Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial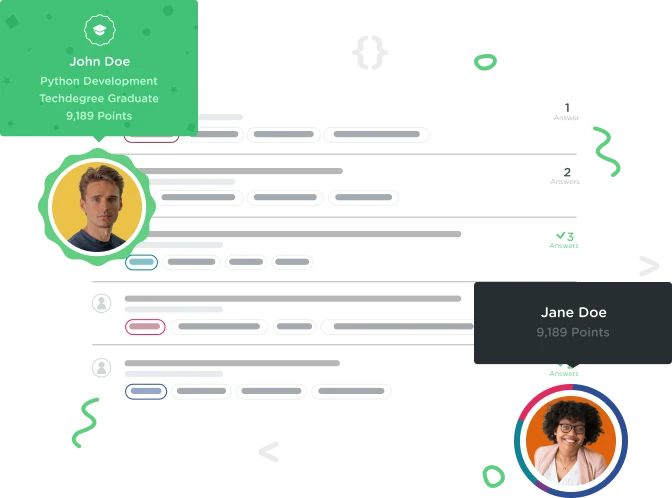
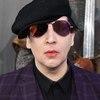
Adham Ali
Courses Plus Student 1,112 PointsCannot access the setter of the property
Let's assume we have an event called RoundLeveledUp, a property called CurrentRound, that has a private setter. And finally a method called OnRoundLeveledUp(object sender, EventArgs e) which fires the event if it's not null.
First let's represent this in code
public event EventHandler RoundLeveledUp;
public int CurrentRound{get; private set;}
public void OnRoundLeveledUp(object sender, EventArgs e)
{
//Firing the event if it's not *null*
RoundLeveledUp?.Invoke();
}
and we want to expose our property so that when any body set it value some event is triggered when the value of CurrentRound is changed we do this:
int _currentRound;
public int CurrentRound
{
get=>_currentRound;
private set
{
_currentRound=value;
OnRoundLeveledUp(this, null);
}
}
till now, we (or i can say "I") 100% good ! When i attempt to set CurrnetRound from a method with-in the class called Start() and i set a break point to see. Actually it doesn't jump to the setter and fire the event after setting the value Why is that for
thanks in advance!
7 Answers
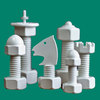
Steven Parker
231,122 PointsWhen does "RoundLeveledUp" get set? In the code shown here it is declared but never set, so the method with the null-coalescing membership operator would never get called.
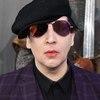
Adham Ali
Courses Plus Student 1,112 PointsU mean when did we subscribed to the event? Even when I put a break point it doesn't jump to the OnRoundLeveledUp
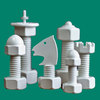
Steven Parker
231,122 PointsWhere's the breakpoint? Inside the setter? Are you sure you're doing "step into" and not "step over"?
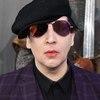
Adham Ali
Courses Plus Student 1,112 PointsI have a method called start which calls OnRoundLeveledUp(object source, EventArgs e) I put a break point in the call of Start() in the Main method
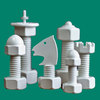
Steven Parker
231,122 PointsAnd did you "step into" all the way through the calls it makes?
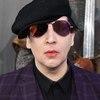
Adham Ali
Courses Plus Student 1,112 Pointsfirst I step into the Start() method walk throw each line and If this line is a call for some another method it jumps to it. and after returning the value or after the work is finished (if it's void) it returns to the start method and continues executing lines when I set the CurrentRound property, it doesn't jump to the setter or didn't even execute call OnCurrentRoundLeveledUp to fire up the event
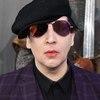
Adham Ali
Courses Plus Student 1,112 Pointsyep. you know the down arrow in visual studio 2017 which u use in debugging are breakpoints right ? I click this little button
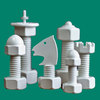
Steven Parker
231,122 PointsDo you have a repo or workspace to share the entire project for a more complete analysis?
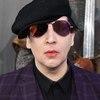
Adham Ali
Courses Plus Student 1,112 Pointsunfortunately, i dont have any. in face u dont need a repo to get what I`m tyin to say. What i'm tryna say : simply the property is been set by the method I put in the setter in order to execute some event doesn't get called
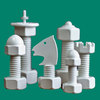
Steven Parker
231,122 PointsI understand the issue as you have described it, but I don't see anything in the code snippets you have shown here that might explain it. I think at this point it would be necessary to replicate your environment using the complete code to be able to observe the problem and to provide an analysis and a solution to the issue.
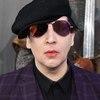
Adham Ali
Courses Plus Student 1,112 PointsWell... you can copy all the code I written, put it in a class and execute it using any online compiler and u will get what i'm tryin' to say