Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial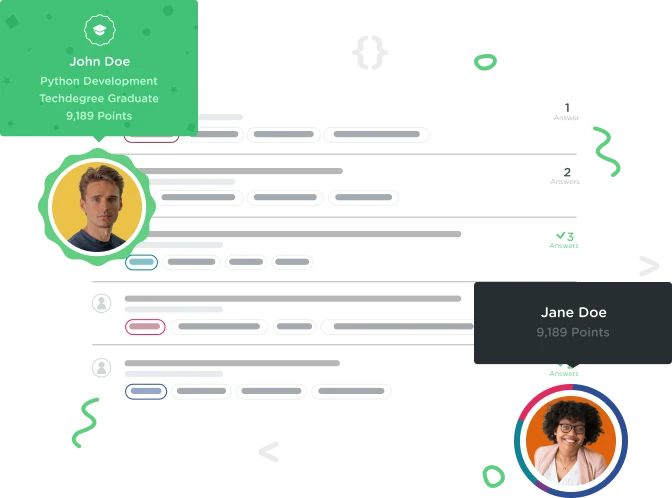

Faithe Yates
806 PointsCan you not use the sign "=" when referring to strings?
It seems you can use if (age = 40) but you can't say if (name = "Craig") instead you have to put if(name.equals("Craig")
is that just because it's a string value? Does it just evaluate it differently based on type?
Because it seems odd considering you use "=" to set the value in the first place...
I'm confused by the difference in {String name = "Craig"} vs {if (name = "Craig")}
3 Answers
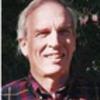
jcorum
71,830 PointsOK, Jennifer has explained = vs ==, but what about equals()?
equals() is used to compare objects. It's a method inherited by all classes from Object.
Consider this code:
String a = new String("Hi")
String b = new String("Hi")
a == b //false
a.equals(b) //true
Here we create two String variables, a and b, each of which points to a new String object, "Hi". The two object variables, a and b, refer/point to separate objects, so a == b is false. But the two String objects that a and b refer to are the same (have the same character sequences) and so a.equals(b) returns true.
Note that equals(), unless overridden, asks if the two objects' memory locations are the same. But since it is overridden in the String class, it returns true if the state of the two Strings are the same (and it ignores their memory locations). You've probably also heard that if you create a class of your own you should override equals() -- and that is correct. That's because you will also want to compare the state of your class's objects, not their memory locations.
Just to make things interesting, though, consider this code:
String x = "Hi"
String y = "Hi"
x == y
true
x.equals(y)
true
Here we have used the shortcut method to create two Strings. As before we have two String variables, x and y, both of which point to a String object, "Hi".
In this case, however, the memory locations in x and y (the locations they point to) must be the same, since x == y is true. Java has taken a shortcut itself. Since it already had a "Hi" String, it didn't create a second one. It just made y a second name for the same object.
There's a more detailed explanation of this gotcha, and several others, at: https://www.owasp.org/index.php/Java_gotchas
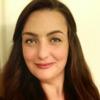
Jennifer Nordell
Treehouse TeacherHi there Faithe Yates! This is a common question when starting with a programming language.
A single equals sign is called an "assignment operator". And there's a reason for this. It's because we're assigning a value to that variable. You've probably heard by now that you can think of a variable as a box that holds a piece of data. The equals here puts a value in the box.
x = 10;
y = 15;
name = "Jane Doe";
These are all assignments. We're telling the variable what it has stored in there... not asking.
We use the double equals in a conditional expression. Here we're asking what their value is, because we don't really know.
if( x == 10) {
}
Here we're saying: Ok, if x is equal to 10 please run the code inside these brackets. Hope that clarifies things a bit!
edited for additional note
Some languages can directly compare strings to each other. However, Java implements this a little differently with the .equals method.

taylornajjar
15,807 PointsIn Java a single = sign is always used to assign a value to a variable. You wouldn't type if (age = 40), as you say, but rather if (age == 40) to make a comparison.
= is used to assign a value to a variable
== is used for object comparisons. It checks the memory addresses of your objects, meaning it checks to see whether it's the same object.
.equals is a method that allows you to compare whether the characters in your given string are the same as the characters in the string you're comparing against, even if they're not actually the same object.