Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial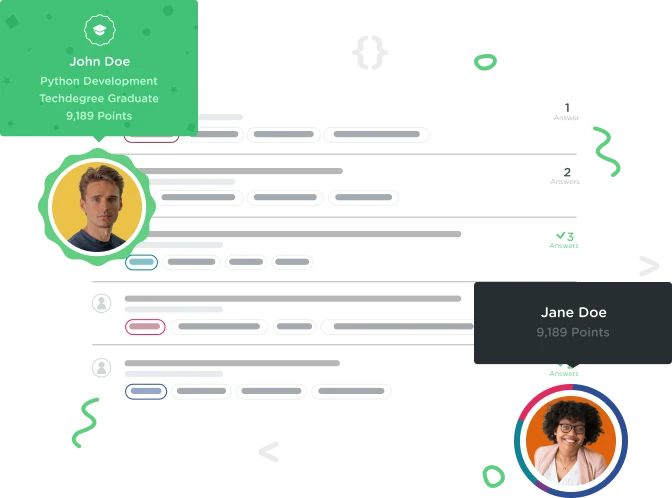

William J. Terrell
17,403 PointsCannot connect to the Database
In the last video, my code connected to the database just fine, but is now throwing the first exception ("Could not connect to the database").
My code is as follows:
<?php
try {
$db = new PDO("mysql:host=localhost;
dbname=shirts4mike;
port=3306;",
"root",
"");
$db -> setAttribute( PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION );
$db -> exec("SET NAMES 'utf-8'");
} catch (Exception $e) {
echo "Could not connect to the database.";
exit;
}
try {
$results = $db -> query("SELECT name, price FROM products");
echo "Query was successful.";
} catch ( Exception $e ) {
echo "Query could not be processed.";
exit;
}
When I remove this code:
$db -> setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
$db -> exec("SET NAMES 'utf-8'");
everything seems to work fine, but I cannot see what I am doing wrong with that bit of code.
Any assistance would be appreciated.
Thanks!
1 Answer
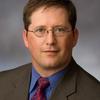
Ted Sumner
Courses Plus Student 17,967 PointsHere is my code from that project. It worked:
<?php
$db->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
$db->exec("SET NAMES 'utf8'");
The only difference I see is the white space. Try removing the spaces on both sides of the -> first. If that does not work, then remove the white space around the rest of the stuff.
Let me know what the answer is because I am very curious.
William J. Terrell
17,403 PointsWilliam J. Terrell
17,403 PointsI tried removing the white space from around the arrows, but that still didn't work, yet it works just fine when I paste in the code that you provided.
Aha! In my code, I have it written as "utf-8", whereas in your code, it is "utf8" (without the hyphen), so it must be catching an error in the database itself, and since we have that ERRMODE thing set up, it throws the Exception in that "catch" block right after it (at least I think that's how that ERRMODE thing works). :)
Thanks!
Ted Sumner
Courses Plus Student 17,967 PointsTed Sumner
Courses Plus Student 17,967 PointsI should have seen the hyphen. I read that we should really use utf8mb4. I am not sure why that is, though. I think it is a security issue.