Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial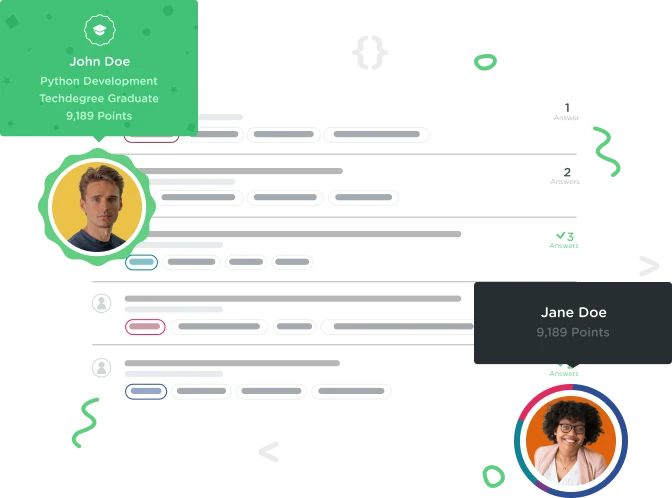
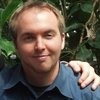
Eric Conklin
8,350 PointsCannot compile Prompter.java
Prompter.java will not compile in the hangman program section of the Java course after I added the code to determine if the game was won or not.
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public void play() {
while (mGame.getRemainingTries() > 0 && !mGame.isSolved()) {
displayProgress();
promptForGuess();
}
if (mGame.isSolved()) {
system.out.printf("Congratulations, you won with %d tries remaining. The answer was %s.", mGame.getRemainingTries(), mGame.getAnswer());
} else {
System.out.print("Bummer, the word was %s.", mGame.getAnswer());
}
}
public boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (! isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
try {
isHit = mGame.applyGuess(guessAsString);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again. \n", iae.getMessage());
}
}
return isHit;
}
public void displayProgress() {
System.out.printf("You have %d tries left to solve: %s\n",
mGame.getRemainingTries(),
mGame.getCurrentProgress());
}
}
The console is printing out:
Prompter.java:17: error: package system does not exist
system.out.printf("Congratulations, you won with %d tries remaining. The answer w
as %s.", mGame.getRemainingTries(), mGame.getAnswer());
^
Prompter.java:19: error: no suitable method found for print(String,String)
System.out.print("Bummer, the word was %s.", mGame.getAnswer());
^
method PrintStream.print(boolean) is not applicable
(actual and formal argument lists differ in length)
method PrintStream.print(char) is not applicable
(actual and formal argument lists differ in length)
method PrintStream.print(int) is not applicable
(actual and formal argument lists differ in length)
method PrintStream.print(long) is not applicable
(actual and formal argument lists differ in length)
method PrintStream.print(float) is not applicable
(actual and formal argument lists differ in length)
method PrintStream.print(double) is not applicable
(actual and formal argument lists differ in length)
method PrintStream.print(char[]) is not applicable
(actual and formal argument lists differ in length)
method PrintStream.print(String) is not applicable
(actual and formal argument lists differ in length)
method PrintStream.print(Object) is not applicable
(actual and formal argument lists differ in length)
Huh?
1 Answer

Dan Johnson
40,533 PointsTry these fixes out:
Line 17
//system -> System
System.out.printf("Congratulations, you won with %d tries remaining. The answer was %s.", mGame.getRemainingTries(), mGame.getAnswer());
Line 19
// print -> printf
System.out.printf("Bummer, the word was %s.", mGame.getAnswer());
Eric Conklin
8,350 PointsEric Conklin
8,350 PointsWorked. Thanks!