Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial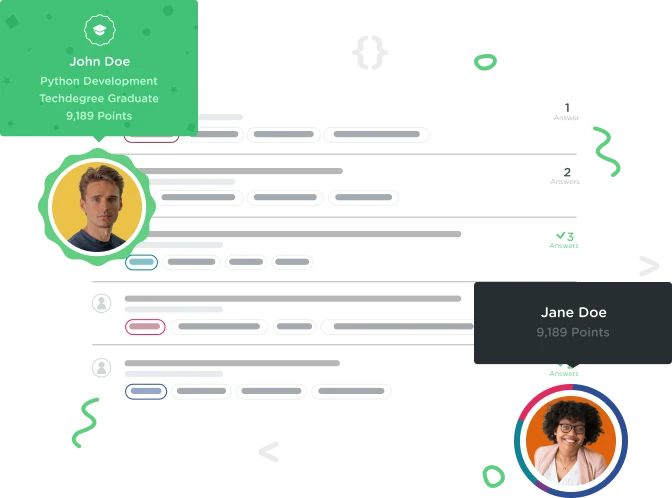

Kyle Rayner
1,629 PointsCan't assign value to an array in Visual Studio
For some reason I can't assign a value to an element in an array that I just initialized. I can initialize and assign to the elements in an array if I do it within the same line of code. However, in this application the elements are going to have a lot of text so I want them to be assigned afterwards. It works in Treehouse's workplaces environment but not on Visual Studio. Can anyone figure it out (Probably something simple) ? Thanks in advance.
Comes up with these error messages: CS0270 Array size cannot be specified in a variable declaration (try initializing with a 'new' expression) CS1519 Invalid token '=' in class, struct, or interface member declaration CS0103 The name 'option' does not exist in the current context.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace TouchTypingGame
{
class Passages
{
string[] option = new string[8];
option[0] = "Hello"; //this is the only line that seems to be the problem;
}
}
1 Answer
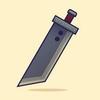
Allan Clark
10,810 PointsYour problem here is that you are building a class not running commands in REPL. anything thats not contained in a method is read as a member variable. option[0] = "Hello"; is not a valid member variable. It works on one line because you can initialize the variable in its declaration.
You could do it separately by adding this instruction to the constructor like this:
class Passages
{
string[] option;
public Passages()
{
option = new string[8];
option[0] = "Hello"; //this is the only line that seems to be the problem;
}
}
or you could do it all on one line as you suggested you have tried, this should work:
class Passages
{
string[] option = new string[8] {"Hello"};
}
the single line should work for what you need. The assignment of the value is only done when the object is constructed and can be changed later as long as the const or readonly keywords are not used. This is an example of 'syntactic sugar' the single line solution accomplishes the same thing but is much easier to read.
Kyle Rayner
1,629 PointsKyle Rayner
1,629 PointsThank you so much for the help. It was doing my head in. Works well now. I knew I was just not thinking about it the right way.