Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial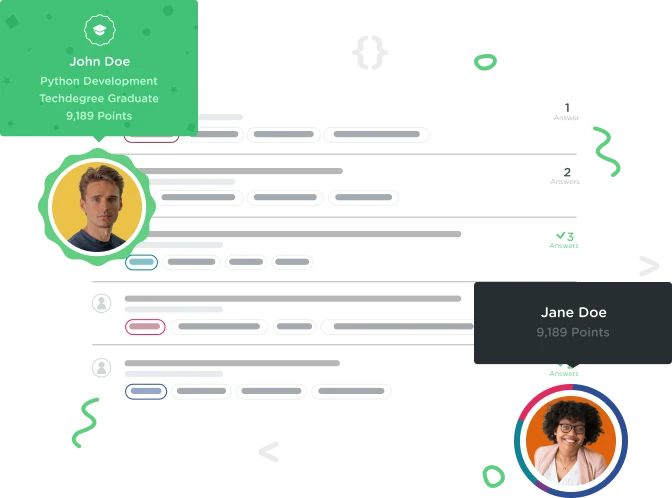

praveen gudimetla
464 PointsCan you please add a new test that verifies that on a successful vend operation that the running sales total is incremen
Hi,
running sales total is not found in below code
package com.teamtreehouse.vending;
import org.junit.Before;
import org.junit.Test;
import static org.junit.AssQert.*;
public class VendingMachineTest {
private VendingMachine machine;
public class NotifierSub implements Notifier {
@Override
public void onSale(Item item) {
return;
}
}
@Before
public void setUp() throws Exception {
Notifier notifier = new NotifierSub();
machine = new VendingMachine(notifier, 10, 10, 10);
machine.restock("A1", "Twinkies", 10, 30, 75);
}
@Test
public void vendingWhenStockedReturnsItem() throws Exception {
machine.addMoney(75);
Item item = machine.vend("A1");
assertEquals("Twinkies", item.getName());
}
}
do you mean onSale() method. I was not clear on question and solution eithr. please advise
com/teamtreehouse/vending/VendingMachineTest.java
package com.teamtreehouse.vending;
import org.junit.Before;
import org.junit.Test;
import static org.junit.AssQert.*;
public class VendingMachineTest {
private VendingMachine machine;
public class NotifierSub implements Notifier {
@Override
public void onSale(Item item) {
return;
}
}
@Before
public void setUp() throws Exception {
Notifier notifier = new NotifierSub();
machine = new VendingMachine(notifier, 10, 10, 10);
machine.restock("A1", "Twinkies", 10, 30, 75);
}
@Test
public void vendingWhenStockedReturnsItem() throws Exception {
machine.addMoney(75);
Item item = machine.vend("A1");
assertEquals("Twinkies", item.getName());
}
}
package com.teamtreehouse.vending;
public class VendingMachine {
private final Notifier notifier;
private final AbstractChooser chooser;
private final Creditor creditor;
private final Bin[][] bins;
private int runningSalesTotal;
public VendingMachine(Notifier notifier, int rowCount, int columnCount, int maxItemsPerBin) {
this.notifier = notifier;
chooser = new AlphaNumericChooser(rowCount, columnCount);
creditor = new Creditor();
runningSalesTotal = 0;
bins = new Bin[rowCount][columnCount];
for (int row = 0; row < rowCount; row++) {
for (int col = 0; col < columnCount; col++) {
bins[row][col] = new Bin(maxItemsPerBin);
}
}
}
public void addMoney(int money) {
creditor.addFunds(money);
}
public int refundMoney() {
return creditor.refund();
}
public Item vend(String input) throws InvalidLocationException, NotEnoughFundsException {
Bin bin = binByInput(input);
int price = bin.getItemPrice();
creditor.deduct(price);
runningSalesTotal += price;
Item item = bin.release();
notifier.onSale(item);
return item;
}
public int getRunningSalesTotal() {
return runningSalesTotal;
}
public void restock(String input, String name, int amount, int wholesalePrice, int retailPrice) throws InvalidLocationException {
Bin bin = binByInput(input);
bin.restock(name, amount, wholesalePrice, retailPrice);
}
private Bin binByInput(String input) throws InvalidLocationException {
AbstractChooser.Location location = chooser.locationFromInput(input);
return bins[location.getRow()][location.getColumn()];
}
}