda gives you the answer but is nice. A few things I didn't implement in my program is hints, you could add another function that breaks numbers apart like you did for 8 + 2 = 10. You could add another function to count the number of guesses before you get hints, etc. This game is really fun and there are tons of things you can do with it. The obvious way would be with random class. Let me know if you have any questions regarding the code.
import random
def yes_or_no(question):
while "the answer is invalid":
reply = str(input(question+' (y/n): ')).lower().strip()
if reply[0] == 'y':
return True
if reply[0] == 'n':
return False
name = input("Hello - what's your name? ")
is_playing_game = yes_or_no("Would you like to play a game?")
is_answer_correct = False
if not is_playing_game:
print("\nOkay - maybe next time! You can always type this to play the game:\n")
# game loop
while is_playing_game:
random_num = random.randint(1, 100)
print("\nGreat, let's get started!\n\nI'm thinking of a number between 1 and 100...")
first_answer = input("Can you guess what number it is? ")
while not is_answer_correct:
# Number changed from string to integer.
first_answer = int(first_answer)
# Determines if the number picked by user is random_num, higher, or lower; if incorrect - gives hint.
if first_answer == random_num:
is_answer_correct = True
print("\nWay to go! That's the number I was thinking of!!!\n")
elif first_answer > random_num:
print("\nGood guess - but not quite! The number I'm thinking of is less than that.")
elif first_answer < random_num:
print("\nGood guess - but not quite! The number I'm thinking of is higher than that.")
else:
print("\nThat's not a valid guess; the number I'm thinking of is between 1-100\nTry again!")
first_answer = input("Can you guess what number it is? [options: exit] ")
# break out of inner while loop
if first_answer == "exit":
is_playing_game = False
break
print("Thank you for playing {}".format(name))
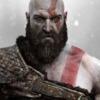
boi
14,242 Pointshey guys!!, Nice game chandelor! ....... Fernando, sir your program is giving several indentation errors.
Samuel Savaria
4,809 PointsSamuel Savaria
4,809 PointsI just realised you can't message people directly on Treehouse. So do you have a Skype or a Discord or something to communicate?