Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial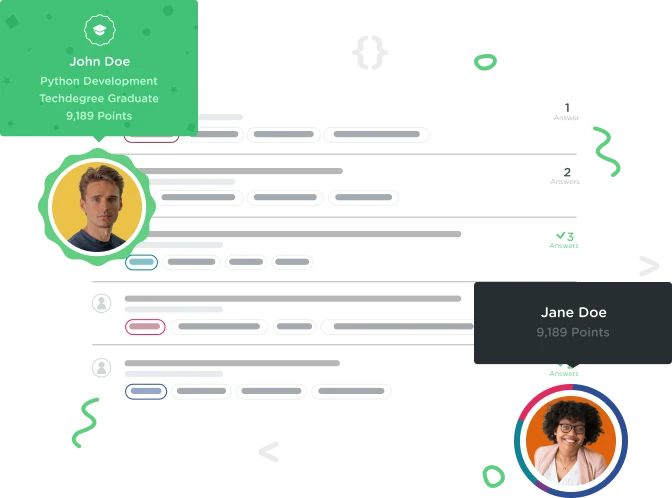

Alastair Herd
2,306 PointsCan't click on categories.
When I click on each of the categories (e.g. http://localhost:8080/category/1) I get a Whitelabel error.
I've put in the main body of Category.html below:
<div class="gifs container">
<!--
In the div element, replace the 'category name' text
with the value of the 'name' field of the 'category'
object from the model map
-->
<div class="chip" th:text="${category.name}">category name</div>
<div class="row">
<!--
Repeat the div element for each object in the 'gifs'
collection of the model map. Each object in the loop
should be named 'gif'
-->
<div class="col s12 l4">
<!--
In the a tag, make the href attribute point to
the GIF detail page using the 'name' field of
the 'gif' object from the loop above
-->
<a>
<!--
In the img tag, make the src attribute point
to the static GIF image, according to the 'name'
field of the 'gif' object from the loop above
-->
<img src="http://placehold.it/200x200" />
<!--
In the a tag, make the class attribute have the value
of 'mark favorite' or 'unmark favorite' according to
the 'favorite' field of the 'gif' object from the
loop above
-->
<a href="#" class="mark favorite"></a>
</a>
</div>
</div>
</div>
And this is my CategoryRepository.java
package com.teamtreehouse.giflib.data;
import com.teamtreehouse.giflib.model.Category;
import org.springframework.stereotype.Component;
import java.util.Arrays;
import java.util.List;
@Component
public class CategoryRepository {
private static final List<Category> ALL_CATEGORIES = Arrays.asList(
new Category(1, "Technology"),
new Category(2, "People"),
new Category (3, "Destruction"));
public List<Category> getAllCategories() {
return ALL_CATEGORIES;
}
public Category findById(int id) {
for (Category category : ALL_CATEGORIES) {
if (category.getId() == id) {
return category;
}
}
return null;
}
}
But I'm not sure where the problem is coming from. The main categories page works fine, it's just the actual categories themselves.
Thank you very much in advanced for any help.
3 Answers

