Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial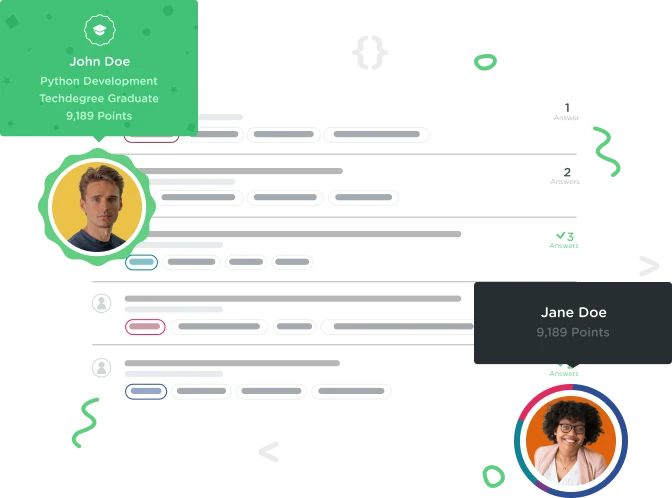

Daniel Springer
7,600 PointsCannot find symbol error when creating KaraokeMachine object
Hey everyone, i was following the lesson and i managed to make it all the way to the end of the video and i felt like i was understanding everything fairly well until i ran into an error when in my testing class i attempted to create a new Karaoke Machine and fill it with the parameter i just called songBook, it tells me in the ide im using that it cannot find symbol songbook. any insight on this would be appreciated everyone thanks! btw ive got two classes setup below, my testing class karaoke and my karaokemachine class which holds all the logic for each karoakemachine object.
package karaoke;
import java.util.ArrayList; import java.util.List; import java.util.Set; import java.util.TreeSet;
public class Karaoke {
public static void main(String[] args)
{
KaraokeMachine machine=new KaraokeMachine(songBook);
machine.run();
}
}
package karaoke;
import java.io.BufferedReader; import java.io.InputStreamReader; import java.io.IOException; import java.util.HashMap; import java.util.Map; import java.util.Scanner;
public class KaraokeMachine { private SongBook dSongBook; private Map<String,String>dMenu; Scanner input=new Scanner(System.in);
public KaraokeMachine(SongBook songBook)
{
this.dSongBook=songBook;
dMenu=new HashMap<String,String>();
dMenu.put("add", "add a new song to the song book");
dMenu.put("quit", "EXIT THE PROGRAM");
}
private String promptAction() throws IOException //possibly create an input output problem
{
System.out.printf("there are %d songs available. "
+ "Your options are \n",dSongBook.getSongCount());
//for each menu option inside dMenu
for(Map.Entry<String,String> menuOption : dMenu.entrySet())
{
System.out.println(menuOption.getKey()+" "+ menuOption.getValue());
}
System.out.print("What would you like to do?");
String choice=input.nextLine();
//for(choice != dMenu.){System.out.print("not a valid command");}
return choice.trim().toLowerCase(); //trim removes space at beggining and end of string
}
public void run()
{
String choice = null;
do{
try
{
choice=promptAction();
switch(choice)
{
case "add": //if choice is equal to add, add a new song
Song song=promptNewSong();
dSongBook.addSong(song);
System.out.println("adding "+song);
break; //ends the block of code running for case add
case "quit": System.out.print("exiting program");
break;
//if value is not equal to any of the cases
default: System.out.printf("Unknown Choice, try again \n \n"+ choice);
}
}
catch(IOException ioe){System.out.print("Problem with input");
ioe.printStackTrace();
}
}
while(!choice.equals("quit"));
}
private Song promptNewSong()
{
System.out.println("Enter the artists name");
String artist=input.nextLine();
artist.trim().toLowerCase();
System.out.print("Enter the title: ");
String title=input.nextLine();
title.trim().toLowerCase();
System.out.print("Enter video link");
String videoLink=input.nextLine();
videoLink.trim().toLowerCase();
return new Song(artist,title,videoLink);
}
}
2 Answers

Benjamin Barslev Nielsen
18,958 PointsKaraokeMachine machine=new KaraokeMachine(songBook);
Where does the variable songBook come from? Looking at your code I can't find an instantiation of SongBook called songBook, so that might be why your IDE is complaining. Try:
KaraokeMachine machine=new KaraokeMachine(new SongBook());

Daniel Springer
7,600 Pointswell thank you, that solved everything, new it was a simple solution too lol. just couldnt wrap my head around it.