Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial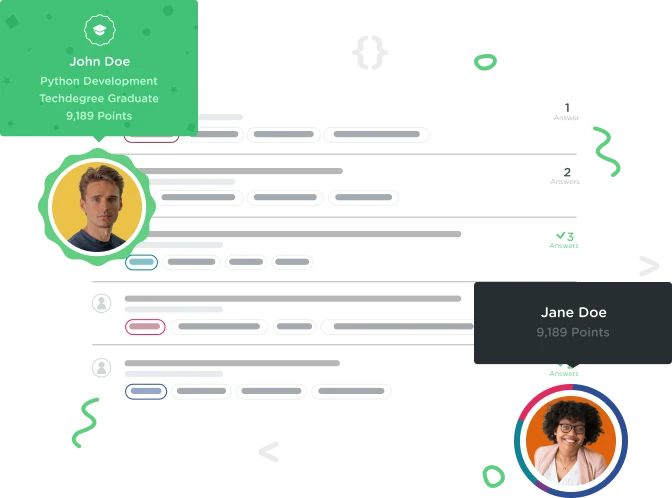

Sadettin Esenlik
1,423 PointsCannot pass challenge even though my answer is correct
I' m running the attached python script on my system and it returns disemvoweled word as expected, but when I click recheck work, I get that my code is wrong. What am I doing wrong ?
def disemvowel(word):
vowels = ["a","e","i","o","u"]
vowels = vowels + list("".join(vowels).upper())
letters = list(word)
for letter in letters:
if letter in vowels:
letters.remove(letter)
word = "".join(letters)
return word
1 Answer

andren
28,558 PointsThe problem is that you are removing items from a list while looping over that same list. Doing that will lead to items being moved around in the list while it is being looped over, which will result in some item being skipped by the loop.
The code mainly fails in cases where a vowel appears repeatedly, for example if you pass the string "aaaa" to your function it will return "aa" which is clearly not correct.
This can be fixed by using the string itself for the loop source instead of the list, like this:
def disemvowel(word):
vowels = ["a","e","i","o","u"]
vowels = vowels + list("".join(vowels).upper())
letters = list(word)
for letter in word:
if letter in vowels:
letters.remove(letter)
word = "".join(letters)
return word
Also just as a tip you can perform this task without having both lowercase and uppercase versions in the vowel list by calling lower
on the letter as you compare it to the vowels list. Like this:
def disemvowel(word):
vowels = ["a","e","i","o","u"]
letters = list(word)
for letter in word:
if letter.lower() in vowels:
letters.remove(letter)
word = "".join(letters)
return word
Which at least to me feels a bit cleaner than adding more letters to the vowels list.
Sadettin Esenlik
1,423 PointsSadettin Esenlik
1,423 PointsThanks for the answer. I see where I was mistaken now.