Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial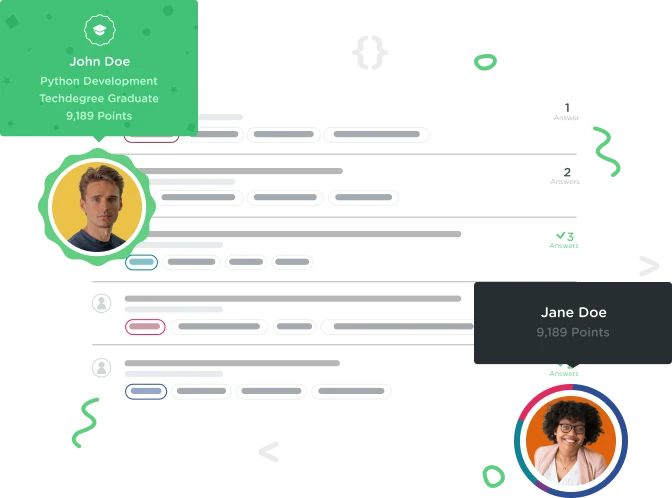
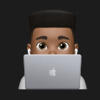
Caesar Bell
24,829 PointsCan't access user profile, (error "Moved Permanently")
Using the code as instructed in the course and I am getting an error message "Moved Permanently". Is anyone else getting this error ?
following code
//Router page
var Profile = require("./profile.js");
var renderer = require("./renderer.js");
var querystring = require("querystring");
var contentType = {'Content-Type': 'text/html'};
//Handle HTTP route GET / and POST / i.e. Home
function home(request, response) {
//if url == "/" && GET
if(request.url === "/") {
if(request.method.toLowerCase() === "get"){
//show search
response.writeHead(200, contentType);
renderer.view("header", {}, response);
renderer.view("search", {}, response);
renderer.view("footer", {}, response);
response.end();
}else{
//if url == "/" && POST
//get the post data from body
request.on("data", function(postBody){
//extract the username
var query = querystring.parse(postBody.toString());
//redirect to /:username
response.writeHead(303, {"location": "/" + query.username});
response.end();
});
}
}
}
//Handle HTTP route GET /:username i.e. /chalkers
function user(request, response) {
//if url == "/...."
var username = request.url.replace("/", "");
if(username.length > 0) {
response.writeHead(200, contentType);
renderer.view("header", {}, response);
//get json from Treehouse
var studentProfile = new Profile(username);
//on "end"
studentProfile.on("end", function(profileJSON){
//show profile
//Store the values which we need
var values = {
avatarUrl: profileJSON.gravatar_url,
username: profileJSON.profile_name,
badges: profileJSON.badges.length,
javascriptPoints: profileJSON.points.JavaScript
}
//Simple response
renderer.view("profile", values, response);
renderer.view("footer", {}, response);
response.end();
});
//on "error"
studentProfile.on("error", function(error){
//show error
renderer.view("error", {errorMessage: error.message}, response);
renderer.view("search", {}, response);
renderer.view("footer", {}, response);
response.end();
});
}
}
module.exports.home = home;
module.exports.user = user;
Any ideas on what might be causing the issue?
6 Answers

Milo Winningham
Web Development Techdegree Student 3,317 PointsIt looks like you're missing a /
at the end of the URL now.

Milo Winningham
Web Development Techdegree Student 3,317 PointsWe recently upgraded the Treehouse website so every page is served securely over HTTPS, but this code doesn't follow the redirect when loading a student profile like browsers normally do. Try opening up profile.js and changing require('http')
to require('https')
, and http://teamtreehouse.com
to https://teamtreehouse.com
.
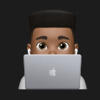
Caesar Bell
24,829 PointsMade the changes but now I get an error message "throw er; // Unhandled 'error' event"
var EventEmitter = require("events").EventEmitter;
var http = require("https");
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = http.get("https://teamtreehouse.com" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"));
}
//Read the data
response.on('data', function (chunk) {
body += chunk;
profileEmitter.emit("data", chunk);
});
response.on('end', function () {
if(response.statusCode === 200) {
try {
//Parse the data
var profile = JSON.parse(body);
profileEmitter.emit("end", profile);
} catch (error) {
profileEmitter.emit("error", error);
}
}
}).on("error", function(error){
profileEmitter.emit("error", error);
});
});
}
util.inherits( Profile, EventEmitter );
module.exports = Profile;
Could there be another issue causing the error ?

Justin Carver
15,887 PointsWell that explains why my jquery request broke. I'll have to check this when I get home to see if I can fix it.
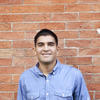
Wilson Usman
35,206 PointsWhat's weird is that it will work if I directly write /chalkers, but once I refresh I get an error:
``` profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"));
Any advice?
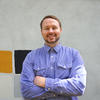
Tom Geraghty
24,174 PointsFollowing what Milo said above, you have to make changes to the profile.js file so it calls https instead of http. You can read his instructions and work it out, or, for the lazy:
var EventEmitter = require("events").EventEmitter;
var https = require("https");
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + " (" + response.statusCode + ")."));
}
//Read the data
response.on('data', function (chunk) {
body += chunk;
profileEmitter.emit("data", chunk);
});
response.on('end', function () {
if(response.statusCode === 200) {
try {
//Parse the data
var profile = JSON.parse(body);
profileEmitter.emit("end", profile);
} catch (error) {
profileEmitter.emit("error", error);
}
}
}).on("error", function(error){
profileEmitter.emit("error", error);
});
});
}
util.inherits( Profile, EventEmitter );
module.exports = Profile;
If you replace profile.js with the above code the app works!
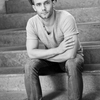
Rune Rapley-Møller
Courses Plus Student 24,411 Pointshttp.STATUS_CODES is deprecated: Use http.request() instead.

Curtis Slone
Courses Plus Student 13,318 PointsI did this awhile ago, the app works in node but doesn't load the web page itself.

Curtis Slone
Courses Plus Student 13,318 PointsI did this awhile ago, the app works in node but doesn't load the web page itself.
Caesar Bell
24,829 PointsCaesar Bell
24,829 PointsThank you so much, it works now.