Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial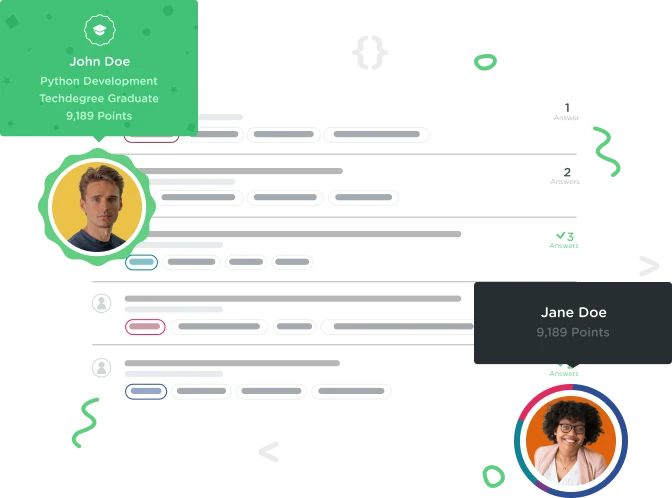

Luca Di Pinto
8,051 PointsCannot understand when using parentNode!
Hi guys,
can somebody explain me why and when to use parentNode? It's not clear to me this step! In the previous stage we learned hot to remove an element with removeChild and it's not so clear what's the difference with this example. Why in the previous stage we used only removeChild and we cannot use removeChild also in this step? What is the reference Guil speaks about in this stage? Is maybe regarding for this reference that we have to use parentNode? HELP! I cannot understand!!!
7 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Luca,
I think that there's maybe 2 issues here. One is, what do we need to use .removeChild()
properly? And when is .parentNode
needed?
To use removeChild() properly, you need both the element that you want to remove and the parent of that element.
You can think of it like this:
parentElement.removeChild(childElementToRemove);
You need both the parent and the child of that parent that you would like removed. This will always be true no matter what situation you're in.
Sometimes you'll be able to accomplish this without needing .parentNode
. Other times, you'll need .removeChild() in combination with .parentNode to get the job done.
Maybe a simple example will help.
Suppose we have the following html:
<p>This is a paragraph.<span> It contains a span</span></p>
and the goal is to remove that span element.
Suppose the following js code to start off with
var span = document.querySelector("span");
var p = document.querySelector("p");
I have references to both the span
that I'm trying to remove as well as the parent of that span which is the paragraph element. I have access to both of these elements through those 2 variables.
I can remove the span with
p.removeChild(span);
In this case, I could do it without needing .parentNode
because I already had the parent stored in the variable p
On the other hand, let's say we have the following code
var span = document.querySelector("span");
In this case, I only have a reference to the element I want to remove. I don't have a reference to the parent. I'm pretending that I don't know it's the paragraph.
This is where .parentNode
is useful. It will get the parent for us so that we can properly use removeChild()
var parent = span.parentNode; // save a reference to the parent of the span in the variable parent
parent.removeChild(span); // use that parent to call removeChild and pass in the child span
.parentNode
can be used in any situation where you don't already know what the parent is. Either you don't know what the parent is or you simply don't have a reference to it saved in a variable.
This is what the example in this video is showing with the code inside the event listener.
The teacher first gets the LI that should be removed usingevent.target
let li = event.target;
At this point, we can't remove that li with removeChild()
because we don't have a reference to the parent of that li.
This is why the teacher uses .parentNode
, because it will give us a reference to the parent of that li.
let ul = li.parentNode;
Now we have both things that we need in order to use removeChild() properly.
let li = event.target;
let ul = li.parentNode;
ul.removeChild(li);
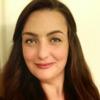
Jennifer Nordell
Treehouse TeacherHi there, Luca! When removing a child element we have to "refer" to the parent node. And what I mean by that is we have to tell it specifically which parent this child belongs to. Imagine for a moment that we have code that looks a bit like this:
<div id="first-div">
<p> This is the first div </p>
</div>
<div id="second-div">
<p> This is the second div </p>
</div>
Now imagine that we want to remove the second paragraph by using remove child. We have to first select the element that contains that paragraph. In this case it's the div with the id "second-div". Then we use removeChild to remove the paragraph inside that div. The div is the "parent" because it contains the paragraph. The paragraph is the child because it is contained within the div.
When using removeChild you will always have to tell it which parent you're removing the child from. And this is what we mean by a "reference".
Hope this clarifies things!
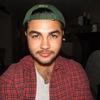
Asai Andrade
Full Stack JavaScript Techdegree Student 2,049 PointsI appreciate your explanation of this particular subject.
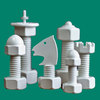
Steven Parker
231,268 PointsThese are very different things.
The parentNode is an element property that refers to the node that contains this one, also known as the "parent" node. Making a reference to this property does not (by itself) cause any changes in the document.
On the other hand, removeChild() is a method that will take an element out of the document as a permanent change.
You might use these together if you have variable representing an element you want to remove. You could use parentNode to refer to its parent, and then apply the removeChild method to that parent.
Does that help clear things up?
The removeChild method needs both the parent and the child.
When you use removeChild, you need a reference to both the parent and child elements, since you call it like this:
<parent>.removeChild(<child>);
So, if you start with only the element you want to remove (in this case the paragraph is the child), then you would use the parentNode property to get the reference to the parent. Then when you have both you can use the method.

