Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial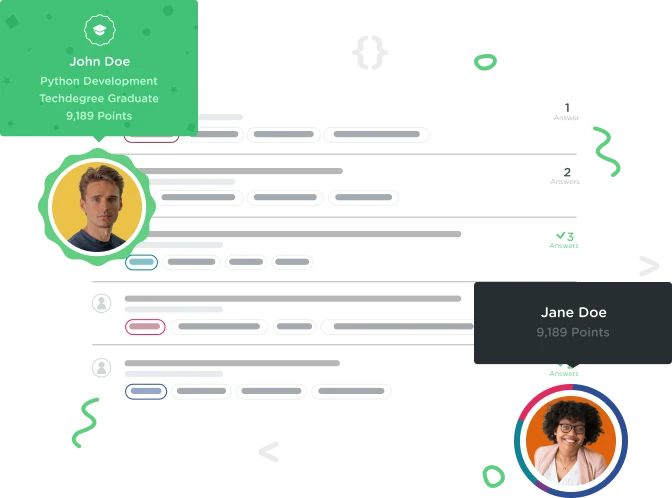

Shane Conroy
Courses Plus Student 11,032 PointsCannot Find Symbol Errors:
This is likely a missing bracket somewhere, but i can't find it for the life of me. I'm getting the following errors when i try to compile after modifying the "DocProcessor.java" file:
treehouse:~/workspace$ javac -d out -cp src src/com/teamtreehouse/docgen/Main.java
Picked up JAVA_TOOL_OPTIONS: -Xmx128m
Picked up _JAVA_OPTIONS: -Xmx128m
src/com/teamtreehouse/docgen/DocProcessor.java:99: error: cannot find symbol
int actualParamCount = method.getParamCount();
^
symbol: method getParamCount()
location: variable method of type Method
src/com/teamtreehouse/docgen/DocProcessor.java:113: error: cannot find symbol
return method.getRetunType.equals(Void.TYPE) || !doc.retunVal().isEmpty();
^
symbol: variable getRetunType
location: variable method of type Method
src/com/teamtreehouse/docgen/DocProcessor.java:113: error: cannot find symbol
return method.getRetunType.equals(Void.TYPE) || !doc.retunVal().isEmpty();
^
symbol: method retunVal()
location: variable doc of type Doc
3 errors
Here's my DocProcessor class:
package com.teamtreehouse.docgen;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
public class DocProcessor {
/**
* Analyzes the given class's Doc annotation, displaying output
* for the class and for each of its non-private methods.
* @param clazz Class to analyze
* @return True if Doc annotation is used sufficiently on the class
* and its methods, false otherwise
*/
public static boolean process(Class<?> clazz) {
// Store simple name of the class for quicker access
String className = clazz.getSimpleName();
// Display class name
System.out.printf("Analyzing '%s'...",className);
// Track the number of class errors
int classErrors = 0;
if (clazz.isAnnotationPresent(Doc.class)) {
for(Method method : clazz.getDeclaredMethods()) {
int modifierInt = method.getModifiers();
String methodName = method.getName();
if(!Modifier.isPrivate(modifierInt)) {
int methodErrors = 0;
// Display method name
System.out.printf("%n%n\t%s:", methodName);
if (method.isAnnotationPresent(Doc.class)) {
Doc doc = method.getAnnotation(Doc.class);
// Does the number of items in param descriptions match
// the number of actual parameters?
int numMissing = getNumMissingParams(method, doc);
if (numMissing > 0) {
methodErrors++;
String message = "%n\t\t=> Missing %s parameter description(s)";
System.out.printf(message, numMissing);
}
// Is there a return description when needed?
if(!hasReturnDescription(method, doc)) {
methodErrors++;
String message = "%n\t\t=> Missing description of return value";
System.out.printf(message);
}
} else { // non-private method is missing the Doc annotation
methodErrors++;
System.out.printf("%n\t\t=> Doc annotation missing");
}
// Check for zero errors
if (methodErrors == 0) {
System.out.printf("%n\t\t=> No changes needed");
}
// Add method errors to class errors
classErrors += methodErrors;
}
}
} else { // class is missing the annotation
classErrors++;
System.out.printf("%n\t=> Class does not contain the proper documentation");
}
// Display final message
String yayOrNay = classErrors == 0 ? "YAY" : "Get to documenting";
String finalMessage = "%n%nDocProcessor has found %s error(s) in class '%s'. %s!%n";
System.out.printf(finalMessage, classErrors, className, yayOrNay);
// Return success or failure
return classErrors == 0;
}
/**
* Checks whether or not the number of descriptions provided in the Doc annotation
* match the number of parameters in the given method.
* @param method Method under consideration
* @param doc Annotation to check
* @return Number of descriptions missing.
* Note: This could be negative if too many descriptions are provided)
*/
private static int getNumMissingParams(Method method, Doc doc) {
int numMissing = 0;
int annotatedParamCount = doc.params().length;
int actualParamCount = method.getParamCount();
if (annotatedParamCount < actualParamCount) {
numMissing = actualParamCount - annotatedParamCount;
}
return numMissing;
}
/**
* Determines whether or not a method's return value description is missing
* @param method Method under consideration
* @param doc Annotation to check
* @return True if method has a void return type or the annotation has a non-empty return description
*/
private static boolean hasReturnDescription(Method method, Doc doc) {
return method.getRetunType.equals(Void.TYPE) || !doc.retunVal().isEmpty();
}
}
2 Answers

Seth Kroger
56,413 PointsFor the first error, there's no method called getParamCount() on the Method class. It should be getParameterCount(). On the next two errors, you misspelled "Return" in the method names.

Shane Conroy
Courses Plus Student 11,032 PointsThanks for taking the time. I knew it was something simple. I'll be sure to check the actual method names next time. I skimmed over those completely for some reason. I was focusing on the variable declaration, which was fine.