Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial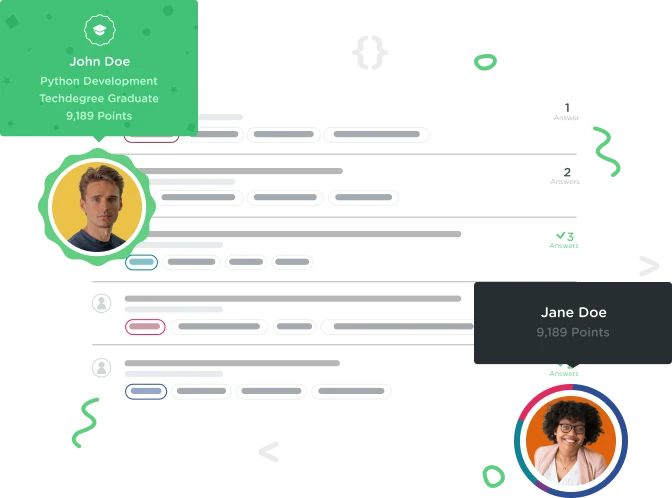

Andrei Oprescu
9,547 PointsCan you transform a string to a name of a method/function?
I am trying to create a program in python that is pretty difficult to make. I have come across a constant need to transform a string to a name of a method.
For example, I have a method called 'guessed()' and I want to call that method if a string that i get from a string is matching with that method's name. Let's say the string I use contains the string 'guessed'. Can a create a code that will call that method if it has a matching name as that string?
Thanks!
2 Answers
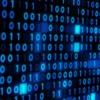
Alexander Davison
65,469 PointsIf you must use strings—be warned though, using eval
is dangerous—you can use the built-in function eval
. Read the Python Docs for eval
or simply call the built-in help
function on eval
for more information. It is unsafe to use, though, because if the user typing into the input is a clever Python programmer, he can do dangerous stuff like stopping your server or hacking your project.
If possible, do not use strings that represent the function name. You can do so in Python, as Python is also functional:
def say_hello(name):
print("Hello {}!".format(name))
def say_bye(name):
print("Goodbye {}!".format(name))
say_hello("Bob") # Prints "Hello Bob!"
say_bye("Bob") # Prints "Goodbye Bob!"
funcs = [say_hello, say_goodbye]
for func in funcs:
func("Bob")
# Prints:
# Hello Bob!
# Goodbye Bob!
For more on functional programming in Python, watch the Treehouse course on Functional Python.
Happy coding! ~Alex

Andrei Oprescu
9,547 PointsHi!
I have read your comment and it was very helpful, but I have some questions on the function func:
Can it work on strings? And if yes, then how would the code look like?
Would it work on a string that is named as a method? e.g. If I have a code that would change a string called 'my_string' and output it to another string:
"self.{}".format(my_string)
Then can I use func to call that method?
If you know the answer, please reply to this comment.
Thanks!
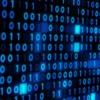
Alexander Davison
65,469 Points I don't recommend this: it isn't safe to use. The
eval
function can evaluate any expression, and that might be dangerous.
But if this is just some little thing you are building, using eval
is fine.
To call a function (from self
):
eval('self.{}()'.format(my_string))