Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial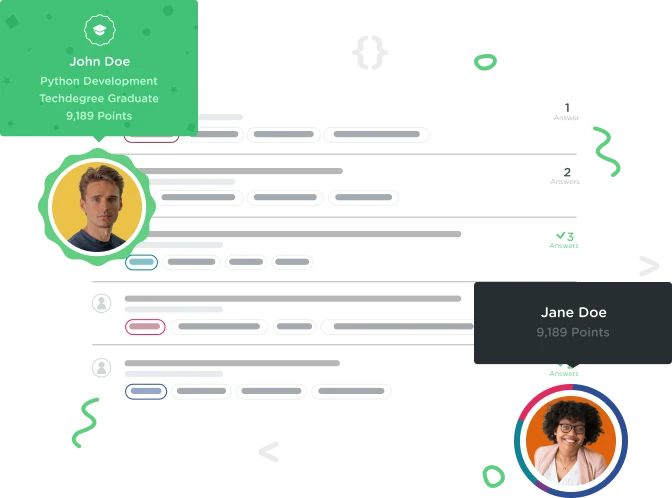

Ivan Cox
11,216 PointsCannot find the Issue with my code
i am trying to follow the movement tutorial of the dungeon game, but when i input the movement commands it does not update the coordinates of the player.below is my Code
import random
import os
#draw a grid
CELLS = [(0,0), (1,0), (2,0), (3,0), (4,0),
(0,1), (1,1), (2,1), (3,1), (4,1),
(0,2), (1,2), (2,2), (3,2), (4,2),
(0,3), (1,3), (2,3), (3,3), (4,3),
(0,4), (1,4), (2,4), (3,4), (4,4),
]
def move_player(player, move):
x, y = player
if move == "Left":
x -= 1
if move == "Right":
x += 1
if move == "Up":
y -= 1
if move == "Down":
y += 1
return x, y
def get_moves(player):
moves = ["Left","Right","Up","Down"]
x,y = player
if x == 0:
moves.remove("Left")
if x == 4:
moves.remove("Right")
if y == 0:
moves.remove("Up")
if y == 4:
moves.remove("Down")
return moves
#clear screen
def clear_screen():
os.system('cls' if os.name == 'nt' else 'clear')
def get_location():
return random.sample(CELLS, 3)
#pick random location for the player
#pick random location for the exit door
#pick random location for the monster
player, monster, door = get_location()
while True:
valid_moves = get_moves(player)
clear_screen()
print("Welcome to the dungeon!!")
print("You are currently at the position {}".format(player))
print("You can make the following moves {}".format(", ".join(valid_moves)))
print("Enter Quit to exit the game")
move = input(">> ").lower()
if move == 'quit':
break
if move in valid_moves:
player = move_player(player, move)
else:
print("\n Cannot go through the wall\n")
continue
1 Answer

andren
28,558 PointsYour valid moves list are written with the first letter capitalized, but you convert all input to lower case. Therefore you will always end up doing an invalid move. If you change your input line to this:
move = input(">> ").capitalize()
Then it should work. The capitalize
method return a string that only has it's first letter capitalized, which matches the naming convention you have used on the valid moves list. Though note that you will then also have to rename quit
to Quit
in order for the exit command to still work.
Alternatively you could also just rename the valid moves and write them all in lower case and then leave your input and exit code as is.
Ivan Cox
11,216 PointsIvan Cox
11,216 PointsThank you very much. that did it