Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial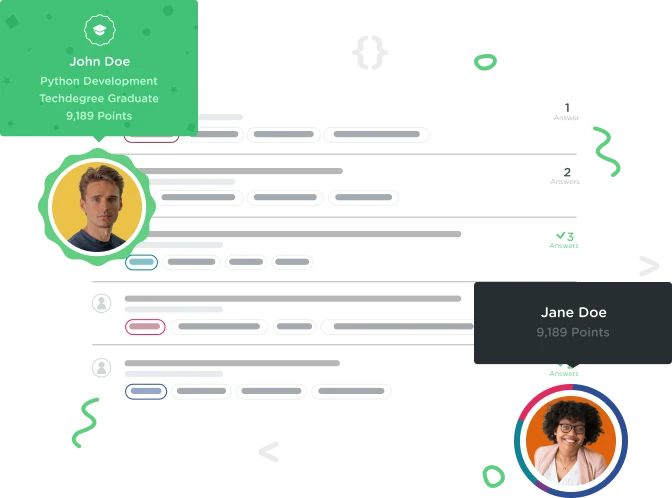

Peter Gess
16,553 PointsCannot parse the JSON in a node.js API call. I am trying to pull in the following data via the coindesk API found here:
//Goal: develop an application that retrieves the price of bitcoin and prints in to the page
//Require https
const https = require('https');
//Print bitcoin price
function printBitcoin (bpi) {
const message = `Current price of Bitcoin is ${bpi.currentprice}`
console.log(message);
};
// API Call
function get(currentPrice) {
const request = https.get(`https://api.coindesk.com/v1/bpi/currentprice/USD.json`, (res) => {
res.on('data', (d) => {
const bpi = JSON.parse(d)
printBitcoin(bpi);
});
}).on('error', (e) => {
console.error(e);
});
};
// Export to app.js
module.exports.get = get
//Must include "powered by coindesk" with a link to price page

Rogier Nitschelm
iOS Development Techdegree Student 5,461 PointsThe JSON-response returns something along the lines of:
{
"time": { ... },
"bpi": {
"USD": {
"code": ...,
"rate": ...,
"description": ...,
etc..
}
}
}
There is no currentprice in there. So in order to get the data you want, you will have to dig through the data and get the 'rate' property.
const data = JSON.parse(d);
console.log(data.bpi.USD.rate);
3 Answers

Seth Kroger
56,413 PointsWith the HTTP/HTTPS response the 'data' event isn't necessarily (and probably not) the complete data, but the next chunk of it to be added to the rest. The 'end' event is what signals all the data has been fetched and the response is complete. You will usually have a string variable for the response data outside of the event handlers you concatenate to in the 'data' event and then parse it in the 'end'

Peter Gess
16,553 PointsSeth, thanks for your help on this project getting the data logged in the console. What would be the best way to render the result data to an HTML page? I have been able to use express and pug as a templating engine to display text, but am having trouble getting the "message" variable with the API call result to the page. Let me know if I can provide any code. Thanks for your continued help!

Peter Gess
16,553 PointsThanks guys. Rogier, that worked! To swift through the JSON are you using a linter or something like that?

Rogier Nitschelm
iOS Development Techdegree Student 5,461 PointsYou should try one of those pretty print json chrome extensions. This way you can just surf to the json-endpoint and view the response in a clear and structured way. :)

Peter Gess
16,553 PointsRoger, thanks for your help on this project getting the data logged in the console. What would be the best way to render the result data to an HTML page? I have been able to use express and pug as a templating engine to display text, but am having trouble getting the "message" variable with the API call result to the page. Let me know if I can provide any code. Thanks for your continued help!
Peter Gess
16,553 PointsPeter Gess
16,553 Points(https://www.coindesk.com/api/). The request is coming back fine but I am getting the output of "Current price of Bitcoin is undefined:. Please advise!