Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial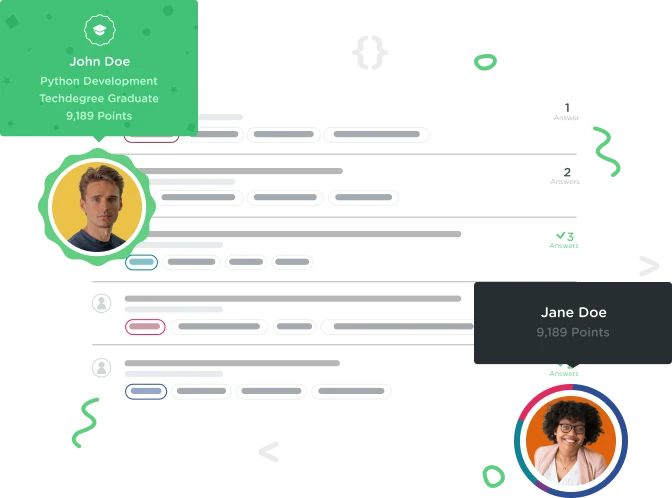

Ryan Lail
4,386 PointsCan you use string interpolation with enum?
In the results pane doesn't show the day when i've used string interpolation (not sure if i've got that spelling right) - but rather it shows '(Enum Value)'
http://www.mediafire.com/view/e1171cyd0et6cio/Screen_Shot_2015-08-13_at_21.54.17.png
2 Answers

Andrew Rodko
27,881 PointsYeah, to convert an enum to a string you'd need to specify the string value for each enum. One way to do this is with raw values. So your enum would become:
enum Day: String { case Monday = "Monday" case Tuesday = "Tuesday" etc.... }
Your string interpolations would then become (dayOfWeek.rawValue) instead of (dayOfWeek)
You could also clean it up a bit by moving your function inside your enum. If you do that, the function would become:
func weekdayOrWeekend() -> String { switch self { case .Monday, etc...: return "(self.rawValue) is a weekday!" and so on... } }
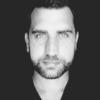
Jhoan Arango
14,575 PointsHey Ryan : I’m using the latest version of swift, and Xcode and can’t post any screenshots until is out of beta. But I am not 100% sure, but I think what you are trying to do is possible in swift 2.0 and the latest Xcode releases. This is my code
enum Day {
case Monday, Tuesday
}
func whatday(enumDay: Day) {
switch enumDay {
case .Monday, .Tuesday :
print("The day is \(enumDay)")
}
}
whatday(Day.Monday)
// on the results pain shows me “The day is Monday"
So perhaps its an upgrade coming up with the latest versions of Swift and Xcode. I think I did see on the apple’s Swifts 2.0 new features keynote video something about swift not showing “Enum Value” anymore.
Hope this information helps

Ryan Lail
4,386 PointsThanks, looking forward for Swift 2.0's release!!!
Ryan Lail
4,386 PointsRyan Lail
4,386 PointsThanks for the help! Do you think you could explain the moving my function inside my enum part a bit more. It looks very interesting but I'm a bit confused about how to do it? Thank you once again!
Andrew Rodko
27,881 PointsAndrew Rodko
27,881 PointsSure, here's what your entire enum would like like. After moving the function inside the enum, you just need to remove the dayOfWeek input parameter from the function, and replace any reference to this parameter inside the function with "self." Calling the function would then be done like this:
var today = Day.Monday today.weekdayOrWeekend()
or
Day.Monday.weekdayOrWeekend()
enum Day: String { case Monday = "Monday" case Tuesday = "Tuesday" case Wednesday = "Wednesday" case Thursday = "Thursday" case Friday = "Friday" case Saturday = "Saturday" case Sunday = "Sunday"
}