Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial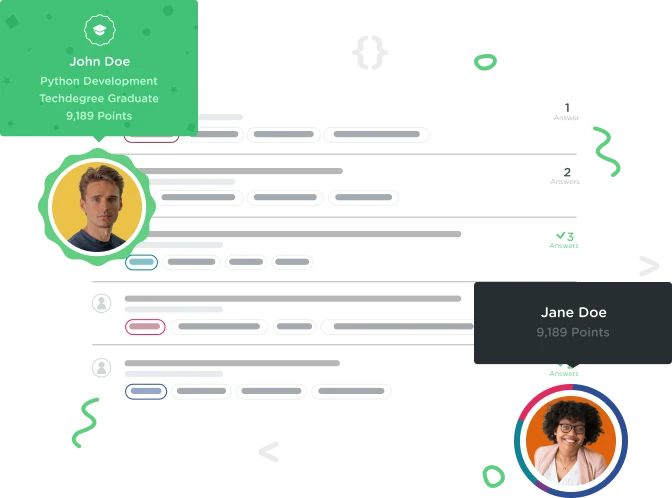

Emma Back
3,119 PointsCannot call the function correctly
Hi (again...) :)
Have tried conditional statements here in different variations, but cannot get it right. Would be super grateful if anyone could give me some advice!
// E
function max(number1, number2){
if (number1 = 1 || number1 = 'zero') {
alert('you are NOT right!');
}
else if (number2 => 3 || number2 === number2); {
alert('that is the highest number');
}
return number2;
}
max(1, 2);
1 Answer
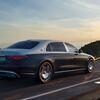
Balazs Peak
46,160 PointsYou have made a couple of mistakes. I think these are just beginner mistakes which will disappear eventually with practice. Try to give more attention to detail. This is a habit which will pay you great benefits while becoming a programmer.
- single equal signs are good for giving value, not for checking it. For checking, you should use double or triple, depending on wether you want to check the type also or not.
- when you are checking "greater than or equal" or "less than or equal" scenarios, you should put the ">" or "<" before the equal sign, not after it
- you even tried to compare a number to itself, but I think that was pure coincidence :D
The solution should look something like this. As you can see, I only changed the checking parts of the "if" and "else if" statements.
function max(number1, number2){
if (number1 === 1 || number1 === 'zero') {
alert('you are NOT right!');
}
else if (number2 >= 3 || number2 === number1); {
alert('that is the highest number');
}
return number2;
}
max(1, 2);
Benjamin Bock
5,998 PointsBenjamin Bock
5,998 PointsHey there,
in the challenge, it says, that you are supposed to return the higher number and not alert it directly from within the function.
Two little mistakes, that might get you into trouble:
Let me know if that helped.
Cheers Ben