Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial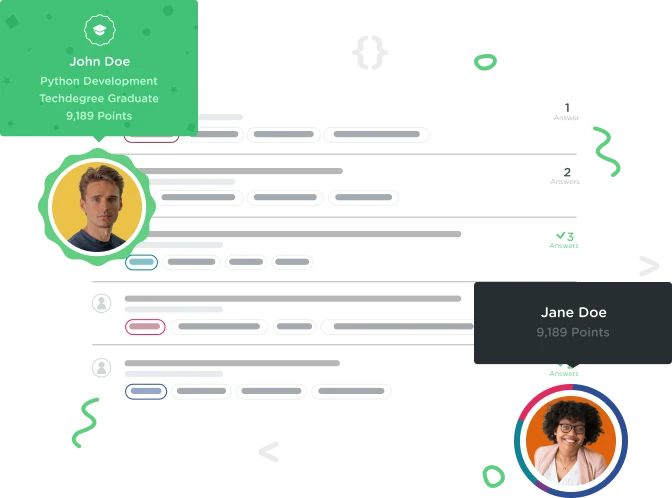
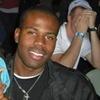
Ryan White
33,388 PointsCannot resolve symbol 'JsonSerializer'
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
using Newtonsoft.Json;
namespace SoccerStats
{
class Program
{
static void Main(string[] args)
{
string currentDirectory = Directory.GetCurrentDirectory();
DirectoryInfo directory = new DirectoryInfo(currentDirectory);
var fileName = Path.Combine(directory.FullName, "SoccerGameResults.csv");
var fileContents = ReadSoccerResults(fileName);
fileName = Path.Combine(directory.FullName, "players.json");
var players = DeserializePlayers(fileName);
foreach (var player in players)
{
Console.WriteLine(player.second_name);
}
}
public static string ReadFile(string fileName)...
public static List<GameResult> ReadSoccerResults(string fileName)...
public static List<Player> DeserializePlayers(string fileName)
{
var players = new List<Player>();
var serializer = new JsonSerializer(highlighted in red) ();
using (var reader = new StreamReader(fileName))
using (var jsonReader = new JsonTextReader(highlighted in red)(reader))
{
players = serializer.Deserialize<List<Player>>(jsonReader);
}
return players;
}
}
}
I imported Newtonsoft.Json and Newtonsoft.Json.Schema to try to find reference.
Moderator edited: Markdown added so that code renders properly in the forums.
2 Answers

Karlijn Willems
9,961 Pointsvar serializer = new JsonSerializer(highlighted in red) (); using (var jsonReader = new JsonTextReader(highlighted in red)(reader))
What is the "highlighted in red" for? The syntax (highlighted in red)(); seems a bit odd to me... I think that should be (some parameter, another parameter);

Deji Adeyemo
5,146 PointsThe correct lines of code are:
var serializer = new JsonSerializer();
using (var jsonReader = new JsonTextReader(reader))
In both cases, it seems you have additional '()' hence the reason they are highlighted in Red.