Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial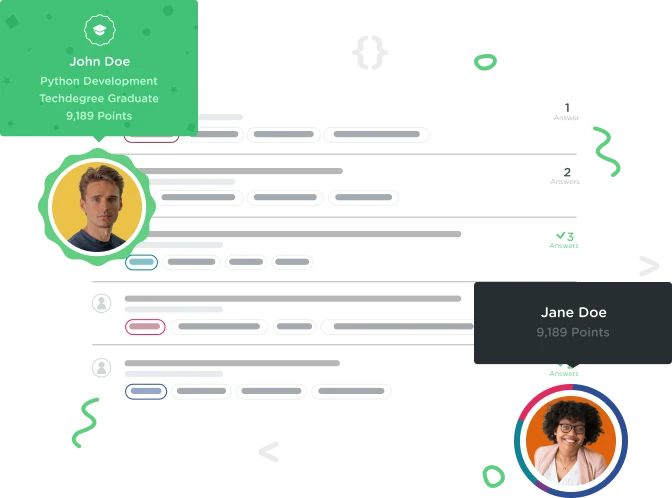

Leonard Fierro
1,123 PointsCannot pass the last Code Challenge in the Java Objects section of Learn Java Track
I can't seem to pass this last Code Challenge and it's getting really frustrating. I don't know what I'm doing wrong here.
It says to catch the Illegal Argument Exception in the code and warn the user. This is what I kept putting:
try { kart.drive(0); System.out.println("This will never happen"); } catch (IllegalArgumentException iae) { System.out.println("Not enough battery!"); System.out.printf("The error message is: %s\n", iae.getMessage()); }
I try deleting "kart.drive(2);", putting my code in before and after "kart.drive(2);", I feel like the problem is in "kart.drive(2);", but I'm not sure. I don't know what the 2 is supposed to represent - is it laps or battery bars? In the exercise before I set the Illegal Argument Exception to the bars count being anything less than 1, so it should only throw out the Illegal Argument Exception if "kart.drive(0);", right?? I'm so confused right now... Any help would be greatly appreciated. Thanks!
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
kart.drive(2);
try {
kart.drive(0);
System.out.println("This will never happen");
} catch (IllegalArgumentException iae) {
System.out.println("Not enough battery!");
System.out.printf("The error message is: %s\n", iae.getMessage());
}
}
}
1 Answer
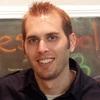
Rick Buffington
8,146 PointsI think that they really want you to go minimal on this one while still using their kart.drive method call. So what I did to pass this one was just that:
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try {
kart.drive(2);
}
catch (IllegalArgumentException iae) {
System.out.println("kart caused an error:" + iae);
}
}
}
I let the compiler get what it needed from iae - more than likely toString() - and kept their drive call. Hope this helps.
Leonard Fierro
1,123 PointsLeonard Fierro
1,123 PointsThanks for the response! Yep, that seemed to work! But, I'm still confused and don't understand... I coded the exercise before that to say that the bars count had to be less than 1 in order to pass the Illegal Argument Exception, so why does it pass the Illegal Argument Exception even though the value is 2?
Rick Buffington
8,146 PointsRick Buffington
8,146 PointsIt's more than likely that this part of the code instruction only includes a small bit of previous exercise work. They also have probably coded the GoKart class specific to this coding example so that it could fail when needed. I've learned that the best way to pass the code exercises is to do the code exactly how they tell you - and not to read into them. In your work space it's cool to play around and add your own exploratory code - however in the exercises they are executed a specific way looking for specific things. I understand your frustration, I've been their myself!
Leonard Fierro
1,123 PointsLeonard Fierro
1,123 PointsGotcha. Thanks for the advice! I'll try to remember that going forward.
Gonzalo Torres del Fierro
Courses Plus Student 16,751 PointsGonzalo Torres del Fierro
Courses Plus Student 16,751 PointsRick you deserve the best answer :)