Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial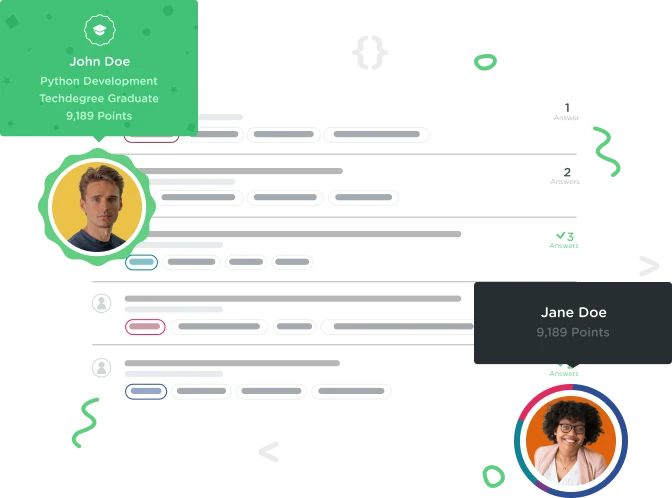
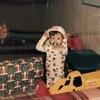
jahabeebs
3,804 PointsCan't add more than one item to list
For some reason, after I try to add any second item to my list (in this video, after adding "apples") my program simply doesn't add it. I've been looking for an error in the code but there's so many elements to this program that I can't figure it out.
import os
shopping_list = []
def clear_screen():
os.system("cls" if os.name == "nt" else "clear")
def show_help():
clear_screen()
print("What should pick up at the store?")
print("""
Enter "DONE" to stop adding items.
Enter "HELP" for this help.
Enter "SHOW" to see your current list.
Enter "REMOVE" to delete an item from your list.
""")
def add_to_list(item):
show_list()
if len(shopping_list):
position = input("Where should I add {}?\n"
"Press ENTER to add to the end of the list\n"
"> ".format(item))
else:
position = 0
try:
position = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(position-1, item)
else:
shopping_list.append(new_item)
show_list()
def show_list():
clear_screen()
print("Here's your list:")
index = 1
for item in shopping_list:
print("{}. {}".format(index, item))
index += 1
print("-"*10)
def remove_from_list():
show_list()
what_to_remove = input("What would you like to remove?/n> ")
try:
shopping_list.remove(what_to_remove)
except ValueError:
pass
show_list()
show_help()
while True:
new_item = input("> ")
if new_item.upper() == "DONE" or new_item.upper() == "QUIT":
break
elif new_item.upper() == "HELP":
show_help()
continue
elif new_item.upper() == "SHOW":
show_list()
continue
elif new_item.upper() == "REMOVE":
remove_from_list()
else:
add_to_list(new_item)
show_list()
2 Answers
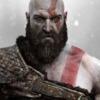
boi
14,242 PointsBOY, your problem of "items not adding in the list" is in the "add_to_list" function. You're (try) block is indented inside the (first else) block. or in other words, your try block is in the else block. make the indentation of the try block the same as the first else block, and your problem is solved.
remember what John Cavanah said? "Python is sensitive to indentation" hope this solves your problem :)

Daniel Smith
10,172 Pointsyour last call simply adds a single item to the list instead of appending whatever value is passed through so it effectively has completed the task after one item
Ben Aldrich
3,946 PointsBen Aldrich
3,946 PointsI will take a look at this for you now... Edit 1: First thing to notice is Line 35:
shopping_list.append(new_item)
You should be passing through item:
shopping_list.append(item)
This is because your function add_to_list has the parameter passed in called (item). Remember it doesn't matter what the name of the thing you pass in is, it will be treated based on the name you gave it as a parameter. So when you called the function you said add_to_list("NEW_ITEM)" but when it is in the function it is treated as ("ITEM") - ignore the caps.
EDIT2 2: The core problem you were having is indentation... On line 26
All of your code that is required to run has been indented so that it will only run as part of the Else statement. It should be following your Else statement (indented at the same level).
See final code before and read comments.