Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial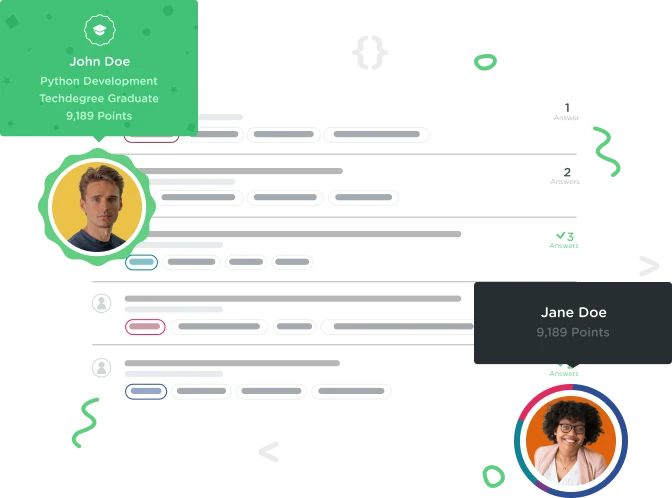
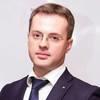
Dmitry Bruhanov
8,513 PointsCannot pass the coding challenge due to misunderstanding. I end up in Bummer! Error # 14876 - wrong coordinates received
Please, kindly advise what is wrong or missing in my code. Maybe I misunderstood the task. Thanks in advance for swift assistance.
public class GeolocationService extends Service {
int mLatitude;
int mLongitude;
private Messenger mMessenger = new Messenger(new LocationHandler(this));
// some methods omitted
@Override
public IBinder onBind(Intent intent) {
return mMessenger.getBinder();
}
private class LocationHandler extends Handler{
private GeolocationService mGeoService;
public LocationHandler(GeolocationService service){
mGeoService = service;
}
@Override
public void handleMessage(Message msg) {
mLatitude = getLatitude();
mLongitude = getLongitude();
}
}
}
7 Answers
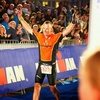
Steve Hunter
57,712 PointsSo for the second task, you add a Messenger
in the main class body, just as you have done but don't pass in this
as the constructor isn't liking that data type:
private Messenger mMessenger = new Messenger(new LocationHandler());
Then return the result of calling getBinder()
on it in onBind()
, as you have done.
The final code looks like:
public class GeolocationService extends Service {
int mLatitude;
int mLongitude;
private Messenger mMessenger = new Messenger(new LocationHandler());
// some methods omitted
@Override
public IBinder onBind(Intent intent) {
return mMessenger.getBinder();
}
class LocationHandler extends Handler{
@Override
public void handleMessage(Message msg) {
mLatitude = getLatitude();
mLongitude = getLongitude();
}
}
}
That should do it.
Steve.
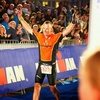
Steve Hunter
57,712 PointsHi there,
I think your code will work if you skip the constructor. That's being handled by the challenge in the "some methods omitted part".
I got this to work which looks the same as your code:
class LocationHandler extends Handler {
public void handleMessage(Message msg){
mLatitude = getLatitude();
mLongitude = getLongitude();
}
}
Steve.
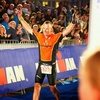
Steve Hunter
57,712 PointsHi Dmitry,
Have a look at the code i posted and check out the small differences to your code.
The code for the first task of the two-part challenge looks fine. I'm assuming that this works OK and allows you to get to task 2.
Leave that new internal class alone for task 2 - you don't need to change it any more. So, remove the lines of code you added to LocationHandler
after task 1. Leave it as:
class LocationHandler extends Handler{
@Override
public void handleMessage(Message msg) {
mLatitude = getLatitude();
mLongitude = getLongitude();
}
}
Next, for task 2, add the Messenger
as you have done but remove the parameter value of this
. That's causing a type mismatch so delete it. Just use the default constructor:
private Messenger mMessenger = new Messenger(new LocationHandler());
Your onBind()
method is fine as it it.
So, you need to remove this
and also remove these lines:
// these aren't required - remove them
private GeolocationService mGeoService;
public LocationHandler(GeolocationService service){
mGeoService = service;
}
Let me know how you get on - you shouldn't get any errors but if you do, click on Preview to see what the error is and let me know.
Steve.
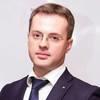
Dmitry Bruhanov
8,513 PointsHi, Steve! Thanks for your reply! I'll try omitting the constructor. However, it was already there when I opened the task, so it is not very likely to be the right solution. Thanks anyway! Good luck to you in coding))
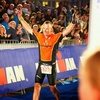
Steve Hunter
57,712 PointsI was on the wrong section ... I was looking at task 1 - you're on task 2. Let me have a go at that ...
Sorry!
Steve.
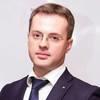
Dmitry Bruhanov
8,513 PointsHi, Steve.
the task is as follows:
Challenge Task 1 of 2
For this app, you need the user's location: latitude and longitude coordinates. But the only way to get these coordinates is by communicating with GeolocationService from your Activity (not shown). Start setting up GeolocationService by adding a custom Handler class named 'LocationHandler' as an inner class. Then override the 'handleMessage' method, and update the coordinates fields by using 'getLatitude()' and 'getLongitude()'; both return an integer.
This code results in: Bummer! Error #14876 - wrong coordinates received!
public class GeolocationService extends Service {
int mLatitude;
int mLongitude;
// some methods omitted
@Override
public IBinder onBind(Intent intent) {
return null;
}
//This is the new part, I added, the reast was here in the task
class LocationHandler extends Handler {
@Override
public void handleMessage(Message msg){
mLatitude = getLatitude();
mLongitude = getLongitude();
}
}
}
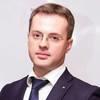
Dmitry Bruhanov
8,513 Pointspublic class GeolocationService extends Service {
int mLatitude;
int mLongitude;
private Messenger mMessenger = new Messenger(new LocationHandler(this)); // remove 'this'
// some methods omitted
@Override
public IBinder onBind(Intent intent) {
return mMessenger.getBinder();
}
private class LocationHandler extends Handler{
/* private GeolocationService mGeoService;
public LocationHandler(GeolocationService service){
mGeoService = service;
}
*/
@Override
public void handleMessage(Message msg) {
mLatitude = getLatitude();
mLongitude = getLongitude();
}
}
}
//Same result: Bummer! Error #14876 - wrong coordinates received!
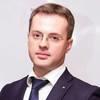
Dmitry Bruhanov
8,513 PointsSteve, thanks! I removed all the extra, like you suggested, and it worked! Thanks a lot!!! Good luck to you in your coding career!!!
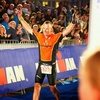
Steve Hunter
57,712 PointsGlad you got it fixed!