Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial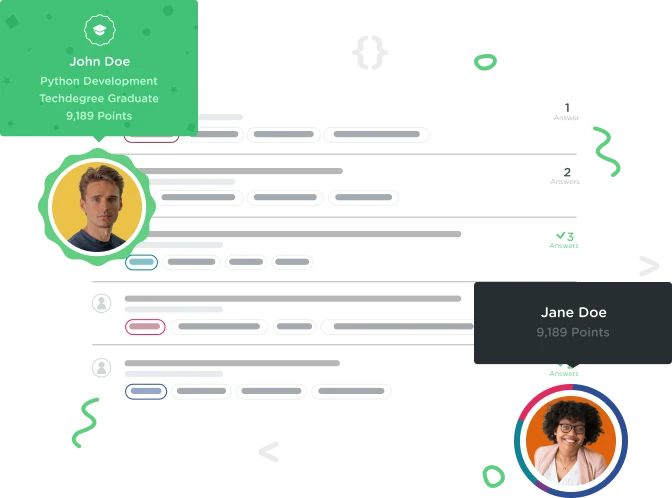
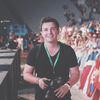
Jamie Halvorson
12,590 PointsCan't display username, receiving error
Hi,
I'm struggling to get the username and badge count to display. I've tried for almost an hour now, changing the HTTP to HTTPS, different ports and so on. Any help would be greatly appreciated.
Code:
//App.js file
var router = require("./router.js");
//Problem:We need a simple way to look at a user's badge count and JavaScript points from the web browser
//Solution:Use Node.js to perform the profile look ups and serve our templates via HTTPS
// Create a web server
var http = require('http');
http.createServer(function (request, response) {
router.home(request, response);
router.user(request, response);
//response.end('Hello World\n');
}).listen(3000);
console.log('Server running at http://<workspace-url>');
// Function that handles the reading of files and merge in values
//read from file and get a string
// merge values into string
//Router.js File
var Profile = require("./profile.js");
// Handle the HTTPS route GET / and POST / i.e Home
function home(request, response){
//if url == "/" && GET
if(request.url === "/"){
// show search
response.writeHead(200, {'Content-Type': 'text/plain'});
response.write("Header \n");
response.write("Search \n");
response.end('Footer \n');
}
//if url == "/" && POST
// redirect to /:username
}
// Handle the HTTPS route for GET/:username i.e /jamiehalvorson
function user(request, response){
//if the url == "/...|
var username = request.url.replace("/", "");
if(username.length > 0){
response.writeHead(200, {'Content-Type': 'text/plain'});
response.write("Header \n");
//get the json from Treehouse
var studentProfile = new Profile(username);
//on "end"
studentProfile.on("end", function(profileJSON){
//show profile
//Store the values which we need
var values = {
avatarUrl: profileJSON.gravatar_url,
username: profileJSON.profile_name,
badges: profileJSON.bages.length,
javascriptPoints: profileJSON.points.JavaScript
}
//simple response
response.write(values.username + " has " + values.badges + " badges\n");
response.end('Footer \n');
});
//on "error"
studentProfile.on("error", function(error){
//show error
response.end('Error \n');
});
}
}
module.exports.home = home;
module.exports.user = user;
//Profile.js File
var EventEmitter = require("events").EventEmitter;
var http = require("http");
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"));
}
//Read the data
response.on('data', function (chunk) {
body += chunk;
profileEmitter.emit("data", chunk);
});
response.on('end', function () {
if(response.statusCode === 200) {
try {
//Parse the data
var profile = JSON.parse(body);
profileEmitter.emit("end", profile);
} catch (error) {
profileEmitter.emit("error", error);
}
}
}).on("error", function(error){
profileEmitter.emit("error", error);
});
});
}
util.inherits( Profile, EventEmitter );
module.exports = Profile;
The current 404 error that I am getting is:
var EventEmitter = require("events").EventEmitter;
var http = require("http");
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"));
}
//Read the data
response.on('data', function (chunk) {
body += chunk;
profileEmitter.emit("data", chunk);
});
response.on('end', function () {
if(response.statusCode === 200) {
try {
//Parse the data
var profile = JSON.parse(body);
profileEmitter.emit("end", profile);
} catch (error) {
profileEmitter.emit("error", error);
}
}
}).on("error", function(error){
profileEmitter.emit("error", error);
});
});
}
util.inherits( Profile, EventEmitter );
module.exports = Profile;
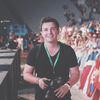
Jamie Halvorson
12,590 PointsThanks, Andrew VanVlack, I've changed the link.
2 Answers

Andrew VanVlack
20,155 PointsTreehouse seems to have changed/broken there api for the profiles and only responding to https requests. If you want to continue untill they fix this I have updated the profile.js to make https request:
var EventEmitter = require("events").EventEmitter;
var http = require("http");
var https = require("https");
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"));
}
//Read the data
response.on('data', function (chunk) {
body += chunk;
profileEmitter.emit("data", chunk);
});
response.on('end', function () {
if(response.statusCode === 200) {
try {
//Parse the data
var profile = JSON.parse(body);
profileEmitter.emit("end", profile);
} catch (error) {
profileEmitter.emit("error", error);
}
}
}).on("error", function(error){
profileEmitter.emit("error", error);
});
});
}
util.inherits( Profile, EventEmitter );
module.exports = Profile;
All I did was added node's https module and changed the get request to https. I left the http as the https dose not have a list of status codes for error handling.
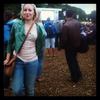
Rachel Black
3,943 PointsI really wish treehouse would update the tutorial for this. I just wasted an hour trying to figure it out.
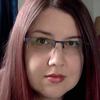
Tree Casiano
16,436 PointsThank you! This did the trick.
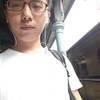
PeiYao Chung
7,386 PointsThank you!!
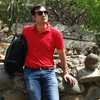
Nikhil Khandelwal
9,371 PointsHey @Jamie Halvorson, please mark Andrew VanVlack answer as accepted answer, as I too wasted hours finding the solution for https issue. This will make this solution stand out and save many more hours of many.
Thank in advance
Andrew VanVlack
20,155 PointsAndrew VanVlack
20,155 PointsIt looks like you pasted the wrong text for your error. Also your link for your workspace is only viewable by you. To share a workspace, click on the camera in corner and press 'Take Snapshot' and share that link.