Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial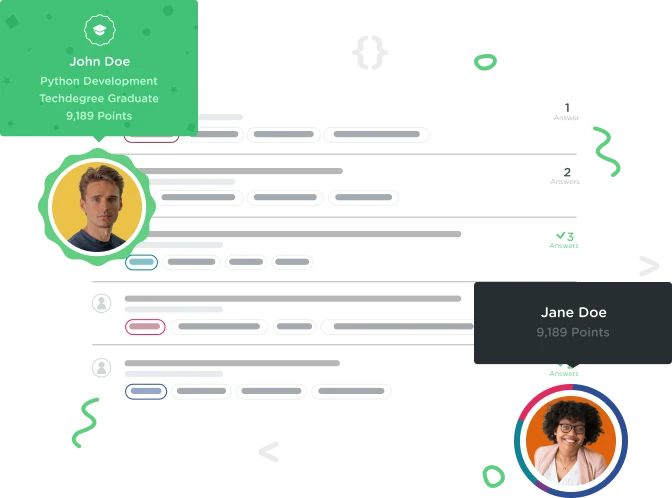

siemkazer
3,276 PointsCannot understand the mechanism of/logic behind the compareTo()
part 1: I cannot seem to understand how the compare() method is comparing the treets. Shouldn't there be two items passed into the method's argument ? ex: compareTo(Treet treet1, Treet, treet2). The only thing I can see is Arrays is calling the sort method and then somehow the items are getting compared using "This". How is the method compareTo() deciding which treet is "This" and which treet is "other" ?
part 2: Why are we casting Object there inside of the compareTo() ? I mean why aren't we passing a Treet into compareTo() in the first place?. This seems to me counter intuitive !
2 Answers

Lars Reimann
11,816 Points1) The sort method is calling treet1.compareTo(treet2)
. Within compareTo
we can access treet1
via this
and treet2
obviously via treet2
. So calling a method with one parameter is practically the same as calling a function in a procedural language with two parameters. this
is an implicit first argument.
To elaborate on this here is an excerpt of the code Craig wrote:
public int compareTo(Object o) {
Treet other = (Treet) o;
// ...
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
To make this more explicit, we can add this
when we access mCreation date of the current Treet. The this
is not needed to be there.
public int compareTo(Object o) {
Treet other = (Treet) o;
// ...
int dateCmp = this.mCreationDate.compareTo(other.mCreationDate);
Java expects a function with that signature (name, parameters etc.), but behind the scenes compareTo has an additional parameter and gets this
as first argument. You can think of the function as follows (note the static
). o1
is assigned the value for this
.
public static int compareTo(Object o1, Object o2) {
Treet treet1 = (Treet) o1;
Treet treet2 = (Treet) o2;
// ...
int dateCmp = treet1.mCreationDate.compareTo(treet2.mCreationDate);
2) You would normally do that, but it seems like Craig wanted to avoid using generics for now. You would need to implement the interface Comparable<Treet>
for this to work.

shu Chan
2,951 PointsFantastic, I get it now. Thanks Lars!
shu Chan
2,951 Pointsshu Chan
2,951 Points@Lars Reimann Could you elaborate on this?
"Within compareTo we can access treet1 via this and treet2 obviously via treet2. So calling a method with one parameter is practically the same as calling a function in a procedural language with two parameters. this is an implicit first argument."
Lars Reimann
11,816 PointsLars Reimann
11,816 PointsI added some more information. If you are unsure about what the
this
means, I'd suggest you go over the material of https://teamtreehouse.com/library/java-objects-2.michaelcodes
5,604 Pointsmichaelcodes
5,604 PointsThank you for this information it is extremely helpful! This cleared up all my confusion, including what was being compared. The "if (equals(other))" I now see that "this" is implied here!