Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial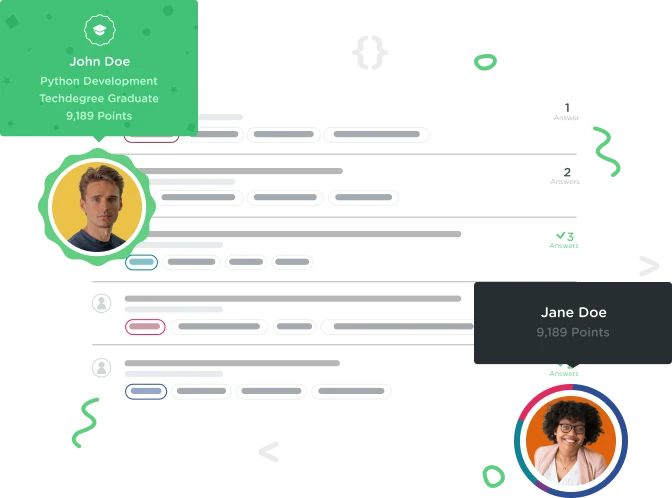

sohtainoguchi
817 PointsCannot figure out solution
As in a title, cannot figure out solution, please help...
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
private int count;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter () {
int count = 0;
for (char letter : tiles.toCharArray()) {
count++;
}
return count;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
2 Answers

Isaiah Duncan
3,241 PointsSo I can see two critical issues you have here:
1) In your main method, you are taking a character as an argument for your getCountOfLetter method. However, in your method's signature, you are not allowing for any arguments for this method. This method's signature should be changed to allow a character as an argument and would look something like this:
// this is what your method's signature should look like
public int getCountOfLetter(char l)
//this is what you have
public int getCountOfLetter()
2) The second critical issue is how you are determining the count. In your current implementation, you are having your count variable increment by 1 for each element in your array of characters. This won't give you the desired functionality of counting specific letter occurrences each player has.
Instead, you would want to set a condition that checks if the character entered as an argument is in the player's pool of letters. If so, then we increase our count by 1 because we've found an instance of the letter we are searching for. So the code would look something like:
//this is what the code should look like
public int getCountOfLetter (char l) {
int count = 0;
for (char letter : tiles.toCharArray()) {
if(l == letter){
count++;
}
}
return count;
}
//this is what you have
public int getCountOfLetter () {
int count = 0;
for (char letter : tiles.toCharArray()) {
count++;
}
return count;
}

sohtainoguchi
817 PointsThank you very much for your help!

Isaiah Duncan
3,241 PointsYou're welcome. Happy coding! :)