Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial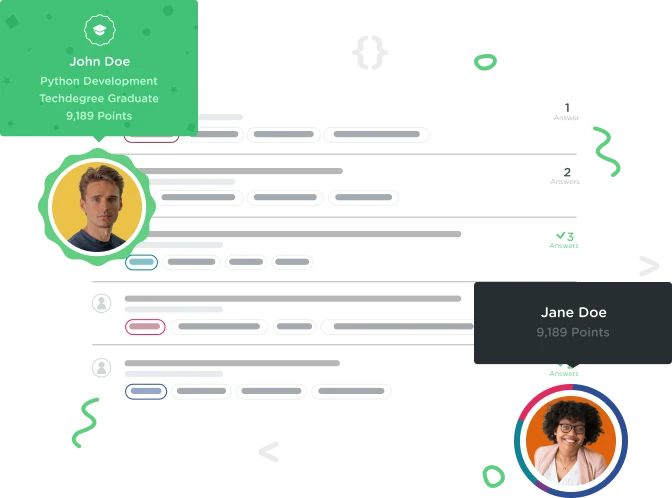

Aritro Banerjee
Courses Plus Student 2,567 PointsCannot find log statement.Also made a toast to display timezone.(Didnt work)
So I am unable to see the log entry even though I debugged it and it showed me that timezone variable does have the value in it. I then made a toast to display the timezone and it crashed my app.
package com.example.aritrobanerjee93.stormy;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.nfc.Tag;
import android.nfc.TagLostException;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.widget.Toast;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class MainActivity extends AppCompatActivity {
private static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather mCurrentWeather;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey="0f6f530003b63e31a5c9f11669711b8c";
double latitude=37.8267;
double longitude=-122.4233;
String forecastUrl="https://api.darksky.net/forecast/"+apiKey+"/" +latitude+","+longitude;
if(isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl).
build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
String jsonData=response.body().string();
//Response response =call.execute(); This is the synchronous call method!
Log.v(TAG,jsonData );
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
} else {
alertUserAboutError();
}
}
catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
catch(JSONException e){
Log.e(TAG, "Exception caught: ", e);
}
}
});
}
else{
Toast.makeText(this, R.string.network_unavailable_message,Toast.LENGTH_LONG).show();
}
Log.d(TAG,"Main UI code is running!");
}
private CurrentWeather getCurrentDetails(String jsonData)throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG,"From JSON: "+ timezone);
Toast.makeText(this,"Timezone is :" + timezone,Toast.LENGTH_LONG).show();
return new CurrentWeather();
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if(networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(),"error_dialogue");
}
}