Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial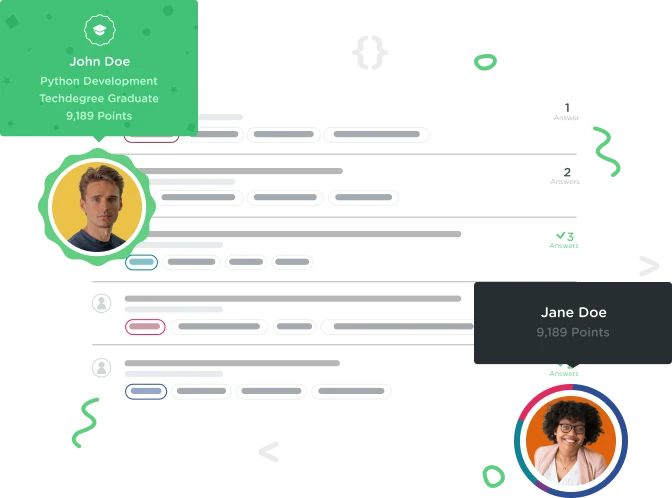
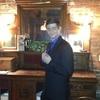
Carl Smith
8,185 PointsCan't adopt this protocol
I've asked this same question earlier but not a response yet, hopefully someone can help? I'm trying to adopt a new protocol to a class. I setup the protocol and the method that the class will have to conform to. However, I can't figure out how to implement the function into the class?
// Declare protocol here
protocol ColorSwitchable {
func switchColor(color: Color)
}
enum LightState {
case On, Off
}
enum Color {
case RGB(Double, Double, Double, Double)
case HSB(Double, Double, Double, Double)
}
class WifiLamp {
let state: LightState
var color: Color
init() {
self.state = .On
self.color = .RGB(0,0,0,0)
}
func switchColor(color: Color) {
let newColor = Color.HSB(255, 255, 255, 255)
}
}
1 Answer
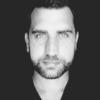
Jhoan Arango
14,575 PointsHello:
You are doing good, the only thing is that the challenge is not asking you to create an instance of "Color" as you are doing in the function.
What you want to do is change the "color" property within the class. Meaning that if you create an instance of wifiLamp, then you will be able to call on that function and change the property.
func switchColor(color: Color) {
self.color = .RGB(2.0,2.0,2.0,1.0)
}
Good luck
Carl Smith
8,185 PointsCarl Smith
8,185 PointsI was trying everything else except for the "self.", Thank you. I need to study the "self." more, i'm also revisiting functions.
Jhoan Arango
14,575 PointsJhoan Arango
14,575 Pointsself is simple.. when you see that there is a conflict of names, then make sure to use self. In this case self.color is being used because the function has a parameter named "color" the same as the property "color" from the class. So we want to specify self.color to let the system know that we are referring to the property and not the parameters name from the function.
Carl Smith
8,185 PointsCarl Smith
8,185 PointsIt's still no passing, however, the code does work in Xcode?
Carl Smith
8,185 PointsCarl Smith
8,185 PointsThis is what I have.
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsYou forgot to adopt the protocol
Carl Smith
8,185 PointsCarl Smith
8,185 PointsThat's what I'm not understanding how to do?
Carl Smith
8,185 PointsCarl Smith
8,185 PointsI figured it out! I was forgetting to add :ColorSwitchable to the class. I didn't understand how to do it until I revisited the video. Thanks!
Jhoan Arango
14,575 PointsJhoan Arango
14,575 Points