Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial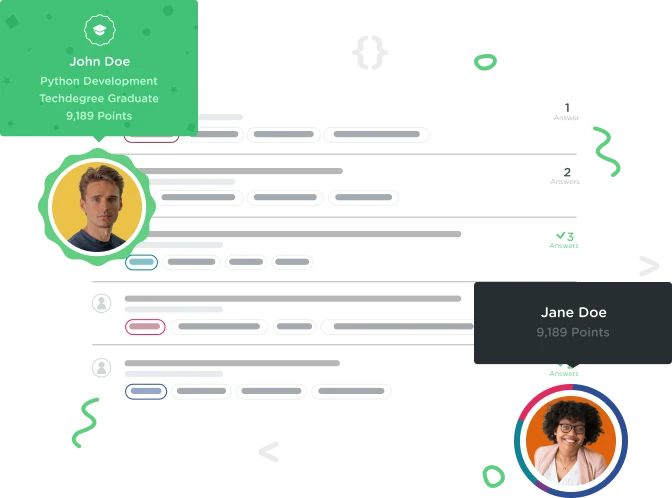
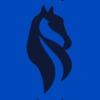
Ben Masel
2,004 PointsCan't complete these tasks!
Task 1 i can't complete, I have tried many things. If you want me to try again i will but please tell me if it uses Unary Operators, Binary Operators or both. It also says on swift 3 "++" and "--" will be removed. What operator will replace them?
// Enter your code below
var initialScore = 8
initialScore = initialScore + 1
let totalScore = initialScore
4 Answers
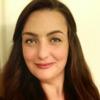
Jennifer Nordell
Treehouse TeacherBenjamin Masel Actually, I just had a thought. Let me show you the equivalent code without using an increment operator. Maybe it will clarify things.
This:
var initialScore = 8
let totalScore = initialScore++
is equivalent to:
var initialScore = 8
let totalScore = initialScore // totalScore is now 8
initialScore = initialScore + 1 // initialScore is now 9
And this:
var initialScore = 8
let totalScore = ++initialScore
is equivalent to:
var initialScore = 8
initialScore = initialScore + 1 //initialScore is now equal to 9
let totalScore = initialScore //totalScore is now equal to 9
Again, the main idea here is the order in which the assignment and the increments happen. Hope this helps
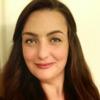
Jennifer Nordell
Treehouse TeacherAs I understand it, in Swift 3 you will be using code like x += 1
instead of x++
. This challenge requires the use of a unary operator.
Now, let's look at step one:
var initialScore = 8
let totalScore = ++initialScore
This sets initalScore
to 8. Then it increments initialScore
to 9 and assigns 9 back into totalScore
. At this point totalScore
and initialScore
both hold the value of 9.
But if we did it this way:
var initialScore = 8
let totalScore = initialScore++
This would first assign initalScore
(which has a value of 8) into totalScore
and then it would increment initialScore
to 9. The result would then be that we would have 8 in totalScore
and 9 in initialScore
. Hope this helps!
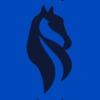
Ben Masel
2,004 PointsThank You!
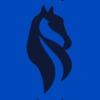
Ben Masel
2,004 PointsIt helped so MUCH!
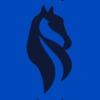
Ben Masel
2,004 PointsSo the "++" makes the constant in this case "totalScore = totalScore + 1" which is "totalScore = ++totalScore"? and if it was "totalScore = totalScore++" it would make totalScore = initialScore but initialScore won't equal totalScore?
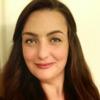
Jennifer Nordell
Treehouse TeacherBenjamin Masel there is no code that increments totalScore
. What they are trying to illustrate to you here is the difference between putting the ++
before the initalScore
and putting it after. Let's look at it one more time.
If we have this:
var initialScore = 8
let totalScore = initialScore++
In this case, initialScore
starts at 8. Now we have an empty constant named totalScore
. It has no value. We assign initalScore
back into totalScore
. At this point, totalScore
is is now equal to 8. But after we do the assignment we're going to increment initialScore
. This will mean that initialScore
now has a value of 9, but totalScore
still only has a value of 8.
If we do this:
var initialScore = 8
let totalScore = ++initialScore
Now this starts off the same. initialScore
has a value of 8 and totalScore has no value. But here's the big thing. We do the increment before we do the assignment. So now we increment initialScore
to 9 and then we assign it back into totalScore
. Now initalScore
and totalScore
both have a value of 9.
Keep in mind though, that this is being deprecated. Also, there are rather few scenarios where this matters that much. But they clearly want you to be familiar with it.
Hope this helps!