Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial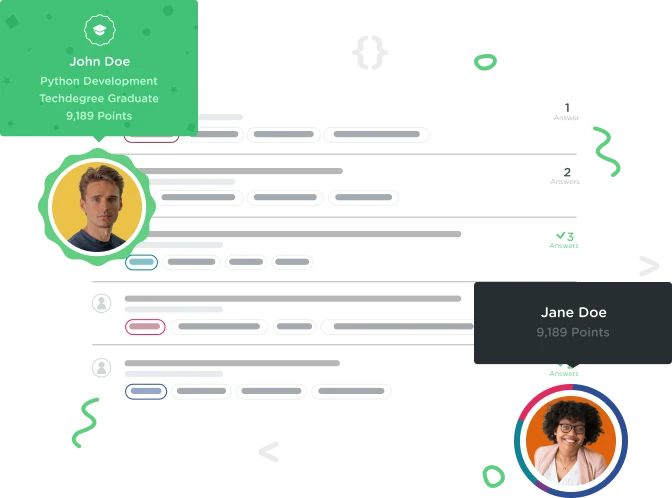

viou
362 PointsCannot solve this question. does anyone can help me out please? thanks
Cannot solve this question. does anyone can help me out please? thanks
available = "banana split;hot fudge;cherry;malted;black and white"
'available'.split()
sundaes = ['available']
11 Answers
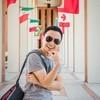
hamsternation
26,616 PointsHey! Let's take a look at what you have:
def printer(count):
count = 0
while count < 100:
print("Hi")
count += 1
printer(10)
So your goal is to define a function called printer
, which you have done here, and have it print "Hi " as many times as the count argument.
So while your function does print count, it doesn't print the right number of "Hi "s, in fact, it actually always prints 100 "Hi "s.
Since you overwrite the variable / argument count
in line 2, count always starts at 0 in your while loop, and it always iterates it 100 times.
What you want is a bit simpler, since we know that strings can be multiplied with an integer ("Hi " * 5 = "Hi Hi Hi Hi Hi "), we can take advantage of this fact and assume that the count argument is always an integer, making the code much simpler:
def printer(count):
print("Hi " * count)
That way, whatever count argument comes in, gets multiplied and printed all in the same line. :)
Remember, the zen of python says that simple is better than complex, do your best to see if there's an easier way of doing the same thing. :)
Hope this helps! Looks like you're coming along at a good pace! Keep it up! :)
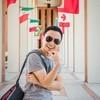
hamsternation
26,616 PointsA couple things, first, putting quotes around 'available' makes it a string. In other words, you want the variable available
and not simpley the word 'available' to split, i.e.: available.split()
also, split method takes in a string argument, or what you want it to split. in this case, available.split(";")
note that the quotes are needed inside the method call.
finally, splitting the string available results in a list of outputs, i.e:
>>> available = "banana split;hot fudge;cherry;malted;black and white"
>>>
>>> available.split(";")
['banana split', 'hot fudge', 'cherry', 'malted', 'black and white']
so you can simply assign sundaes
to available.split(";")
in otherwords:
available = "banana split;hot fudge;cherry;malted;black and white"
sundaes = available.split(";")
Moderator Edit: Moved response from Comments to Answers

viou
362 PointsThank you again!!! :)

viou
362 PointsHello Hamsternation, thank you again. You right I have to think in a much simpler way. Thank again
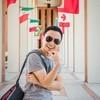
hamsternation
26,616 PointsNo problem! You got this!

viou
362 Pointshamsternation thank you a lot for your help. :)

viou
362 Pointscan't figure out this question. Can you help out please? thank you
banana.py available = "banana split;hot fudge;cherry;malted;black and white" available.split(';') sundaes = available.split(';') menu = "Our available flavors are: {}." ", ".join(sundaes) display_menu = sundaes menu.format(display_menu)
I steal have an error message, yet it works while using python shell
sundaes = available.split(";")
sundaes ['banana split', 'hot fudge', 'cherry', 'malted', 'black and white'] (", ").join(sundaes) 'banana split, hot fudge, cherry, malted, black and white' display_menu = sundaes menu.format(display_menu) "Our available flavors are: ['banana split', 'hot fudge', 'cherry', 'malted', 'black and white']"
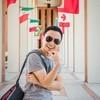
hamsternation
26,616 PointsOkay so when you type code on treehouse, use triple backticks (the button above your tab button) checkout the Markdown Cheatsheet for more information.
This makes the code a lot easier to read.
But here's the code I think you have:
available = "banana split;hot fudge;cherry;malted;black and white"
available.split(';')
sundaes = available.split(';')
menu = "Our available flavors are: {}."
", ".join(sundaes)
display_menu = sundaes
menu.format(display_menu)
so you don't actually need the second available.split(':')
line, it doesn't really do anything since it's not assigned to any variable nor returned to anything. it just disappears, so you can get rid of it.
so I think what you're missing is that sundaes
created a list of menu items, which looks like this:
['banana split', 'hot fudge', 'cherry', 'malted', 'black and white']
you then assigned sundaes to display_menu, which means the variable display_menu is just another copy of sundae, which as a reminder, still looks like this:
['banana split', 'hot fudge', 'cherry', 'malted', 'black and white']
so when you menu.format(display_menu), you're sticking literally the list into the bracket, making the answer wrongly formatted.
"Our available flavors are: ['banana split', 'hot fudge', 'cherry', 'malted', 'black and white']"
what you want is:
"Our available flavors are: banana split, hot fudge, cherry, malted, black and white."
you had the right idea with the ", ".join(sundaes)
but you didn't assign it to anything.
", ".join(sundaes)
returns the string: 'banana split, hot fudge, cherry, malted, black and white'
what you want to do is assign that to the display_menu as such:
display_menu = ", ".join(sundaes)
so now, display_menu is the string: 'banana split, hot fudge, cherry, malted, black and white'
now finally, use the menu.format(display_menu) and now you should have the right answer.
Good luck!

