Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial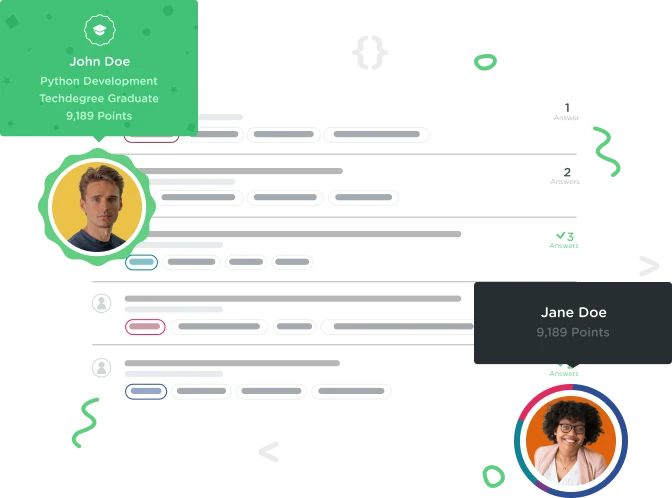

Jeff Rutherford
2,565 PointsCan you use switch for FizzBuzz challenge?
I've looked through many questions on this challenge and they all use the If/if else control flow.
Could switch work too?
I've tried this code, but it's not working.
switch n { case (n % 3 == 0): return "Fizz" case (n % 5 == 0): return "Buzz" default: return "FizzBuzz" }
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
// End code
return "\(n)"
}
4 Answers

rh12
4,407 PointsYou are close :)
The case statements are expecting expressions that are compatible with the type that you are switching on. In your code you are switching on an Int but are producing Booleans in your cases.
Instead you could use pattern matching in the switch statement:
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch n {
case let x where (x % 3 == 0):
return "Fizz"
case let x where (x % 5 == 0):
return "Buzz"
default:
return "FizzBuzz"
}
// End code
//return "\(n)"
}
The where statement allows for matching on a Boolean expression.
That said, i do not think the above code satisfies the requirement for this particular challenge. It seems they are looking for an if statement.

Jeff Rutherford
2,565 PointsThank you Ryan. Were they clear in instructions about if statement? Not questioning you.
I was confused, because it went straight from the 2-3 switch statement videos into this challenge, and I thought, "Oh, I should use a switch statement to solve this." :)
But, yeah, I don't think they've covered the where statement.
Thanks again.

rh12
4,407 PointsYou're welcome!
I agree, it wasn't very clear in the instructions. I based it on step 2 saying "For example if (n % 3 == 0)..." and to a lesser extent the "return (n)" code just after the "// End code" comment. If you are using a switch statement, that last return statement would never be called because the switch statement would need a default case that itself could return something. Unless the default case did't return anything, then their final return statement would make sense.
That said, if they showed 2-3 switch statement videos before this challenge I would be thinking the same as you :)
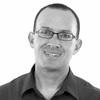
David Papandrew
8,386 PointsJeff, I had the same question as you and wanted to use a switch statement (since I'm not familiar with them).
I used this solution and it was accepted:
func fizzBuzz(n: Int) -> String {
switch (n%3, n%5) {
case (0, 0): return "FizzBuzz"
case (0, 1..<5): return "Fizz"
case (1..<3, 0): return "Buzz"
default: return "\(n)"
}
}