Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial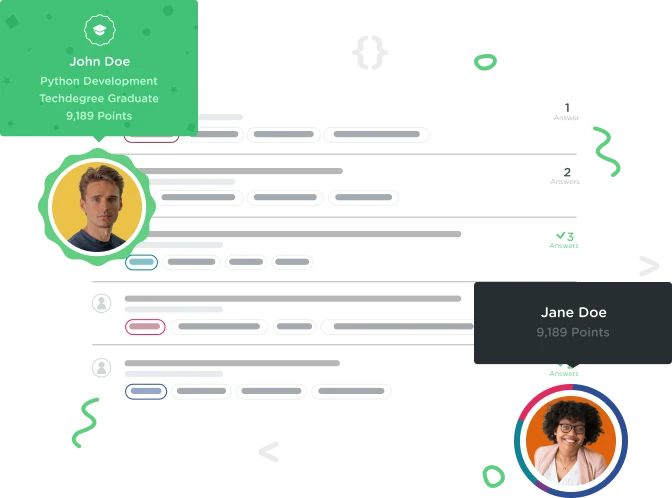

Junyoung Sim
471 Points"Cannot find symbol": I can't solve an error
I wanted to practice what I learned from the course by duplicating what was done with the "PezDispenser" example. I did exactly the same:
Under "Example.java" file, I coded as follows:
public class Example {
public static void main(String[] args) { // Your amazing code goes here... System.out.println("We are making a new PEZ Dispenser"); PezDispenser dispenser = new PezDispenser("Yoda"); System.out.printf("The dispenser is %s %n", dispenser.getCharacterName() );
System.out.println("2019 has come:");
NewYearResolution resolution = new NewYearResolution("coding every day");
System.out.printf("One of my new year resolutions is %s %n",
resolution.getNewResolution()
);
}
}
Under "NewYearResolution.java" file, I coded as follows:
class NewYearResolution { final private String newResolution;
public NewYearResolution(newResolution) { this.newResolution = newResolution; }
public String getNewResolution; { return newResolution; }
}
However, I am getting the following errors:
./NewYearResolution.java:4: error: <identifier> expected
public NewYearResolution(newResolution) {
^
./NewYearResolution.java:5: error: ';' expected
this.newResolution = newResolution
^
./NewYearResolution.java:4: error: cannot find symbol
public NewYearResolution(newResolution) {
^
symbol: class newResolution
location: class NewYearResolution
Example.java:14: error: cannot find symbol
resolution.getNewResolution()
^
symbol: method getNewResolution()
location: variable resolution of type NewYearResolution
./NewYearResolution.java:9: error: return outside method
return newResolution;
^
5 errors
Can you please tell me what I have missed?
1 Answer

Unsubscribed User
1,321 PointsI have left comments in the code below to help.
Example.java
public class Example {
public static void main(String[] args) {
// Comment out all the old PezDispenser code by adding /* and */ so it doesn't interfere with your code or DELETE it altogether.
/*
System.out.println("We are making a new PEZ Dispenser");
PezDispenser dispenser = new PezDispenser("Yoda");
System.out.printf("The dispenser is %s %n", dispenser.getCharacterName() );
*/
System.out.println("2019 has come:");
NewYearResolution resolution = new NewYearResolution("coding every day!");
System.out.printf("One of my new year resolutions is to %s %n",
resolution.getNewResolution());
}
}
NewYearResolution.java
class NewYearResolution {
final private String newResolution;
public NewYearResolution(newResolution) { // You did not define newResolution as a string.
this.newResolution = newResolution;
}
public String getNewResolution; { // You have a semicolon here which should be removed.
return newResolution;
}
}
FIXED CODE
----------------
Example.java
public class Example {
public static void main(String[] args) {
System.out.println("2019 has come:");
NewYearResolution resolution = new NewYearResolution("code every day!");
System.out.printf("One of my new year resolutions is to %s %n",
resolution.getNewResolution());
}
}
NewYearResolution.java
class NewYearResolution {
final private String newResolution;
public NewYearResolution(String newResolution) {
this.newResolution = newResolution;
}
public String getNewResolution() {
return newResolution;
}
}

Unsubscribed User
1,321 PointsYou're on track just slow down and make sure you are following the syntax.
Reach out to me and I would be more than happy to help as much as I can.
Unsubscribed User
1,321 PointsUnsubscribed User
1,321 PointsJust wanted to format your text so I could read it a little better.
Example.java
NewYearResolution.java