Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial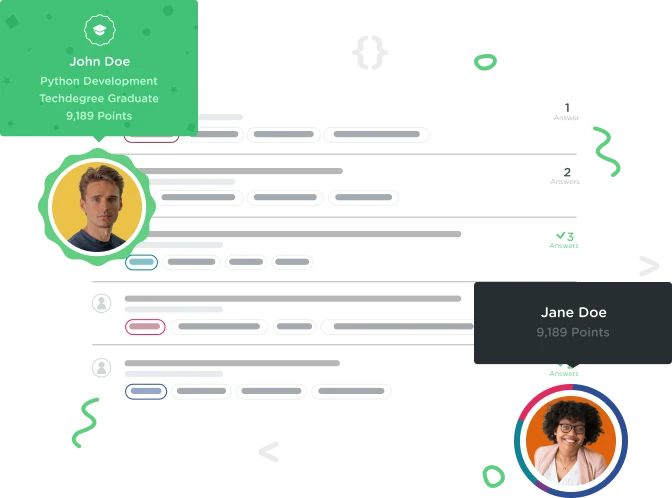
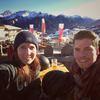
Mark Bojesen
12,873 Points"Cannot read property 'value' of null" - When the variables is declared and I would like to access the value.
Hi!
I am currently trying to build a Todo App with some different functionality in it. However I keep getting this error logged in the console which as far as I can understand implies I haven't really declared the variable I am trying to get the value from?
Error is created from the fourth last line in the bottom of the JS script, I have highlighted with a comment.
"Uncaught TypeError: Cannot read property 'value' of null at Object.addTodo (VM461 script.js:88) at HTMLButtonElement.onclick (VM491:21)"
Here is the HTML:
<div>
<button onclick="handlers.displayTodos()">Display Todos</button>
<button onclick="handlers.toggleAll()">Toggle All</button>
</div>
<div>
<button onclick="handlers.addTodo()">Add</button>
<input id="addTodoTextInput "type="text">
</div>
And the JavaScript
const todoList = {
todos: [],
displayTodos: function() {
if (this.todos.length === 0) {
console.log('Your todo list is empty.');
} else {
console.log('My Todos:');
for (var i = 0; i < this.todos.length; i++) {
if (this.todos[i].completed === true) {
console.log('(x)', this.todos[i].todoText);
} else {
console.log('( )', this.todos[i].todoText);
}
}
}
},
addTodo: function(todoText) {
this.todos.push({
todoText: todoText,
completed: false
});
this.displayTodos();
},
changeTodo: function(position, todoText) {
this.todos[position].todoText = todoText;
this.displayTodos();
},
deleteTodo: function(position) {
this.todos.splice(position, 1);
this.displayTodos();
},
toggleCompleted: function(position) {
const todo = this.todos[position];
todo.completed = !todo.completed;
this.displayTodos();
},
// Get number of completed todos
toggleAll: function() {
const totalTodos = this.todos.length;
let completedTodos = 0;
for (let i = 0; i < totalTodos; i++) {
if (this.todos[i].completed === true) {
completedTodos++;
}
}
// 1, If everything is true, make everything false
if (completedTodos === totalTodos) {
for (let i = 0; i < totalTodos; i++) {
this.todos[i].completed = false;
}
// 2, Otherwise make everything true
} else {
for (let i = 0; i < totalTodos; i++) {
this.todos[i].completed = true;
}
}
this.displayTodos();
}
};
// Handlers for onclick="" in HTML
const handlers = {
displayTodos: function() {
todoList.displayTodos();
},
toggleAll: function() {
todoList.toggleAll();
},
addTodo: function() {
var addTodoTextInput = document.getElementById('addTodoTextInput');
todoList.addTodo(addTodoTextInput.value); // << Line 88 is logged with error in console
addTodoTextInput.value = ''; //Clear back to default
}
};
Any help is super appreciated as I have been struggeling with this one for 3 evenings now, thanks in advance :)
1 Answer
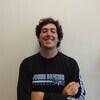
Charles Kenney
15,604 PointsHey Mark,
Anytime you see 'cannot get value of null', it means the element you have selected is non existent. If you take a quick look at your markup, you accidentally added a space when declaring the id property of the input.
<input id="addTodoTextInput "type="text">
If you fix that, you should be golden. Let us know if you need help with anything else!
-Charles
Mark Bojesen
12,873 PointsMark Bojesen
12,873 PointsHi Charles!
Waaauww I can't believe I didn't spot that. I have honestly had my head down 3 evenings in a row and was pulling hair out of my head in frustration.
Thank you so much for your quick response, that's amazing!
I guess you can never check enough for typos ;)
Charles Kenney
15,604 PointsCharles Kenney
15,604 PointsHaha, no problem. It happens to everyone!