viou
362 PointsHello Hamstenation: thank you again for helping me out. really appreciated. I got my mistake now, go from a list to a string by using a string.
Listen, I've tried what you said in the shell it worked without the [ ], but in treehouse it does not and I don't know why the following message below still pop up : Bummer! Did you use ", ".join()
?
if you can take a look at it'd be great and again I'm sorry for bothering you see below
available = "banana split;hot fudge;cherry;malted;black and white"
available.split(";")
sundaes = available.split(";")
menu ="Our available flavors are:{}"
display_menu =",".join(sundaes)
menu.format(display_menu)
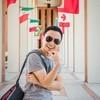
hamsternation
26,616 Pointsoh okay I see the challenge now.
First off, you made a syntax error with the
display_menu=",".join(sundaes)
part of your code. you're missing a space after the comma. Your code yields a list of sundaes with just comma and no space.
So about the challenge, you have to be very carefully with the instructions:
Alright, let's finish making our menu. Combine the sundaes list into a new variable named display_menu, where each item in the list is rejoined together by a comma and a space (", "). Then reassign the menu variable to use the existing variable and .format() to replace the placeholder with the new string in display_menu. If you're really brave, you can even accomplish this all on the same line where menu is currently being set.
The key word is that they want you to reassign the menu variable
That means the variable menu becomes the string "Our available flavors are: banana split, hot fudge, cherry, malted, black and white."
Basically you're missing the reassignment back to menu:
available = "banana split;hot fudge;cherry;malted;black and white"
sundaes = available.split(";")
menu ="Our available flavors are:{}."
display_menu =", ".join(sundaes)
menu = menu.format(display_menu)
A more "brave" version they're talking about is doing this in 1 step. It effectively assigns menu while formatting the join strings. phew.
available = "banana split;hot fudge;cherry;malted;black and white"
sundaes = available.split(";")
menu ="Our available flavors are:{}.".format(', '.join(sundaes))
Hope this helps! best of luck! :)

viou
362 PointsOMG you saved my day. I have to work harder!! thanks again.

viou
362 PointsHello Hamsternation,
I hope all is well. listen could you help me out again please. can pass the 2nd Q Challenge Task 1 of 2
I'm going to create a variable named age. I need you to make an if condition that sets admitted to True if age is 13 or more. Important: In each task of this code challenge, the code you write should be added to the code from the previous task. Restart Get Help Check Work conditions.py
True
1
admitted = None
2
age <= 13
3
if age <=13 and admitted:
4
admitted = False
5
else:
6
` admitted = True
2Q:Oops! It looks like Task 1 is no longer passing. and I don't know why
admitted = None
age <= 13
if age <=13 and admitted:
admitted = False
elif: age >=13 and admitted
admitted = True
else:
admitted = False
Oops! It looks like Task 1 is no longer passing.
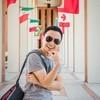
hamsternation
26,616 PointsHello! This task is actually a bit more straightforward than what you're thinking, let's break it down.
So let's start with your code for challenge 1:
admitted = None
age <= 13
if age <=13 and admitted:
admitted = False
else:
admitted = True
Let's see what your 2nd line of code does: age <= 13
The variable age is either greater than 13 or equal to 13 or less than 13, depending on what the challenge assigns, which we don't know.
Let's say the challenge makes "age = 15"
>>> age <= 13
False
If the challenge makes "age = 13"
>>> age <=13
True
I hope you see how line 2 of your code doesn't actually do anything in terms of the program other than returning a True or False that doesn't get used up for anything else, so we actually don't need line 2 of your code.
Now let's break down your if statement and why it passed challenge 1, but probably isn't for the reason you think it did:
admitted=None
if age <=13 and admitted:
admitted = False
else:
admitted = True
Let's say age = 15, then if age <=13
would return True
and admitted
(which is equal to "None") would return False
as such:
if True and False:
True and False
would be evaluated as False
so it triggers the else
, which sets admitted to True
it turns out that this challenge passes as long as you have an if statement and you've somehow set admitted to True
Which is a bug. So a meaningless code like this would pass this challenge:
admitted = None
if age:
admitted = True
So let's figure out how to actually solve this:
The challenge asks:
I need you to make an if condition that sets admitted to True if age is 13 or more.
You basically build it as you read it:
- create an if condition
- if age is 13 or more, then
- set admitted to True
so as follows:
if age >= 13: #create an if condition that tests if age is 13 or more
admitted = True # set admitted to True
We don't actually need to worry about the else yet, but if you want to see the 2nd part:
OK, one more. Add an else to your if. In the else, set admitted to False.
if age >= 13: #create an if condition that tests if age is 13 or more
admitted = True # set admitted to True
else:
admitted = False # if it doesn't meet the if condition, set admitted to False
Ahhhh the zen of python.... :)

viou
362 PointsHello Hamsternation, I hope all is well. I need you help again i f you can please.
here is the challenge: Challenge Task 1 of 1
Write a function named printer. The function should take a single argument, count, and should print "Hi " as many times as the count argument. Remember, you can multiply a string by an integer.
and my code is
def printer(count):
count = 0
while count < 100:
print("Hi")
count += 1
printer(10)
I ran the code into the shell it worked but I have an incorrect answer "Bummer! Didn't find the right number of "Hi"s.
if you can help me out again please
thanks!!!