Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial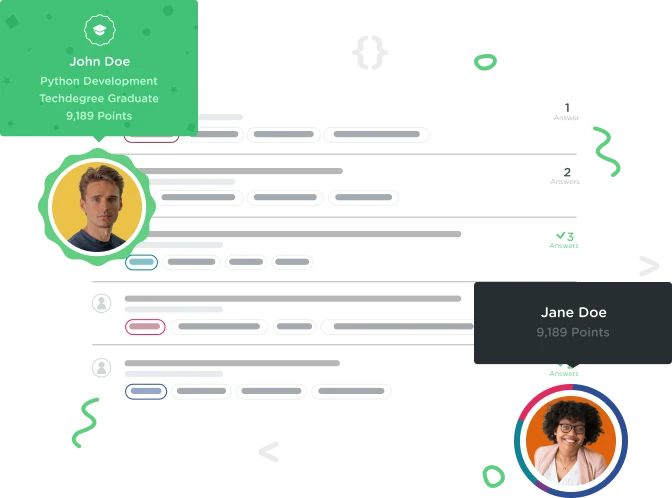
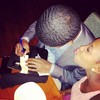
Leviticus Rolle Jr
3,036 PointsCannot complete Object-Oriented Swift code challenge
The Challenge Task says, Assume you are creating a Weather app and are provided the temperature in Celsius. We need to create a computed property called Fahrenheit which will return a computed value. (Note: Fahrenheit = (Celsius * 1.8) + 32)
Here is my code. In Xcode I get no errors, is there something I'm missing?
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
return (celsius * 1.8) + 32
}
init(celsius: Float) {
self.celsius = celsius
}
}
let celsiusConverstionTo = Temperature(celsius: 37.0)
celsiusConversionTo.fahrenheit
The Code Challenge after that
We need to add getter and setter methods to the fahrenheit property of the Temperature class. Add a getter method to the access the value of the fahrenheit property. Then add a setter method. When the user assigns a value to the fahrenheit property, the setter method will calculate and assign a value to the celsius property. (Note: Celsius = (Fahrenheit-32)/1.8))
Here's my code:
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
get{
return (celsius * 1.8) + 32
}
set {
celsius = (fahrenheit-32)/1.8
}
}
}
Which gives the error:
Bummer! The setter method for fahrenheit
did not assign the right value to the celsius
property. (Note: Celsius = (Fahrenheit-32)/1.8)
6 Answers

Chris Shaw
26,676 PointsHi Leviticus,
This task has caught a few people off guard mainly because of the random fahrenheit
occurrence which doesn't actually belong there, if you remember back in the previous video Amit shows us a variable that's scoped to the set
method on the computed variable called newValue
.
Instead of fahrenheit
we want to use newValue
instead.
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
get{
return (celsius * 1.8) + 32
}
set {
celsius = (newValue - 32) / 1.8
}
}
}
Happy coding!

Srinivasan Senthil
2,266 PointsWe have to use the newValue. The newValue holds the value of fahrenheit now. i.e. Once the get method is completed executing.
After the get method is completed, the code moves to the set method. Under the set method, it needs the value of fahrenheit to be subtracted from 32. The variable that holds the fahrenheit is newValue.
Consider the get method as computed property under video Transcript.
Read these lines on getter and setter methods. "The other thing you'll wanna note is that this is a read only property, 1:15 which means that we cannot assign a value here. 1:18 There is no underlying storage mechanism to store this value.
This is my understanding.
So the only thing that has the Fahrenheit value is newValue.

Ahmed Al-Alawi
Front End Web Development Techdegree Student 17,165 Pointsvery good explination Srinivasan :) Well Done!

Yuda Leh
7,618 PointsWe haven't done the set method, so....
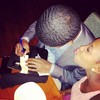
Leviticus Rolle Jr
3,036 PointsGahh.. Good Man! Thanks Chris, you don't happen to have the solution to the first Challenge as well do you?

Chris Shaw
26,676 PointsThe first challenge is the same as above with the minor difference that get
and set
aren't defined yet.
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
return (celsius * 1.8) + 32
}
}
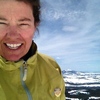
Susanna Remec
12,316 PointsHi, I had the same error. The keyword newValue was the answer that worked for me.

rd. ln.
7,851 Pointsgod. i keep getting
There was a communication problem.
If you continue experiencing problems, please contact support at help@teamtreehouse.com.
this is SO incredibly frustrating.
Walter Stevenson
Courses Plus Student 7,954 PointsWalter Stevenson
Courses Plus Student 7,954 PointsHow does the program know the newValue will be the fahrenheit?
I had code like this
Can you explain why my code wouldn't work (i guess the exercise is checking for only that answer) and how the 'newValue' knows that it belongs to fahrenheit?