Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial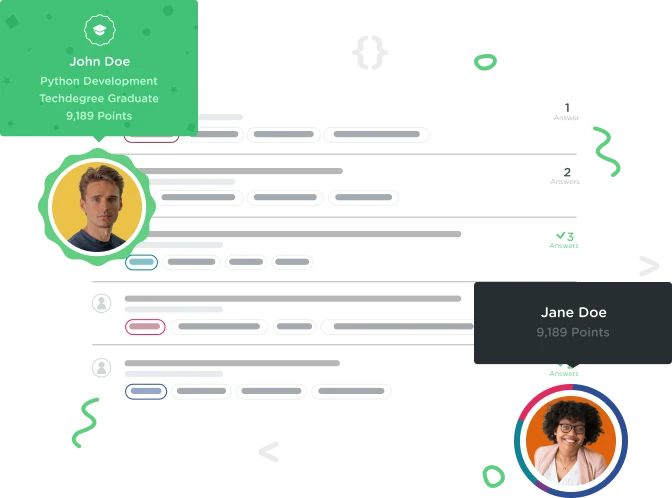

Christopher Morris
iOS Development with Swift Techdegree Student 1,760 PointsCannot figure out why my elif are not working..
name = input("Please enter your name: ") number = int(input("Please enter a number: "))
TODO: Make sure the number is an integer*
TODO: Print out the User's name and the number entered,
making sure the two statements are on separate lines of output.
TODO: Compare the number the user gave with the different
FizzBuzz conditions.
*********************
If the number is divisible by 3, print "is a Fizz number."
If the number is divisible by 5, print "is a Buzz number."
If the number is divisible by both 3 and 5, print "is a FizzBuzz number."
Otherwise, print "is neither a fizzy or a buzzy number."
*********************
print(name,"\n", number) if (number / 3): print("{} is a Fizz number.".format(number)) elif (number / 5): print("{} is a Buzz number.".format(number)) elif (number / 3 & number / 5): print("{} is a FizzBuzz number.".format(number)) elif (number/3 or number/5): print("{} is neither a fizzy or a buzzy number.".format(number))
TODO: Define variables for is_fizz and is_buzz that stores
a Boolean value of the condition. Remember that the modulo operator, %,
can be used to check if there is a remainder.
Using the variables, check the condition of the value, and print the necessary
string
I tried using % and tried removing the parenthesis but no luck! I need help :(
1 Answer

KRIS NIKOLAISEN
54,969 PointsYou'll want to use the modulo operator. In your code if (number / 3) is always true (except if number = 0 ) so the rest of the conditions are never considered.
One solution:
name = input("Please enter your name: ")
number = int(input("Please enter a number: "))
print(name,"\n", number)
if (number % 3 == 0):
if (number % 5 == 0):
print("{} is a FizzBuzz number.".format(number))
else:
print("{} is a Fizz number.".format(number))
elif (number % 5 == 0):
print("{} is a Buzz number.".format(number))
else:
print("{} is neither a fizzy or a buzzy number.".format(number))