Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial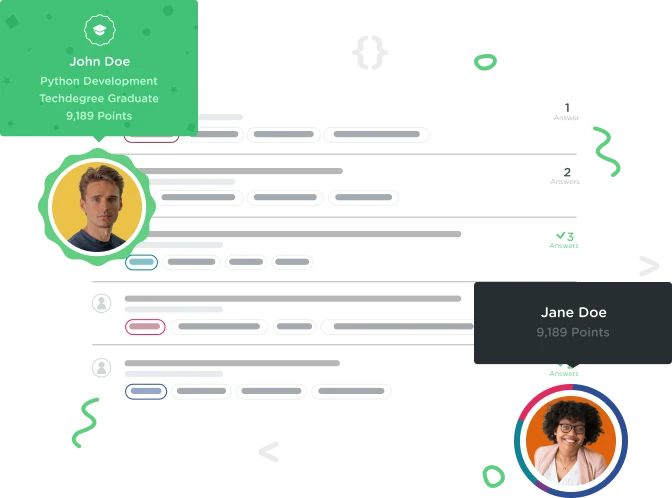

ROLAND KIRALy
Front End Web Development Techdegree Student 35 PointsCannot declare array inside the function
Hello all, this is my code:
var arr = [];
/*Note: We've supplied you a basic function for generating a random number from 1 to 100 */
function random100() {
return Math.floor(Math.random() * 100) + 1;
}
/* 1. Create a function named createRandomList that uses a for loop to create an array containing 10 random numbers from 1 to 100 (use the supplied function above to generate the numbers). The function should return that array. */
function createRandomList() {
for (i = 0; i < 10; i++) {
arr[i] = (random100());
}
}
/* 2. Call the createRandomList() function and store the results in a variable named myRandomList. */
createRandomList();
var myRandomList = arr.join(',');
console.log(myRandomList);
/* 3. Use a for loop to access each element in the loop. Each time through the loop log a message to the console that looks something like this:
Item 0 in the array is 48
When you're done you should have output 10 lines to the console -- one for each element.
*/
for (i = 0; i < 10; i++) {
console.log("Item " + i + " in the array is " + arr[i]);
}
This is a working code, but the instruction asks me to declare the array in the createRandomList()
function. If I move my var arr = []
inside the function, the program won't run.
2 Answers
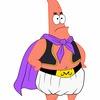
<noob />
17,062 PointsYou have few mistakes. You need to declare the arr before the function starts. and use let to declare the i variable inside the for loop. after that u need in each iteration to add a random number.
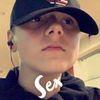
Keegan Swanson
1,339 Pointsthis doesn't make sense. Please help me!