Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial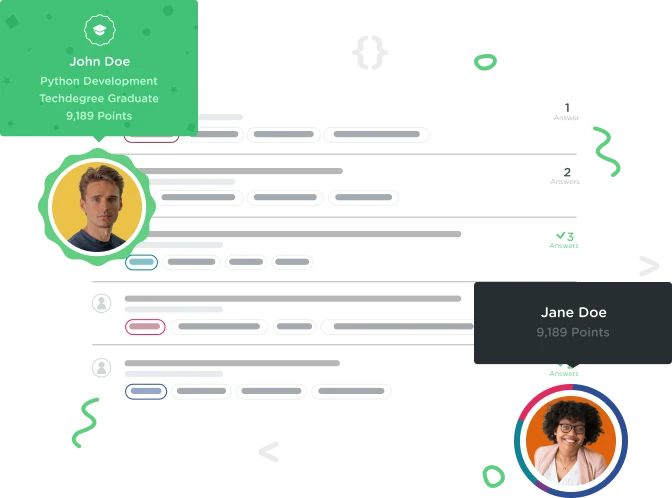
Ryan Doyle
8,587 PointsCannot access values of JSON objects
I'm having an issue with the following code:
function printCurrent(location, time, temp) {
const message = `In ${location} it is currently ${temp}. As of: ${time}`;
console.log(message)
}
const https = require('https');
const http = require('http');
const api = require('./api.json');
function get(location) {
const req = https.get(`https://api.wunderground.com/api/${api.key}/forecast/conditions/q/${location}.json`, response => {
let body = "";
response.on('data', data => {
body += data.toString(); // adds to body each time a data packet is received
});
response.on('end', data => {
const forecast = JSON.parse(body);
console.log(forecast.current_observation.display_location.full);
});
})
}
get();
module.exports.getLocation = get;
I cannot seem to access any of the actual data from the "forecast" object. I can get the type of as an object, and print out the forecast and it looks like an object, but then if I try to access anything, I get: "TypeError: Cannot read property 'display_location' of undefined"
I believe it has to do with the fact that it seems to return 2 objects. (If I do typeof forecast, I get 2 objects). But I'm not sure why that's happening either :/
2 Answers

Myers Carpenter
6,421 PointsYou are really close, let's see if I can give you a nudge:
I ran your code and got
/Users/myers/forum/weather.js:19
console.log(forecast.current_observation.display_location.full);
^
TypeError: Cannot read property 'display_location' of undefined
at IncomingMessage.response.on.data (/Users/myers/forum/weather.js:19:53)
at emitNone (events.js:110:20)
at IncomingMessage.emit (events.js:207:7)
at endReadableNT (_stream_readable.js:1059:12)
at _combinedTickCallback (internal/process/next_tick.js:138:11)
at process._tickCallback (internal/process/next_tick.js:180:9)
so let's explore what is in the forecast variable, with console.log(forecast);
{ response:
{ version: '0.1',
termsofService: 'http://www.wunderground.com/weather/api/d/terms.html',
features: { forecast: 1, conditions: 1 },
error:
{ type: 'querynotfound',
description: 'No cities match your search query' } } }
Ok, so we called get()
with no arguments and that meant we didn't ask for any location, what if we do get("IAD")
(that's Washington Dulles International, VA), and IT WORKS!
{ response:
{ version: '0.1',
termsofService: 'http://www.wunderground.com/weather/api/d/terms.html',
features: { forecast: 1, conditions: 1 } },
current_observation:
{ image:
{ url: 'http://icons.wxug.com/graphics/wu2/logo_130x80.png',
title: 'Weather Underground',
link: 'http://www.wunderground.com' },
display_location:
{ full: 'Washington Dulles International, VA',
city: 'Washington Dulles International',
state: 'VA',
state_name: 'Virginia',
country: 'US',
country_iso3166: 'US',
zip: '20101',
magic: '5',
wmo: '99999',
latitude: '38.94444275',
longitude: '-77.45555878',
elevation: '95.0' },
observation_location:
{ full: 'Dulles Airport, Virginia',
city: 'Dulles Airport',
state: 'Virginia',
country: 'US',
country_iso3166: 'US',
latitude: '38.93999863',
longitude: '-77.45999908',
elevation: '312 ft' },
estimated: {},
station_id: 'KIAD',
observation_time: 'Last Updated on February 19, 3:00 PM EST',
observation_time_rfc822: 'Mon, 19 Feb 2018 15:00:00 -0500',
observation_epoch: '1519070400',
local_time_rfc822: 'Mon, 19 Feb 2018 15:29:04 -0500',
local_epoch: '1519072144',
local_tz_short: 'EST',
local_tz_long: 'America/New_York',
local_tz_offset: '-0500',
weather: 'Light Drizzle',
temperature_string: '46 F (8 C)',
temp_f: 46,
temp_c: 8,
relative_humidity: '96%',
wind_string: 'From the SSE at 12 MPH',
wind_dir: 'SSE',
wind_degrees: 160,
wind_mph: 12,
wind_gust_mph: 0,
wind_kph: 18,
wind_gust_kph: 0,
pressure_mb: '1027',
pressure_in: '30.33',
pressure_trend: '-',
dewpoint_string: '45 F (7 C)',
dewpoint_f: 45,
dewpoint_c: 7,
heat_index_string: 'NA',
heat_index_f: 'NA',
heat_index_c: 'NA',
windchill_string: '41 F (5 C)',
windchill_f: '41',
windchill_c: '5',
feelslike_string: '41 F (5 C)',
feelslike_f: '41',
feelslike_c: '5',
visibility_mi: '3.0',
visibility_km: '4.8',
solarradiation: '--',
UV: '1',
precip_1hr_string: '0.00 in (0.0 mm)',
precip_1hr_in: '0.00',
precip_1hr_metric: '0.0',
precip_today_string: '0.04 in (1.0 mm)',
precip_today_in: '0.04',
precip_today_metric: '1.0',
icon: 'rain',
icon_url: 'http://icons.wxug.com/i/c/k/rain.gif',
forecast_url: 'http://www.wunderground.com/US/VA/Washington_Dulles_International.html',
history_url: 'http://www.wunderground.com/history/airport/KIAD/2018/2/19/DailyHistory.html',
ob_url: 'http://www.wunderground.com/cgi-bin/findweather/getForecast?query=38.93999863,-77.45999908',
nowcast: '' },
forecast:
{ txt_forecast: { date: '2:27 PM EST', forecastday: [Array] },
simpleforecast: { forecastday: [Array] } } }
Washington Dulles International, VA
Ryan Doyle
8,587 PointsThanks! Yes, I realized it was calling get() twice. I had just the get() and then in another linked file, I was actually using the terminal to call get() again but with an argument. The empty one would always finish first and get me that first object.