Alastair Herd
2,306 Points'''HTML
<!DOCTYPE html> <html lang="en" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"/> <meta name="viewport" content="width=device-width, initial-scale=1"/>
<link rel="icon" th:href="@{/favicon.png}" />
<link rel="stylesheet" th:href="@{/vendor/materialize/css/materialize.css}" />
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons" />
<link rel="stylesheet" th:href="@{/app.css}" />
<title th:text="'giflib | ' + ${category.name}">giflib | Category</title>
</head> <body> <div class="navbar-fixed"> <nav> <div class="container"> <a th:href="@{/}" class="brand-logo">gif<span>.</span>lib</a> <a href="#" data-activates="mobile-nav" class="button-collapse right"><i class="material-icons">menu</i></a> <ul class="right hide-on-med-and-down"> <li><a th:href="@{/}">Explore</a></li> <li><a th:href="@{/categories}">Categories</a></li> <li><a th:href="@{/favorites}">Favorites</a></li> </ul> <ul id="mobile-nav" class="side-nav"> <li><a th:href="@{/}">Explore</a></li> <li><a th:href="@{/categories}">Categories</a></li> <li><a th:href="@{/favorites}">Favorites</a></li> </ul> </div> </nav> </div> <div class="search-bar container"> <div class="row"> <div class="col s12"> <form action="#" method="get"> <div class="input-field"> <input name="q" type="search" placeholder="Search all gifs..." required="required" autocomplete="off"/> <i class="material-icons">search</i> </div> </form> </div> </div> </div> <div class="gifs container"> <!-- In the div element, replace the 'category name' text with the value of the 'name' field of the 'category' object from the model map --> <div class="chip" th:text="${category.name}">category name</div> <div class="row"> <!-- Repeat the div element for each object in the 'gifs' collection of the model map. Each object in the loop should be named 'gif' --> <div class="col s12 l4"> <!-- In the a tag, make the href attribute point to the GIF detail page using the 'name' field of the 'gif' object from the loop above --> <a> <!-- In the img tag, make the src attribute point to the static GIF image, according to the 'name' field of the 'gif' object from the loop above --> <img src="http://placehold.it/200x200" /> <!-- In the a tag, make the class attribute have the value of 'mark favorite' or 'unmark favorite' according to the 'favorite' field of the 'gif' object from the loop above --> <a href="#" class="mark favorite"></a> </a> </div> </div> </div>
<script th:src="@{/vendor/jquery/jquery-1.11.3.js}"></script> <script th:src="@{/vendor/materialize/js/materialize.js}"></script> <script th:src="@{/app.js}"></script> </body> </html>
'''
I can't seem to post it without it malfunctioning and looking like a mess, but this is my best shot.

Isaiah Duncan
3,241 PointsThere doesn't appear to be anything wrong with this HTML file. Can I see your Category Controller class and your Category model?

Alastair Herd
2,306 PointsThis is my category controller class.
'''Java
package com.teamtreehouse.giflib.controller;
import com.teamtreehouse.giflib.data.CategoryRepository; import com.teamtreehouse.giflib.data.GifRepository; import com.teamtreehouse.giflib.model.Category; import com.teamtreehouse.giflib.model.Gif; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.ModelMap; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping;
import java.util.List;
@Controller public class CategoryController { @Autowired private CategoryRepository categoryRepository;
@Autowired
private GifRepository gifRepository;
@RequestMapping("/categories")
public String listCategories(ModelMap modelMap) {
List<Category> categories = categoryRepository.getAllCategories();
modelMap.put("categories",categories);
return "categories";
}
@RequestMapping ("/categories/{id}")
public String category(@PathVariable int id, ModelMap modelMap) {
Category category = categoryRepository.findById(id);
modelMap.put("category", category);
List<Gif> gifs = gifRepository.findByCategoryId(id);
modelMap.put("gifs", gifs);
return "category";
}
}
'''
And this is my category model
'''Java
package com.teamtreehouse.giflib.model;
public class Category {
private int id;
private String name;
public Category(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
'''

Isaiah Duncan
3,241 PointsIt's a bit hard to read your code properly due to the formatting issues.
Ensure that you are using this character: ` (this is next the the 1 on a standard keyboard) Ensure that you are NOT using this character: ' (this is next to the enter/return on a standard keyboard)
Also, when specifying which language it is, keep it all lowercase like: java And NOT like: JAVA
So the beginning of your code block would look like: [```java ] And NOT like: ['''JAVA]
Remove brackets.

Isaiah Duncan
3,241 PointsSo I believe I see the issue now.
This is the URI you gave initially, saying you couldn't hit the page:
http://localhost:8080/category/1
And this is the URI you are trying to hit in your CategoryController:
@RequestMapping ("/categories/{id}")
There is inconsistency between the two URIs and I believe this is where you are going wrong.
Isaiah Duncan
3,241 PointsIsaiah Duncan
3,241 PointsCan I see your full HTML file for Category?