Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial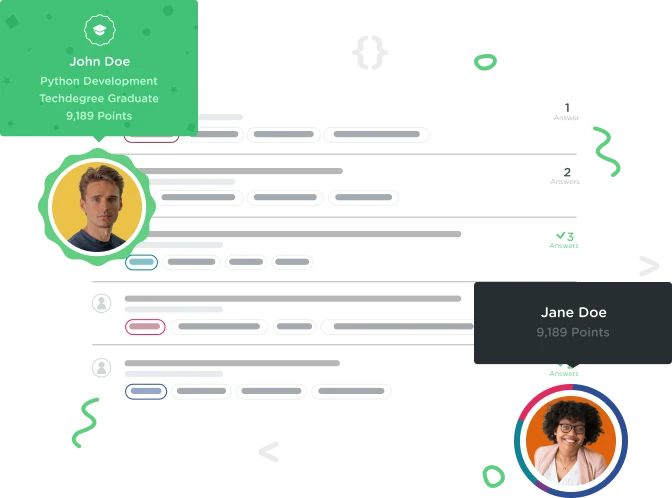

ori
2,307 PointsCan you please check if my solution works?
I tried to solve the challenge before watching the video, and here is how I did it. Can you please let me know if I missed anything conceptually or if I should have done anything better (based on best standards)? Thanks so much.
var products = ["Banana", "Bread", "Apple", "Orange", "Cottage Cheese", "Egg"];
var productList = products.join(', ');
for (i = 0; i < products.length; i += 1) {
var search = prompt("Search for a product in our store. Type 'list' to show all of the produce and 'quit' to exit.").toLowerCase();
productSearch = products.indexOf(search);
if (search === "list" ) {
document.write(productList + "<br />");
} else if (search === "quit") {
document.write("Okay..." + "<br />");
} else if (productSearch !== -1) {
inStock = true;
document.write("Yes, we have " + search + " in stock" + "<br />");
} else {
inStock = false;
document.write(search + " is not in stock" + "<br />");
}
}
2 Answers

Bradley Weston
Courses Plus Student 360 PointsIn future can you use Markdown syntax to add code
tags around your code snippets to allow for readability.
If you're on Chrome you can press F12
and in the console tab test your code in there.
Also you don't need to use the a new line to represent a new line the following works using escape sequences. http://www.delorie.com/gnu/docs/gawk/gawk_27.html
var myMultiLineComment = "my\n\rmultiline\n\rcomment";
But if you just want a HTML new line try wrapping your snippet in p
tags.

ori
2,307 PointsI'm trying to format the code but it's not working for me... Am I supposed to add the following?
<code>
</code>
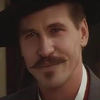
huckleberry
14,636 Pointsori, to make your code show up right you need to place three backticks <code>```</code> and then the name of the language that makes up the code. Then hit enter a couple of times. Paste/write your code. Hit enter a couple of times and then put 3 more backticks. Like so
so <code>```</code> javascript
your code here
end with <code>```</code>
Here's your code wrapped in the proper syntax for the forums code pasting.
var products = ["Banana", "Bread", "Apple", "Orange", "Cottage Cheese", "Egg"];
var productList = products.join(', ');
for (i = 0; i < products.length; i += 1) {
var search = prompt("Search for a product in our store. Type 'list' to show all of the produce and 'quit' to exit.").toLowerCase();
productSearch = products.indexOf(search);
if (search === "list" ) {
document.write(productList + "<br />");
} else if (search === "quit") {
document.write("Okay..." + "<br />");
} else if (productSearch !== -1) {
inStock = true;
document.write("Yes, we have " + search + " in stock" + "<br />");
} else {
inStock = false;
document.write(search + " is not in stock" + "<br />");
}

Iain Simmons
Treehouse Moderator 32,305 PointsSo, you've probably watched the video by now, but a few comments/suggestions:
First, because your list has capital letters and you're not converting them or the search term, the user would need to search with the exact same string, including the capital letters. i.e. banana
or Cottage cheese
would not work, or rather, would tell the user that they are not in stock. Dave uses a combination of a list of only lowercase products and converting the search term to lowercase to solve this.
Second, you have a few variables that you're not declaring before using. The first is the loop index variable i
, the second is productSearch
, and the last one is inStock
, which you actually not even using for anything and therefore probably isn't required.
Third and probably most importantly, you're using a for
loop, which will have a limited number of iterations or cycles before it finishes. In this case, the user only has 6 opportunities to search through or list the products before the loop finishes and no longer prompts them for a search term.
You'll need a while
or do ... while
loop instead, and need to use break
if they enter quit
.
Lastly, you're not really changing anything if the user tries to quit, you're just printing a different message. You can also use break
in a for loop, but again, it's not the best loop for this scenario anyways.
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsI added a code block around your code to make it easier to read.