Luca Di Pinto
8,051 PointsHi Steven,
not so clear sorry! So you mean that we do not have strictly use parentNode and removeChild together, right? At the beginning of the video Guil says that we want to remove a paragraph from the DOM and we need to call removeChild on the paragraph's parent but we do not have a reference to the parent. What does this mean? If our goal is simply to remove the paragraph, could not we use only removeChild? Sorry, but I think this step is a little difficult to understand!
Thanks again everyone!

Luca Di Pinto
8,051 PointsSo when do we have to use removeChild and when parentNode? Can you make an example? And what does it mean "reference"?
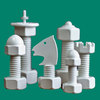
Steven Parker
231,268 PointsPerhaps a different viewpoint may help....

Luca Di Pinto
8,051 PointsThank you Jennifer!
Ok I understand what does it mean "reference" now.
But now I have a trouble. In which situations we do not have a reference so to have to use parentNode?
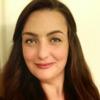
Jennifer Nordell
Treehouse TeacherI apologize. I'm not sure I understand your question exactly. But let me give you an example that has nothing to do with coding and see if that helps.
Let's say I just started running a daycare for toddlers. But I don't yet know any of the toddlers names, I only know their parents' names. The parents are:
- Bill and Sue
- Tom and Linda
- Mike and Sandy
When I get there on the first day, my assistant runs over to me and says "The child is allergic to peanuts and we just served peanut butter sandwiches. Oh no!". But that doesn't help me because I have no idea which child the assistant means. I only know the names of the parents. So I have to ask the assistant to please "refer to" or name the parents. In this case, the assistant says "Tom and Linda's child".
When using removeChild() you will always have to say from which parent. Otherwise, it will never know to which parent you're referring. This can be seen in this MDN documentation. As you can see the parent element must first be referenced and then the removeChild method. Hope this helps!
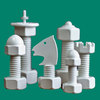
Steven Parker
231,268 PointsI thought the video gave a great example in the case of a an event handler, where you only have the event target to start with.

Luca Di Pinto
8,051 PointsThanks a lot Jennifer for your patience! Sorry but I'm a newbie and this part is a little bit difficult to understand! :-)
It's a little bit clear now but I still cannot understand when I have to use parentNode. For example which is the difference when in the third stage "Removing Nodes" we used only removeChild without using parentNode? Passing your example to code, in which situation I could know only the parent and not the element? If I know only the parent I should use parentNode and if I know even the element I could use removeChild? Is it right?

Jason Anello
Courses Plus Student 94,610 PointsHi Luca,
Does the latest comment from Steven help you?
Also, please use the "Add Comment" link if you're replying to someone's comment or answer instead of leaving a new answer. It helps keep the conversation better organized.

Luca Di Pinto
8,051 PointsOps sorry Jason! Last answers helped me a little but I posted another question to Jennifer.. :-)
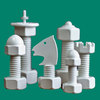
Steven Parker
231,268 PointsRemember what I said the second time.
The removeChild method needs both the parent and the (child) element. If you start with only the parent, you could use .children[n] to get the child. If you start with only the element (like in an event handler) you could use .parent to get the parent.
But you must have both to use .removeChild().
Luca Di Pinto
8,051 PointsLuca Di Pinto
8,051 PointsOk I understand now! But is not easier to insert in the code a declaration where i get a reference to the "p" element instead of using parentNode??
Steven Parker
231,268 PointsSteven Parker
231,268 PointsBy using parentNode you get the specific element that is the parent to the event target. If you get an element using the tag name you could not be certain it is the right one.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsYes, sometimes you will already have a reference to the parent and you won't have to use parentNode.
But there will be times where you don't know what the parent is or maybe it's not convenient to save several references to various parent elements and it will be more convenient to use parentNode in those situations.
Asai Andrade
Full Stack JavaScript Techdegree Student 2,049 PointsAsai Andrade
Full Stack JavaScript Techdegree Student 2,049 PointsReading the explanation above and your explanation, really gave me the confidence to implement the use of
parentNode
andremoveChild()
. Thank you!cyber voyager
8,859 Pointscyber voyager
8,859 PointsThank you very much Jason, I appreciate your effort to make our lives easier <3
Saqib Ishfaq
13,912 PointsSaqib Ishfaq
13,912 Pointsthanks alot for this, was so confused abt the whole concept!
Munish Sharma
Full Stack JavaScript Techdegree Student 5,144 PointsMunish Sharma
Full Stack JavaScript Techdegree Student 5,144 PointsThe best explanation .. Thank you so much !!! :)