Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial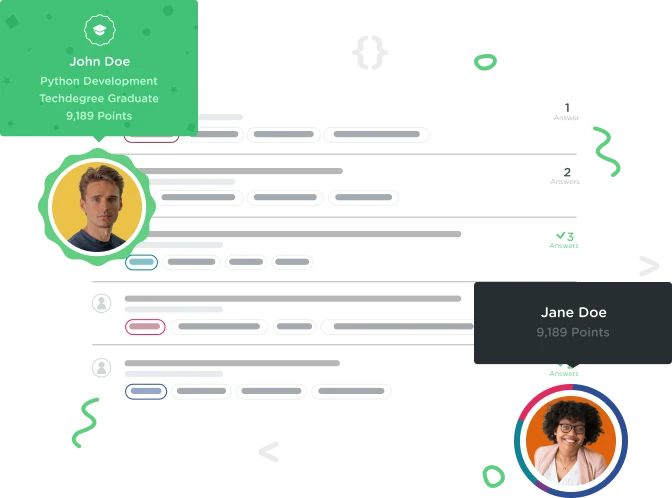

Richard Hummel
Courses Plus Student 5,677 PointsCan't convert 'int' object to str implicitly...not sure what I'm doing wrong
I'm not sure what I'm doing wrong...I know I'm not converting the int properly to strings.
# add_list([1, 2, 3]) should return 6
def add_list(aList):
for each in aList:
total = sum(aList)
return total
# summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
def summarize(aList):
X = " ".join(str(e) for e in aList)
Y = add_list(aList)
return "The sum of " + X + "is " + Y + "."
# Note: both functions will only take *one* argument each.
1 Answer
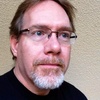
Chris Freeman
Treehouse Moderator 68,441 PointsThe error occurs in your return
statement where it is trying to form a string from concatenation. This only works with items that are strings.
Since X is an int
it needs to be converted directly using str()
or through a format statement:
# using str()
return "The sum of " + str(X) + "is " + Y + "."
# using format()
return "The sum of {} is ".format(X) + Y + "."
Other issues with your code::
- The Y value for a list
[1, 2, 3]
is "1 2 3". The challenge is looking for the string representation of the list "[1, 2, 3]". A list can be converted to a string directly also usingstr()
orformat()
- missing space before "is "
# using str()
def summarize(aList):
X = str(aList)
Y = str(add_list(aList))
return "The sum of " + X + " is " + Y + "." #<-- added missing space
# using format()
def summarize(aList):
return "The sum of {X} is {Y}.".format(X=aList, Y=add_list(aList))
# which can be shortened to
def summarize(aList):
return "The sum of {} is {}.".format(aList, add_list(aList))