Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial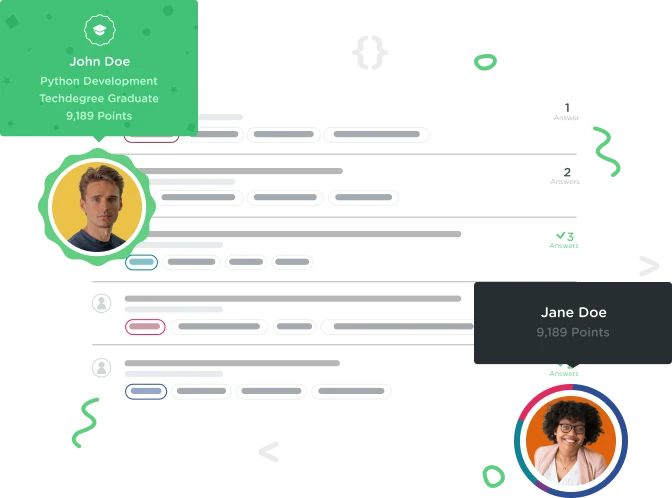

Robert Farrimond
2,654 PointsCannot return my_dict.items() and unsure what the error is.
I'm very close to the solution here but I'm just unsure as to why my code currently causes an error. Can anyone shed some light on this?
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
# If you use .append(), you'll want to pass it a tuple of new values.
def combo(it_1, it_2):
my_dict = {}
for one, two in zip(it_1, it_2):
my_dict[one] = two
return my_dict.items()
3 Answers
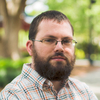
Kenneth Love
Treehouse Guest TeacherYour code actually found a mistake in my code challenge. You actually shouldn't be able to pass with dict.item()
because dicts are unsorted and combo
needs to be in a particular order. I've updated the code challenge to better test this.
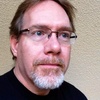
Chris Freeman
Treehouse Moderator 68,423 PointsThe simple fix, using your current code, is to convert the iterable my_dict.items()
into a list is use list
:
return list(my_dict.items())
Another solution is to build up a list of tuples instead of using a dict
:
def combo(it_1, it_2):
my_list = []
for one, two in zip(it_1, it_2):
my_list.append((one, two))
return my_list

Robert Farrimond
2,654 PointsThanks Chris, I actually just got to that second answer myself, but thanks for the insight on list(my_dict.items()) that's much simpler!
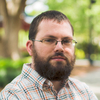
Kenneth Love
Treehouse Guest Teacherdict.items()
doesn't return a tuple, it returns an iterable named dict_items
.

Robert Farrimond
2,654 PointsYes but the code challenge does not ask for a tuple to be returned, it asks for a list of tuples, and since a list is iterable items() should work I thought? The python documentation even says that items() returns a list of tuples, which is exactly what is asked for in the code challenge so I'm still not sure why my code is not correct.
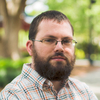
Kenneth Love
Treehouse Guest TeacherYou're right, it's not asking for a tuple, it's asking for a list of tuples. But, again, dict.items()
doesn't return a list of tuples. It returns dict_items
which is a dict view
(link there for more information). If you're finding docs that say dict.items()
returns a list, you must be looking at Python 2 and not Python 3. Your solution is close to a way of doing this but, if you want to keep using dict.items()
, you'll need to change that output to a list explicitly.

Robert Farrimond
2,654 PointsOk thanks Kenneth, I did try my_list = my_dict.items() then returning my_list but I got the same error. I'm going to come back to this tomorrow as I suspect a good night's sleep might help me on this better than staying up past 1am staring at my laptop!
Robert Farrimond
2,654 PointsRobert Farrimond
2,654 PointsAh yes, that makes sense. Just completed the code challenge using a list rather than a dict, it did make more sense after a good night's rest ;)