Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial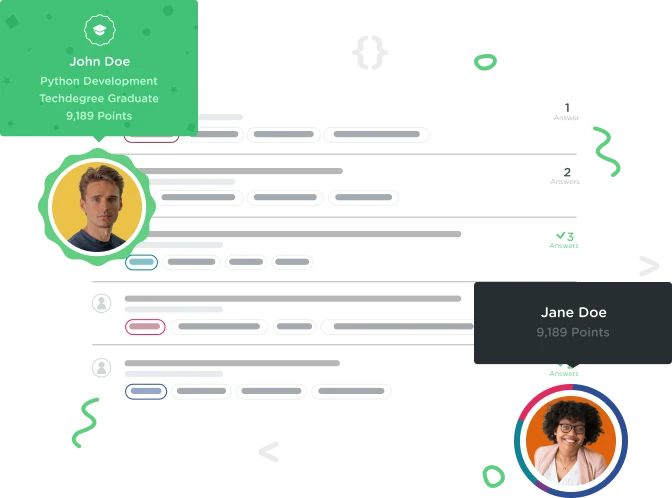

Kyle Papili
9,112 PointsCannot assign value of type '(String) -> Page' to type 'Page'
Within the prepareForSegue function, I am getting an error as I try to assign the constant pageController.page to Adventure.story. I am a bit confused as to why, hoping someone can help me figure this out! Thanks!
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if segue.identifier == "startAdventure" {
if let pageController = segue.destinationViewController as? PageController {
pageController.page = Adventure.story
}
}
}
InteractiveStory.swift
class Page {
let story: Story
typealias Choice = (title: String, page: Page)
var firstChoice: Choice?
var secondChoice: Choice?
init(story: Story) {
self.story = story
}
}
extension Page {
func addChoice (title: String, story: Story) -> Page {
let page = Page(story: story)
return addChoice(title, page: page)
}
func addChoice (title: String, page: Page) -> Page {
switch (firstChoice, secondChoice) {
case (.Some, .Some) : break
case (.None, .None), (.None, .Some) :
firstChoice = (title, page)
case (.Some, .None) :
secondChoice = (title, page)
}
return page
}
}
struct Adventure {
static func story(name: String) -> Page { //Static readonly computed property prevents against story being changed
let returnTrip = Page(story: .ReturnTrip)
let touchdown = returnTrip.addChoice("Stop and Investigate", story: .TouchDown)
let homeward = returnTrip.addChoice("Continue Home to Earth", story: .Homeward)
let rover = touchdown.addChoice("Explore the Rover", story: .Rover)
let crate = touchdown.addChoice("Open the Crate", story: .Crate)
homeward.addChoice("Head back to Mars", page: touchdown)
let home = homeward.addChoice("Continue Home to Earth", story: .Home)
let cave = rover.addChoice("Explore the Coordinates", story: .Cave)
rover.addChoice("Return to Earth", page: home)
cave.addChoice("Continue towards faint light", story: .Droid)
cave.addChoice("Refill the ship and explore the rover", page: rover)
crate.addChoice("Explore the Rover", page: rover)
crate.addChoice("Use the key", story: .Monster)
return returnTrip
}
}
1 Answer
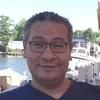
Safwat Shenouda
12,362 PointsHi Kyle, You are missing small part, the line in your prepareForSegue should read: pageController.page = Adventure.story(name)
Please note that Adventure.story is a function that takes a String and returns a Page object, it is defined like this in your code above: static func story(name: String) -> Page {..} So you have to give it the String which represent the story name, and receive back the Page object that will be displayed in the PageController.
I hope this helps. Cheers, Safwat