Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial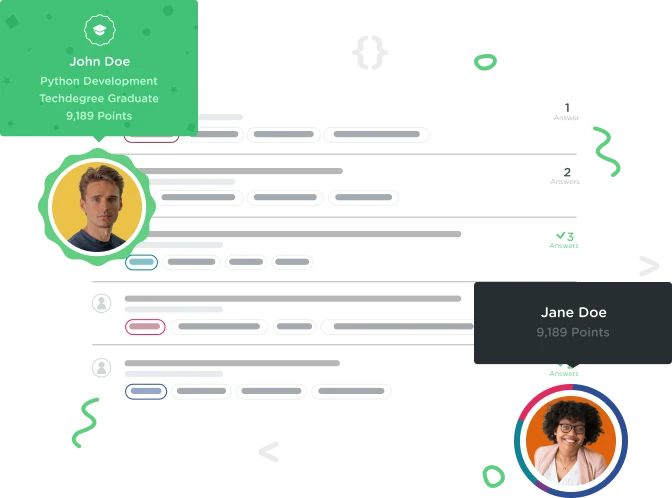

Ermin Bicakcic
5,144 Pointscannot get this to work with else statement
So I cannot get this code to work once I add else statement (In case that username is not in database) why is that?
let message = '';
let student;
function print(message) {
let outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
while (true) {
search = prompt("Search for a student in our database and to quit type in 'quit' to exit").toLowerCase();
if ( search === 'quit') {
break;
} else {
for ( let i = 0; i < students.length; i++ ) {
if ( search === students[i].name.toLowerCase() ) {
let data = students[i];
message += '<h2>Student: ' + data.name + '</h2>';
message += '<p>Track: ' + data.track + '</p>';
message += '<p>Achievement: ' + data.achievements + '</p>';
message += '<p>Points: ' + data.points + '</p>';
print(message);
} else {
print( search + ' is not in database.');
}
}
}
}
3 Answers

KRIS NIKOLAISEN
54,971 PointsThe issue is you are looping through the entire students array whether the student is found or not. So unless the last student in the array is entered, in this case Trish, the message will always be the one from the else statement. To fix you could break if a student was found.
for ( let i = 0; i < students.length; i++ ) {
if ( search === students[i].name.toLowerCase() ) {
let data = students[i];
message += '<h2>Student: ' + data.name + '</h2>';
message += '<p>Track: ' + data.track + '</p>';
message += '<p>Achievement: ' + data.achievements + '</p>';
message += '<p>Points: ' + data.points + '</p>';
print(message);
break;
} else {
print( search + ' is not in database.');
}
}

a v
Full Stack JavaScript Techdegree Student 2,302 PointsI added a break, but My program couldn't stop

Bartlomiej Pajak
5,063 PointsOr you could remove print(message) from the loop and add it after the loop like this:
let message = '';
let student;
function print(message) {
let outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
while (true) {
search = prompt("Search for a student in our database and to quit type in 'quit' to exit").toLowerCase();
if ( search === 'quit') {
break;
} else {
for ( let i = 0; i < students.length; i++ ) {
if ( search === students[i].name.toLowerCase() ) {
let data = students[i];
message += '<h2>Student: ' + data.name + '</h2>';
message += '<p>Track: ' + data.track + '</p>';
message += '<p>Achievement: ' + data.achievements + '</p>';
message += '<p>Points: ' + data.points + '</p>';
} else {
print( search + ' is not in database.');
}
}
}
}
print(message);