Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial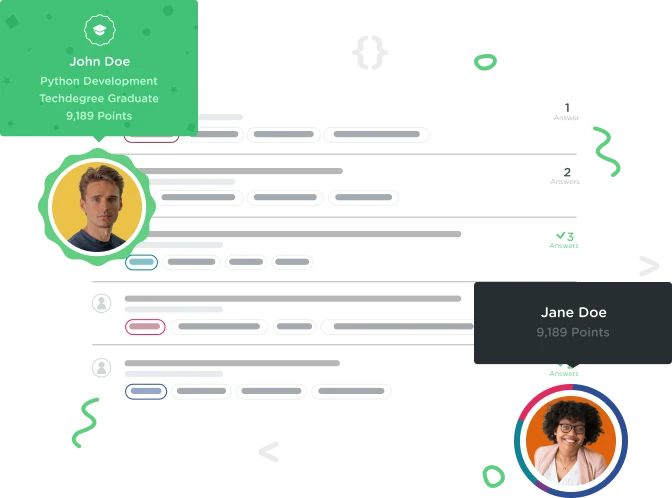

Pavel Rogulin
5,036 PointsCancel button
What if after i pressed edit button i want to cancel editing and return to previous state. For example when we press edit we change textContent of edit button from Edit to Save and for remove button we can change textContent from Remove and Cancel. But what next? How can i get previous value of elements to cancel editing? Need help.
3 Answers

Pavel Rogulin
5,036 PointsMy solution was when clickin' edit button we don't remove preivous element until we hit save button. We just hide it using .style.display = "none". So if we click cancel we'll make previous element visible again and delete the input.

Boyan Anakiev
5,536 PointsThis is how I did it, I didn't think too hard about it so maybe it's not the ideal way since I ended up using a global variable to preserve the span text (so that I can later retrieve it and put it back in to the span) but this way you add a seperate cancel button once you click edit:
ul.addEventListener('click', (e) => {
if (e.target.tagName === 'BUTTON') {
const button = e.target
const li = button.parentNode;
const ul = li.parentNode;
if (button.textContent === 'remove') {
ul.removeChild(li);
} else if (button.textContent === 'edit') {
const span = li.firstElementChild;
cancelSpanText = span.textContent;
const cancelButton = document.createElement('button')
cancelButton.textContent = 'cancel';
const input = document.createElement('input');
li.insertBefore(cancelButton, button.nextElementSibling);
input.type = 'text';
input.value = span.textContent;
li.insertBefore(input, span);
li.removeChild(span);
button.textContent = 'save';
} else if (button.textContent === 'save') {
const input = li.firstElementChild;
const span = document.createElement('span');
span.textContent = input.value;
li.insertBefore(span, input);
li.removeChild(input);
button.textContent = 'edit';
} else if (button.textContent === 'cancel'){
const input = li.firstElementChild;
const span = document.createElement('span');
span.textContent = cancelSpanText;
li.insertBefore(span, input);
button.previousElementSibling.textContent = 'edit';
li.removeChild(input);
li.removeChild(button);
}
}
});
As you can probably see, there's some added code for the edit button:
cancelSpanText = span.textContent;
const cancelButton = document.createElement('button')
cancelButton.textContent = 'cancel';
So, when you click the edit button, you a) save the current span content in the global variable cancelSpanText, then b) you create a cancelButton , then c) you add it between your existing buttons with:li.insertBefore(cancelButton, button.nextElementSibling);
And finally, you just have another elif statement that would tell the program what you want to do if the user clicks the cancel button, it's almost all copy paste from the save button but in this case we set the span value to the global variable cancelSpanText which we saved and we also remove the 'cancel' button we previously created as well as the input form.

Pavel Rogulin
5,036 PointsYeah, i had a similar idea(to store textContext in a variable). But there is one problem, the variable will be rewriten every time you hit Edit button. For example, you can click Edit on several elements(without clicking Cancel) and cancelSpanText will store the value of the last element only.

Boyan Anakiev
5,536 PointsYep, I can see that now. I'm sure there's another way to overcome this issue but I can't think of it right now :D.