Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial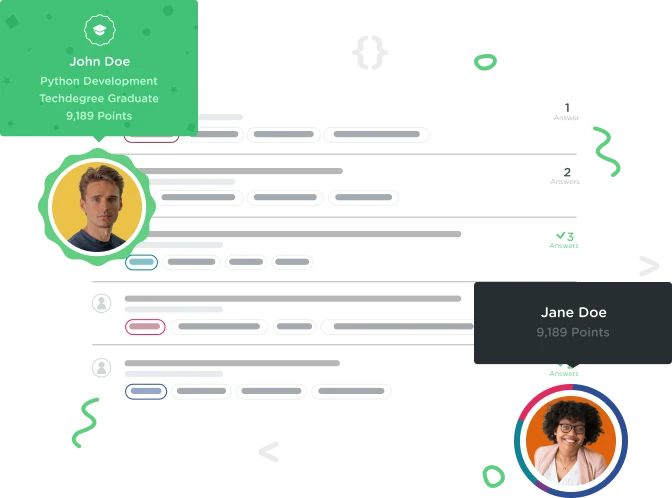
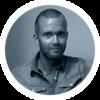
Daniel Fitzhugh
9,715 PointsCan you use for/in loops on Objects that are contained within Arrays ?
Let's say that I wanted to loop through the first Object within this Array --- the entry for "Dave" --- and wanted to console.log() ALL of the property values, regardless of how long this particular Object might be.
var students = [
{ name: 'Dave', track: 'Front End Development', achievements: 158, points: 14730 },
{ name: 'Jody', track: 'iOS Development with Swift', achievements: '175', points: '16375' },
{ name: 'Jordan', track: 'PHP Development', achievements: '55', points: '2025' },
{ name: 'John', track: 'Learn WordPress', achievements: '40', points: '1950' },
{ name: 'Trish', track: 'Rails Development', achievements: '5', points: '350' }
];
If the Object was a standalone object not contained within an Array, I would use a for/in loop like this:
for ( prop in objectVariableName ) {
console.log( objectVariableName[prop] );
}
But since Objects within an Array don't have variable names, I don't think a for/in loop would work. Am I wrong ?
Is there another way to do what I'm trying to do ?
2 Answers
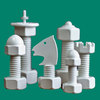
Steven Parker
231,269 PointsYou could give a specific object a name and do exactly what you have:
var objectVariableName = students[0];
Or, just substitute a specific index on the array for the object name:
for ( prop in students[0] ) {
console.log( students[0][prop] );
}
You could also iterate through the entire array if you wanted to see all properties of all objects:
for (student of students) {
for (prop in student) {
console.log(student[prop]);
}
}
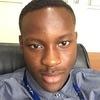
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsHi Daniel, you can still use your 'for in' loop. You just need to declare the specific object in the array you want to iterate over(which is the first object as you claimed). Take for example:
var students = [
{ name: 'Dave', track: 'Front End Development', achievements: 158, points: 14730 },
{ name: 'Jody', track: 'iOS Development with Swift', achievements: '175', points: '16375' },
{ name: 'Jordan', track: 'PHP Development', achievements: '55', points: '2025' },
{ name: 'John', track: 'Learn WordPress', achievements: '40', points: '1950' },
{ name: 'Trish', track: 'Rails Development', achievements: '5', points: '350' }
];
var firstObject = students[0];
for ( prop in firstObject) {
console.log( firstObject[prop] );
}
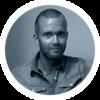
Daniel Fitzhugh
9,715 PointsThanks Osaro, another great answer :)
Daniel Fitzhugh
9,715 PointsDaniel Fitzhugh
9,715 PointsThanks Steven, great answer :)
We haven't learnt about "for/of" loops yet so I'll look into that.