Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial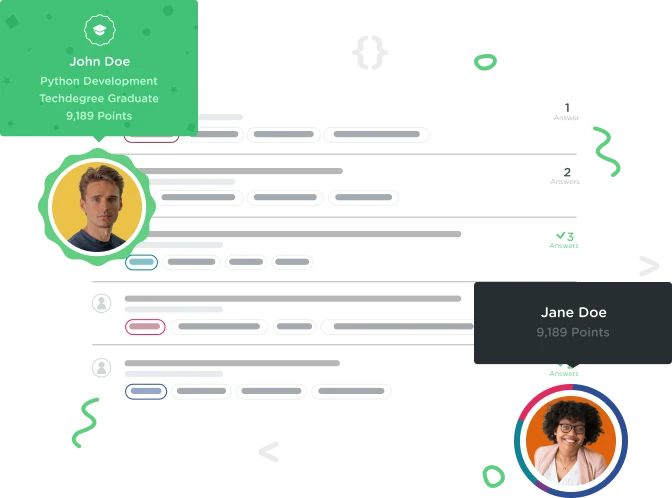

nick fonseca
2,331 PointsCannot get a pass for Python Collections Quiz 2/2
I'm trying to get to pass the Python Collections 2/2. I have entered my code, and other examples but it doesn't seem to pass. Can someone please help?
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(param):
returnable = []
for course in COURSES:
if COURSES[course] & param:
returnable.append(course)
return returnable
def covers_all(set_1):
returnable = []
for course in COURSES:
for parameters in course:
if course[set_1] & course:
returnable.append(course)
return returnable
2 Answers
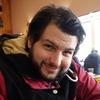
Eric M
11,545 PointsHi Nick,
A course
in COURSES
is a key, value pair so your parameters loop probably isn't doing what you intended.
The &
set operator returns an intersection of the two sets either side of it. When used in an if
statement this will evaluate to True
if anything is returned at all. As we only want to return the names of sets that have all of the items of our subset we actually want to test issubset()
which can be done using the <=
operator.
To loop through a dictionary in python use the dictionaries items()
iterator method.
e.g.
def covers_all(set_1):
returnable = []
for key, value in COURSES.items():
if set_1 <= value:
returnable.append(key)
return returnable
Cheers,
Eric

eestsaid
1,311 PointsGreat resource - thanks Eric.
nick fonseca
2,331 Pointsnick fonseca
2,331 PointsEric McKibbin Thanks! I see what you mean. Your solution got me what I needed.
eestsaid
1,311 Pointseestsaid
1,311 PointsEric - I came upon your response after struggling with this challenge. I have a question for you. I'm not sure what if set_1 <= value: is doing. Is it saying that if set one, taken in full, is less than all the items in value then evaluate to true? I didn't realise you could compare sets in full like that ... pretty cool.
Eric M
11,545 PointsEric M
11,545 PointsHi Eestsaid,
Python has some very strong builtin support for sets including the use of operators to compare sets. I recommend checking out the offical docs [https://docs.python.org/3.7/library/stdtypes.html#set-types-set-frozenset](here) to see what the different operators do.
As you'll see in the docs
set <= other_set
tests whether every element in the set is in other_set. This can also be accomplished with theissubset
method e.g.set.issubset(other_set)
.Cheers,
Eric