Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial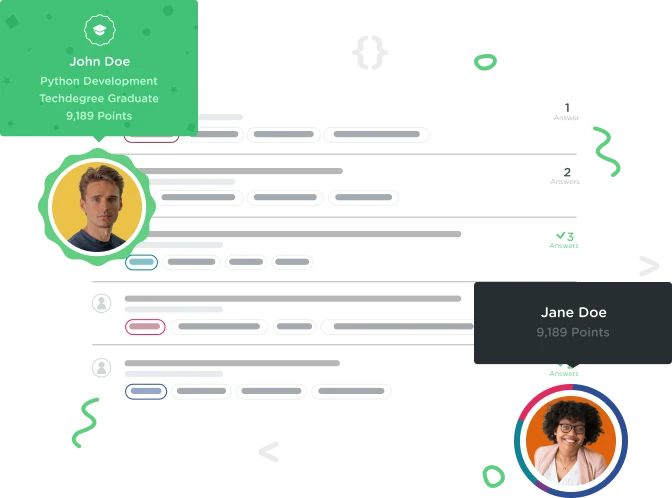

Steven Thomas
2,911 PointsCannot find symbol error at compile of PrinterTest.Java - forEach Java's Functional Toolset
When I attempt to run the unit tests with junit I am getting a compile error. I have made no changes to the test class and it seems to be an issue with this line:
inspector = sourcery.inspectorFor(Printer.class);
The code for the test class is as follows:
package com.teamtreehouse.challenges;
import com.teamtreehouse.ast.Inspector;
import com.teamtreehouse.ast.predicates.Expressions;
import com.teamtreehouse.ast.predicates.Methods;
import com.teamtreehouse.ast.rules.Sourcery;
import com.teamtreehouse.teachingassistant.rules.Consoler;
import org.junit.Before;
import org.junit.ClassRule;
import org.junit.Rule;
import org.junit.Test;
import java.util.Arrays;
import static org.junit.Assert.assertTrue;
public class PrinterTest {
public static final String METHOD_NAME = "printChipmunksDeclaratively";
private Inspector inspector;
@ClassRule
public static Sourcery sourcery = new Sourcery();
@Rule
public Consoler consoler = new Consoler();
@Before
public void setUp() throws Exception {
inspector = sourcery.inspectorFor(Printer.class);
}
@Test
public void declarativeMethodExists() throws Exception {
String msg = String.format("Whoops! Looks like you accidentally removed the '%s' method",
METHOD_NAME);
assertTrue(msg, inspector.hasMethodNamed(METHOD_NAME));
}
@Test
public void declarativelyUsesForEach() throws Exception {
String msg = String.format("Ensure you use forEach in the more declarative '%s' method",
METHOD_NAME);
assertTrue(msg, inspector.matchingChain(chain -> chain
.withMethod(Methods.named(METHOD_NAME))
.withExpression(Expressions.isMethodCall().and(
Expressions.containing("forEach")))));
}
@Test
public void printsProperly() throws Exception {
Printer.printChipmunksDeclaratively(Arrays.asList("Example1", "Example2"));
Printer.printChipmunksDeclaratively(Arrays.asList("Example3"));
consoler.addExpectedPrompt("Example1!");
consoler.addExpectedPrompt("Example2!");
consoler.addExpectedPrompt("Example3!");
String msg = String.format("The output from your method '%s' seems wrong", METHOD_NAME);
consoler.assertExpected(msg);
}
}

Steven Thomas
2,911 PointsMy first thought was the same thing. I tried adding the import but that did not work or change anything. In fact my IDE indicates that it is an unused import statement when I do so. In any case, here is the full set of errors.
Also the track for this is introduction to functional programming in the Java courses. Specifically using forEach
Error:(31, 39) java: cannot find symbol symbol: class Printer location: class com.teamtreehouse.challenges.PrinterTest Error:(53, 5) java: cannot find symbol symbol: variable Printer location: class com.teamtreehouse.challenges.PrinterTest Error:(54, 5) java: cannot find symbol symbol: variable Printer location: class com.teamtreehouse.challenges.PrinterTest

Kamren Kowa
2,958 PointsSteven, did you ever figure this out? I'm having the same issue a year and a half later...

Steven Thomas
2,911 PointsRe posting this to the correct place. Unfortunately I was not able to sort it out. I ended up just moving on and skipping the "points" for it because I was reasonably sure the code I wrote was acceptable.
More frustratingly I just opened up the project sitting on my device and ran the test class without issue.
1 Answer

Kamren Kowa
2,958 PointsActually, I think I just got it! Maybe a quirk between versions of IntelliJ, but...
When importing the project, the default import options contain:
- [ ] Use Auto Import (default for me: OFF)
- [ ] Create directories for empty content roots automatically (default for me: OFF)
- [ ] Create separate module per set (default for me: ON)
When i imported the file with ON, ON, OFF above, I no longer encountered the issue. I know it's not a very technical solution, but trial and error won out! Testing multiple configurations pointed to "Create separate module per set" being the root of the issue; with that disabled, the issue is no longer present.
Now you can go back and get those point if you still want them ha
Brandon Khan
21,619 PointsBrandon Khan
21,619 PointsWhat does the error say exactly? My first guess is the Printer class needs an import or If its trying to inspect this class maybe PrinterTest as its the name of the class. I couldn't find what track this is in so I'm just shooting from the hip lol