Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial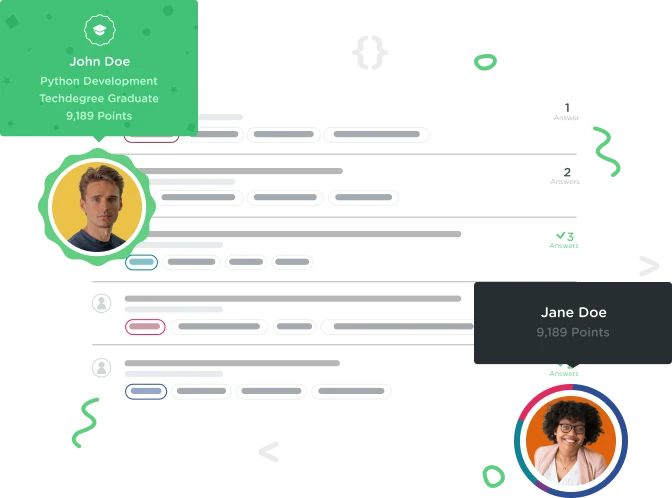

Roderick Kar
8,456 Pointscannot move to inbox of tabbar using imagePickerControllerDidCancel in Swift
i must have made mistakes trying to convert the code to swift. when running on an actual phone, i got into a situation where the camera keeps looping once i pressed "cancel". the lesson says setSelectedIndex to be applied to tabBarController but i could only find selectedIndex.
any help will be much appreciated.
thanks!
class CameraViewController: UITableViewController { var imagePicker = UIImagePickerController()
override func viewDidLoad() {
super.viewDidLoad()
}
override func viewWillAppear(animated: Bool) {
super.viewWillAppear(true)
imagePicker.allowsEditing = false
if UIImagePickerController.isSourceTypeAvailable(UIImagePickerControllerSourceType.Camera) {
imagePicker.sourceType = UIImagePickerControllerSourceType.Camera
} else {
imagePicker.sourceType = UIImagePickerControllerSourceType.PhotoLibrary
}
imagePicker.mediaTypes = UIImagePickerController.availableMediaTypesForSourceType(imagePicker.sourceType)!
self.presentViewController(imagePicker, animated: false, completion: nil)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
// MARK: - Table view data source
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return 0
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 0
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("cell", forIndexPath: indexPath) as UITableViewCell
return cell
}
// MARK: - Image Picker Controller Delegate
func imagePickerControllerDidCancel(picker: UIImagePickerController!){
self.dismissViewControllerAnimated(false, completion: nil)
self.tabBarController?.selectedIndex = 0
}
}
1 Answer

Stone Preston
42,016 Pointsits probably looping because of this line of code in viewWillAppear
self.presentViewController(imagePicker, animated: false, completion: nil)
whenever you dismiss the imagePicker, the viewWillAppear method is called, resulting in the loop. maybe try setting the selectedIndex before dismissing the view controller
func imagePickerControllerDidCancel(picker: UIImagePickerController!){
self.tabBarController!.selectedIndex = 0
self.dismissViewControllerAnimated(false, completion: nil)
}
Roderick Kar
8,456 PointsRoderick Kar
8,456 Pointshi stone,
thanks for your quick response. i think it's looping because tab index 0 cannot be called up. focus of the app is supposed to switch from the camera tab to the inbox tab when the modal camera is cancelled. i just put a println() statement inside func imagePickerControllerDidCancel to see if the function get called at all. turns out it is never called. in face, if i comment out the whole func, the app behaves the same. so i think somehow the func code i wrote is never used. i just couldn't reconcile imagePickerControllerDidCancel with the objective-c code in the lesson completely. by the way, how do you mark the comment to make the codes appear inside a black box? i tried surrounding the code with ``` but it didn't work ...
code in the lesson:
[self.tabBarController setSelectedIndex:0]; }
Stone Preston
42,016 PointsStone Preston
42,016 Pointsdid you set your view controller as the image pickers delegate in viewDidLoad?
self.imagePickerController.delegate = self
Roderick Kar
8,456 PointsRoderick Kar
8,456 Pointscannot, imagePickerController not available, the only option available after typing self is ''' self.imagePicker.delegate = self ''' and it reports an error of "Type 'CameraViewController' does not conform to protocol 'UIImagePickerControllerDelegate'. btw, CameraViewController inherits from UITableViewController.
Stone Preston
42,016 PointsStone Preston
42,016 Pointsyou need to add the UIImagePickerControllerDelegate protocol declaration to your class like so:
class CameraViewController : UITableViewController, UIImagePickerControllerDelegate
Roderick Kar
8,456 PointsRoderick Kar
8,456 Pointsthank you for pointing me the right direction! i watched the lesson again and found there's an additional delegate needed, UINavigationControllerDelegate. also, i added self.imagePicker.delegate = self instead of self.imagePickerController.delegate = self. below is the working code:
class CameraViewController: UITableViewController, UIImagePickerControllerDelegate, UINavigationControllerDelegate {
}